Disable Copy/Paste in React Native Text Input
Published On: 2024-03-05
Posted By: Harish
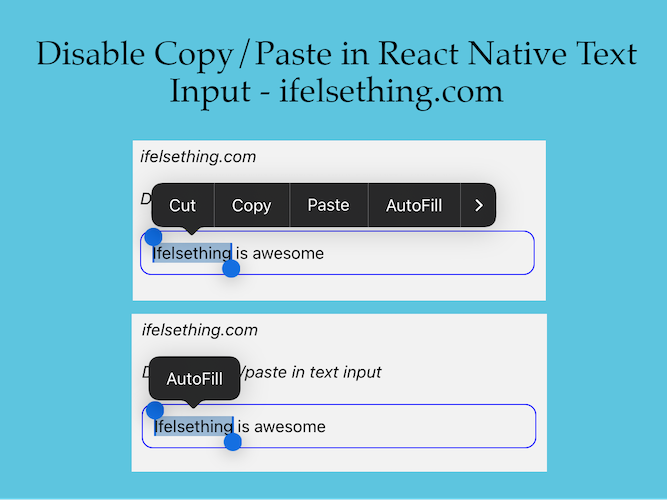
If your app screen has a process of adding a bank account number or anything important like changing password, we may add another confirmation input like confirm account number to match the value of the main input's value.
For this behavior, we need to disable copy and paste functionality of the text input. For this purpose, we can use contextMenuHidden prop which takes boolean values. If false
, the menu is shown and if true
, the menu is hidden. This is the best way to stop copying or pasting content in text input. Default value is false
.
Lets see the working in below example,
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
For this example, we will just take a text input component to check the behavior.
Import TextInput
component and add needed default props.
//app.tsx
import {
StyleSheet,
TextInput,
} from "react-native";
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
showSoftInputOnFocus={true}
autoCorrect={false}
autoFocus={true}
/>
...
Run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
Add some text to the text input, select it and try to copy the selected text by long pressing. You will see a context pop over the selected text and will find different options with copy and paste options.
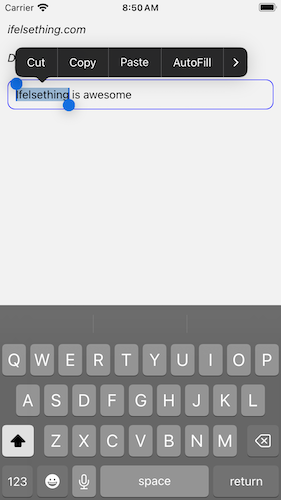
So to disable copy and paste, one of the best options is to not show these options. And contextMenuHidden
prop does the same. It just hides the menu, which will make the user to type the input.
Add contextMenu prop and pass true
value.
...
<TextInput
autoFocus={true}
contextMenuHidden={true}
/>
...
If we again try to open the context menu after refresh, the menu will not open or open with other options other than copy/paste options.
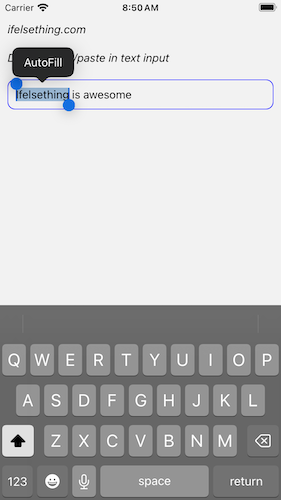
Complete code of our example,
//App.tsx
import {
View,
Text,
StyleSheet,
TextInput
} from "react-native";
export const App = () => {
return (
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Disable copy/paste in text input
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
showSoftInputOnFocus={true}
autoCorrect={false}
autoFocus={true}
contextMenuHidden={true}
/>
<Text>
</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
button_block: {
flexShrink: 1,
flexDirection: 'row',
gap: 20,
justifyContent: 'center'
}
});