Change Cursor Color in React Native TextInput
Published On: 2024-03-15
Posted By: Harish
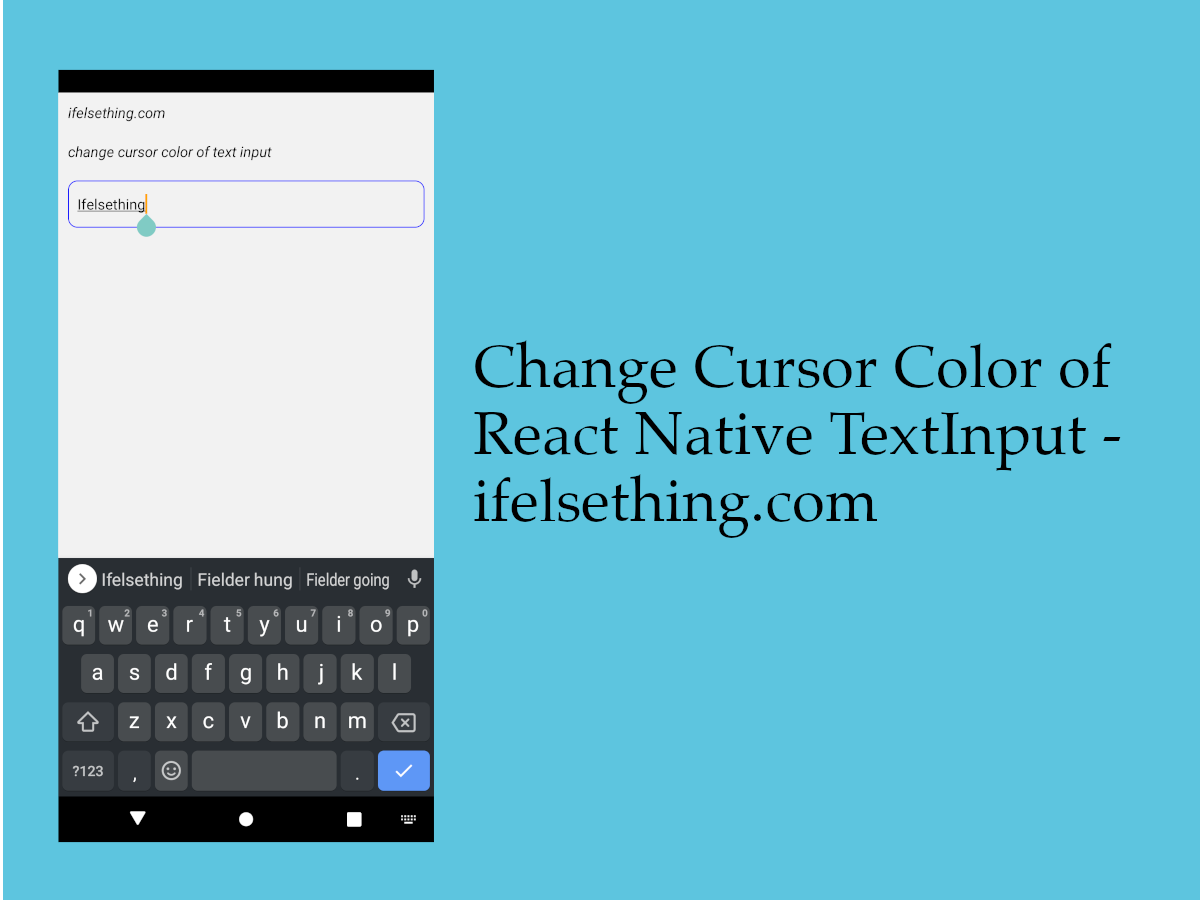
We have seen a way to change the cursor position of text input or to hide the cursor of react native text input. In this post, we will see a way to change the cursor color of text input.
We can change cursor color by using selectionColor prop. Pass the color value to the prop to change cursor color as well as background color when selected or highlighted.
With selectionColor
prop, we have another prop cursorColor which is only available for android devices.
