Clear Text on Focus in React Native TextInput
Published On: 2024-03-11
Posted By: Harish
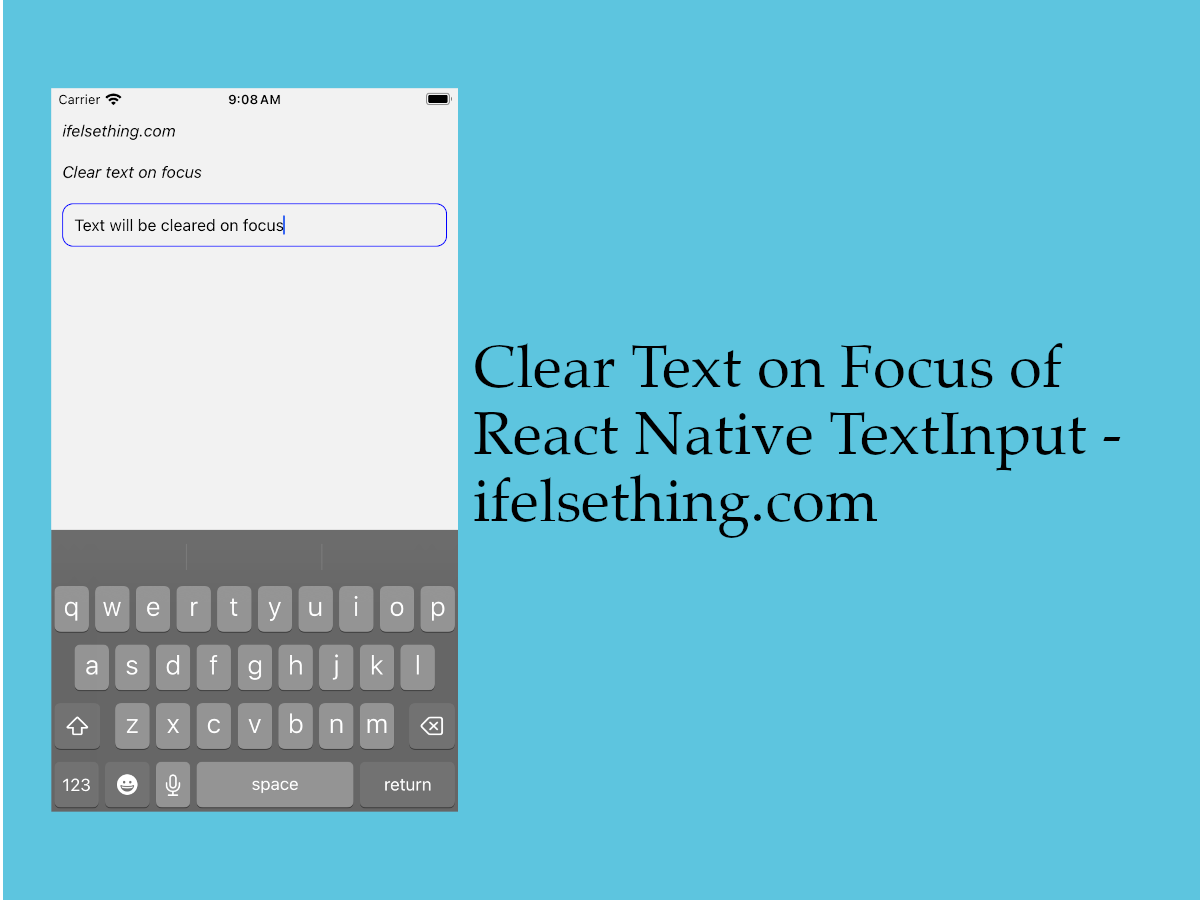
If a text input has a default value on render, we can clear the value when text input is focused by using clearTextOnFocus prop. So, when the user taps on the input to enter text, the value which is already present, will get cleared.
But there is a downside for this prop. clearTextOnFocus
prop is only applicable for iOS devices and not for android devices.
So in this post, we will check the functionality of clearTextOnFocus prop and look into a process to clear text on focus in android devices too.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will implement a simple screen with a text input with a default value.
I changed the app.tsx
file to an arrow function for ease. Import text input and add props which are needed.
//app.tsx
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
defaultValue="ifelsething"
autoCorrect={false}
/>
...
I hardcoded the default value for demonstration purposes. Here, defaultValue
stays till we enter a text in input.
So, to clear text on focus, use clearTextOnFoucs
prop with true
as value. Default value is false
.
...
<TextInput
...
clearTextOnFocus={true}
/>
...
If we re-run the metro builder, we will observe that the default value will be cleared when tapped on text input.
Value prop of text input shows the same behavior with clearTextOnFocus prop.
So, we have seen the functionality of the clearTextOnFocus prop. But as this is only available for iOS devices, we will implement our own clearTextOnFocus functionality for android devices.
Clear Text on Focus Functionality for Android
Without clearTextOnFocus prop, we can directly use this one functionality for both iOS and android.
Now, remove defaultValue prop
and clearTextOnFocus prop
from the text input. And we will import and use useState
to store the text input value, assign this value to input and to clear this value on focus.
import { useState } from 'react';
...
export const App = () => {
const [value, setValue] = useState<string | undefined>("ifelsething");
...
<TextInput
...
value={value}
onChangeText={setValue}
onFocus={() => setValue(undefined)}
/>
...
In the above code, we can see that the value state has a default value and this value changes when the user enters text in input. We are getting the text from the onChangeText
callback.
Now the main part, we have to clear the text on focus. For that, we have a callback called onFocus
for the TextInput component,, which callbacks when a text input is focused.
We are using onFocus to clear the text by updating the value to undefined. Complete behavior can be seen from gif below. Both clearTextOnFocus and this process show the same behavior.
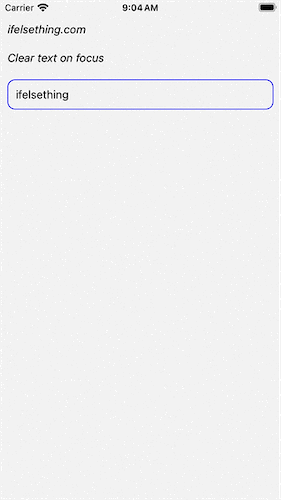
Complete code of our example,
//App.tsx
import { useState } from 'react';
import {
View,
Text,
StyleSheet,
SafeAreaView,
StatusBar,
TextInput
} from "react-native";
export const App = () => {
const [value, setValue] = useState<string | undefined>("ifelsething");
return (
<SafeAreaView style={{ flex: 1 }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Clear text on focus
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
value={value}
onChangeText={setValue}
onFocus={() => setValue(undefined)}
autoCorrect={false}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
}
});