What is AllowFontScaling Prop in React Native?
Published On: 2024-02-14
Posted By: Harish
AllowFontScaling is a React Native's TextInput prop which manages the scaling of the TextInput's font size when system wide font size is changed.
Applied text size will be shown when AllowFontScaling
prop isfalse
even when the OS system’s font size is changed in either android or iOS. Lets see that in action.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Add a TextInput Field
Now let's add a <TextInput>
and <Text>
field inside <View>
. This text field's text is to see the difference of font change when system wide text size is changed.
Add some text in <Text>
and <TextInput>
fields and assign font sizes to both the fields. Now, if you run the project (in iOS or Android), applied font sizes will be displayed.
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
Let's check what will happen when the system wide font size setting is changed.
Add AllowFontScaling
prop to <TextInputField>
with false
as the value.
On Android
In android, change OS wide font size by visiting Settings > Display > Font Size. If you go back to the app, you will notice that the font of the Text
field is changed but the font of TextInput
field is unaffected.
Initial:
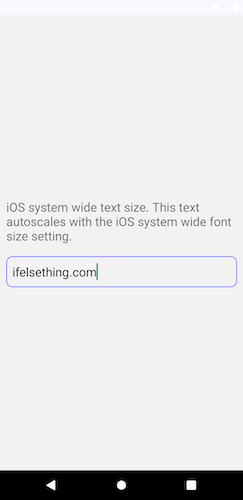
System wide font size is changed:
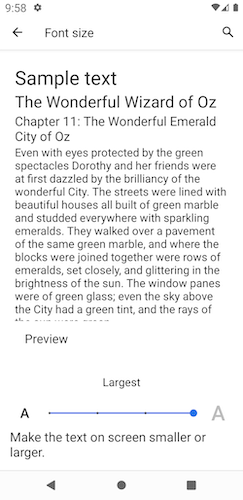
Final result:
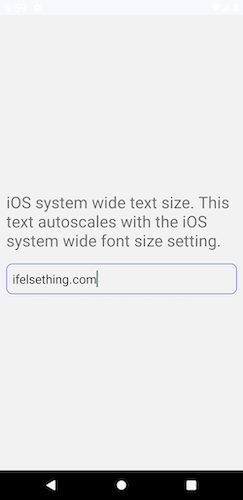
On iOS
You can change the font size on iOS by visiting Settings > Display & Brightness > Text Size. You will see the same behavior as in android.
Initial:
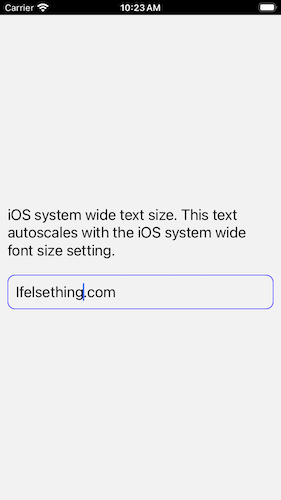
System wide font size is changed:
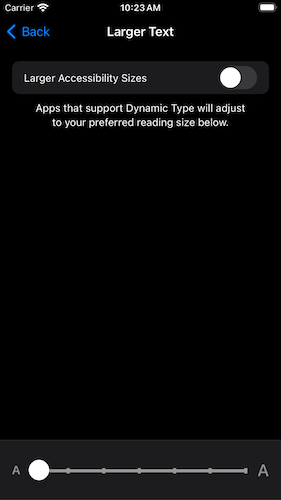
Final result:
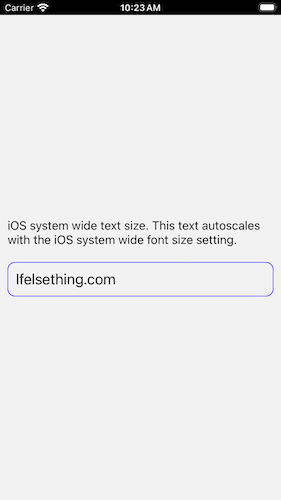
Complete code file,
//app.tsx
import {
View,
Text,
StyleSheet,
TextInput
} from "react-native";
export const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>
iOS system wide text size. This text autoscales with the iOS system wide font size setting.
</Text>
<TextInput
style={styles.input}
keyboardType="default"
allowFontScaling={false} //default is true when not mentioned.
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 20
},
text: {
fontSize: 20
}
});