Set Background Image in React Native
Published On: 2024-05-23
Posted By: Harish
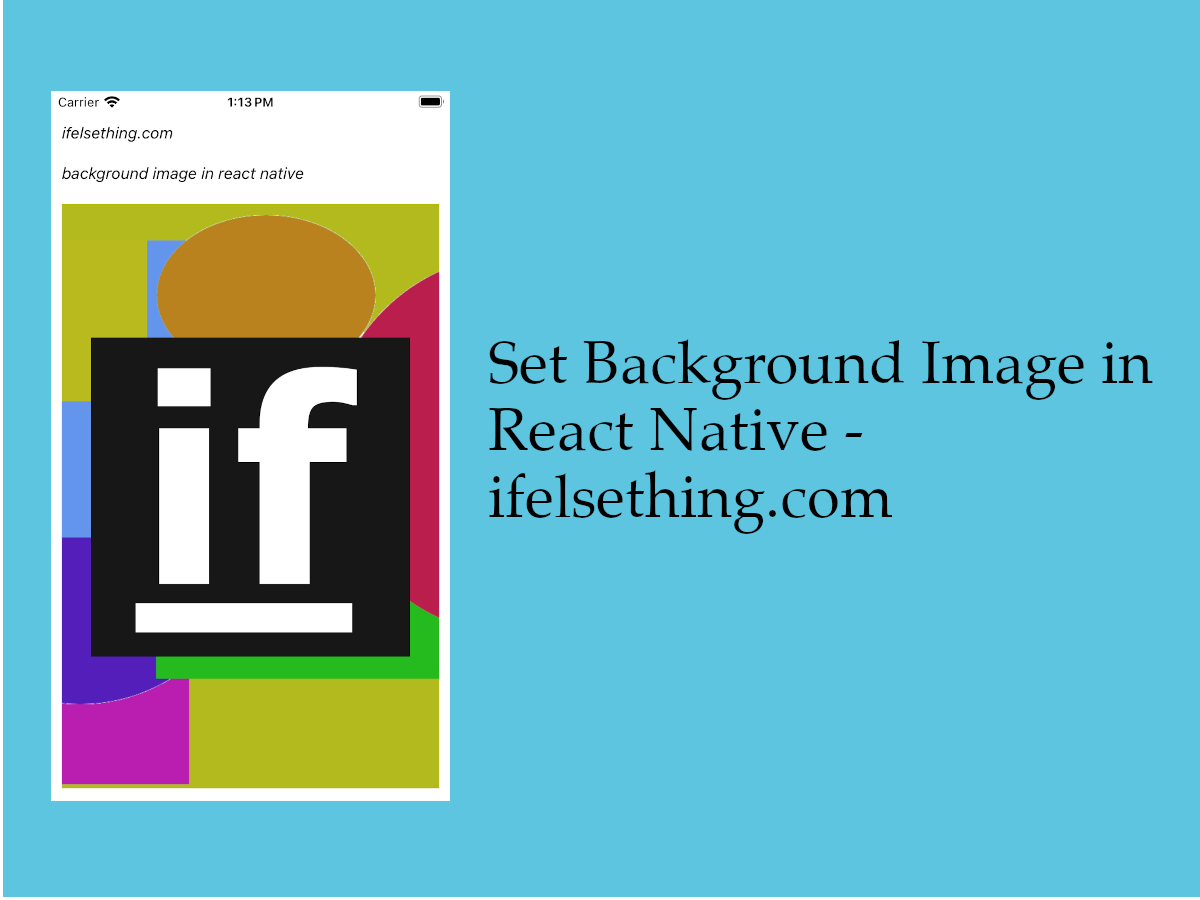
We can show an image using the Image component, but to show as a background image, we have to add extra views and an Image component and stack them to use as a background image.
But with the ImageBackground component, we can simply add a background image.
Lets see its usage with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ImageRN
Example Implementation
We will create a simple screen with a background image and a normal image at the center.
First import ImageBackground
component from react-native.
ImageBackground component has Image component’s props. So, we will add a local asset image as a source. Remote image can also be used.
//App.tsx
...
import { ImageBackground } from 'react-native';
...
<ImageBackground
style={styles.image_background}
resizeMode="cover"
source={require('./assets/background.png')}
>
</ImageBackground>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see the linked image. To have it as a background image, we have to add components inside the ImageBackground component.
Now, import and add the Image
component with an image. Here, we will use a remote image.
...
<ImageBackground ...>
<Image
style={styles.image}
resizeMode="cover"
source={{
uri: 'https://iet-images.s3.ap-south-1.amazonaws.com/fav.png'
}}
/>
</ImageBackground>
...
If we reload the screen, we can see an image over the background image. In this way we can set a background image easily.
We can say that this ImageBackground
component is a view with an image.
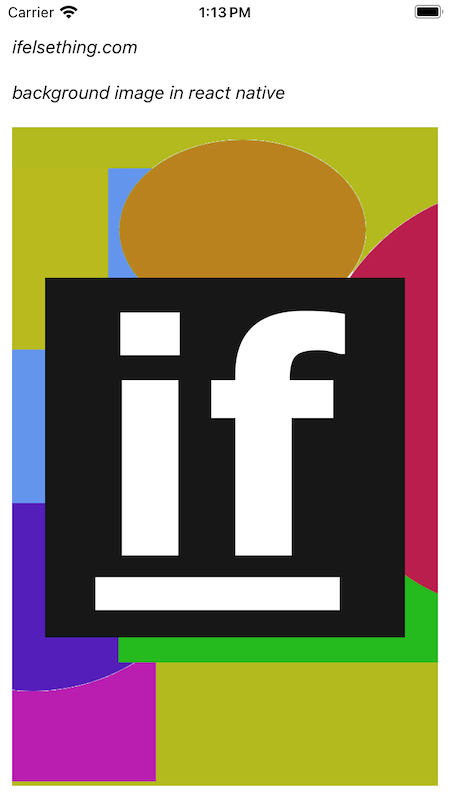
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
Image,
ImageBackground,
} from "react-native";
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
background image in react native
</Text>
<ImageBackground
style={styles.image_background}
resizeMode="cover"
source={require('./assets/background.png')}
>
<Image
style={styles.image}
resizeMode="cover"
source={{
uri: 'https://iet-images.s3.ap-south-1.amazonaws.com/fav.png'
}}
/>
</ImageBackground>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
image_background: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
image: {
flexShrink: 1,
width: 300,
height: 300
},
});