Lets check their working with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will implement a simple screen with a text input and we will see the props in action for changing cursor color.
Import TextInput
and add needed default props.
//App.tsx
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Search"
placeholderTextColor='gray'
showSoftInputOnFocus={true}
autoCorrect={false}
autoFocus={true}
selectionColor="orange"
cursorColor="green"
/>
...
I'm using showSoftInputOnFocus, autoCorrect and autoFocus props to the text input as default props.
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
On screen render, we will see a blinking blue
cursor. This cursor color of the input is the default one.
If we add selectionColor
prop with a color, in our example its orange
, we can see that the cursor color will be changed to orange.
...
<TextInput
...
selectionColor="orange"
/>
...
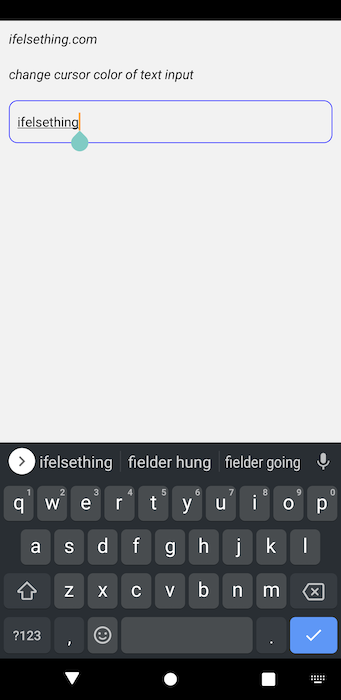
Also text highlight color will also be changed to the same as cursor color, i.e. orange.
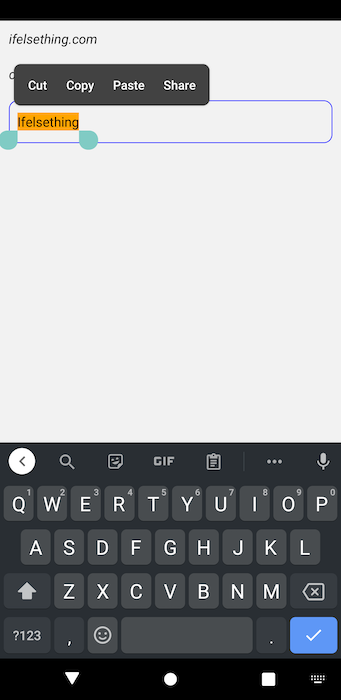
For android, there is an extra prop to change only cursor color irrespective of text highlight color. Add cursorColor
prop with a different color, in our example I passed lime
color. With this you will see that the blinking cursor color will be in lime color and selection color will be in orange, only in android.
...
<TextInput
...
selectionColor="orange"
cursorColor="lime"
/>
...
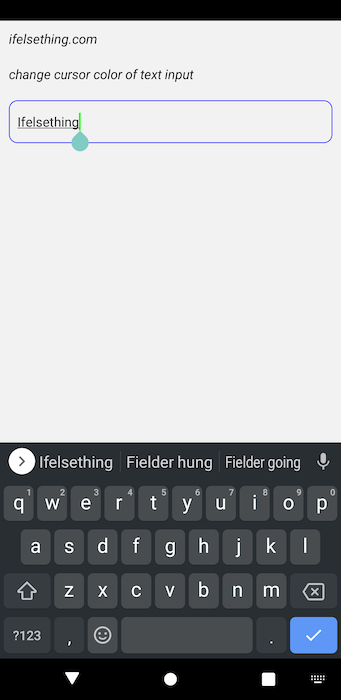
Complete code of our example,
//App.tsx
import {
View,
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
} from "react-native";
export default function App() {
return (
<SafeAreaView style={{ flex: 1 }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
change cursor color of text input
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Search"
placeholderTextColor='gray'
showSoftInputOnFocus={true}
autoCorrect={false}
autoFocus={true}
selectionColor="orange"
cursorColor="lime"
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
}
});