Disable Full Screen Text Input Mode in React Native
Published On: 2024-03-15
Posted By: Harish
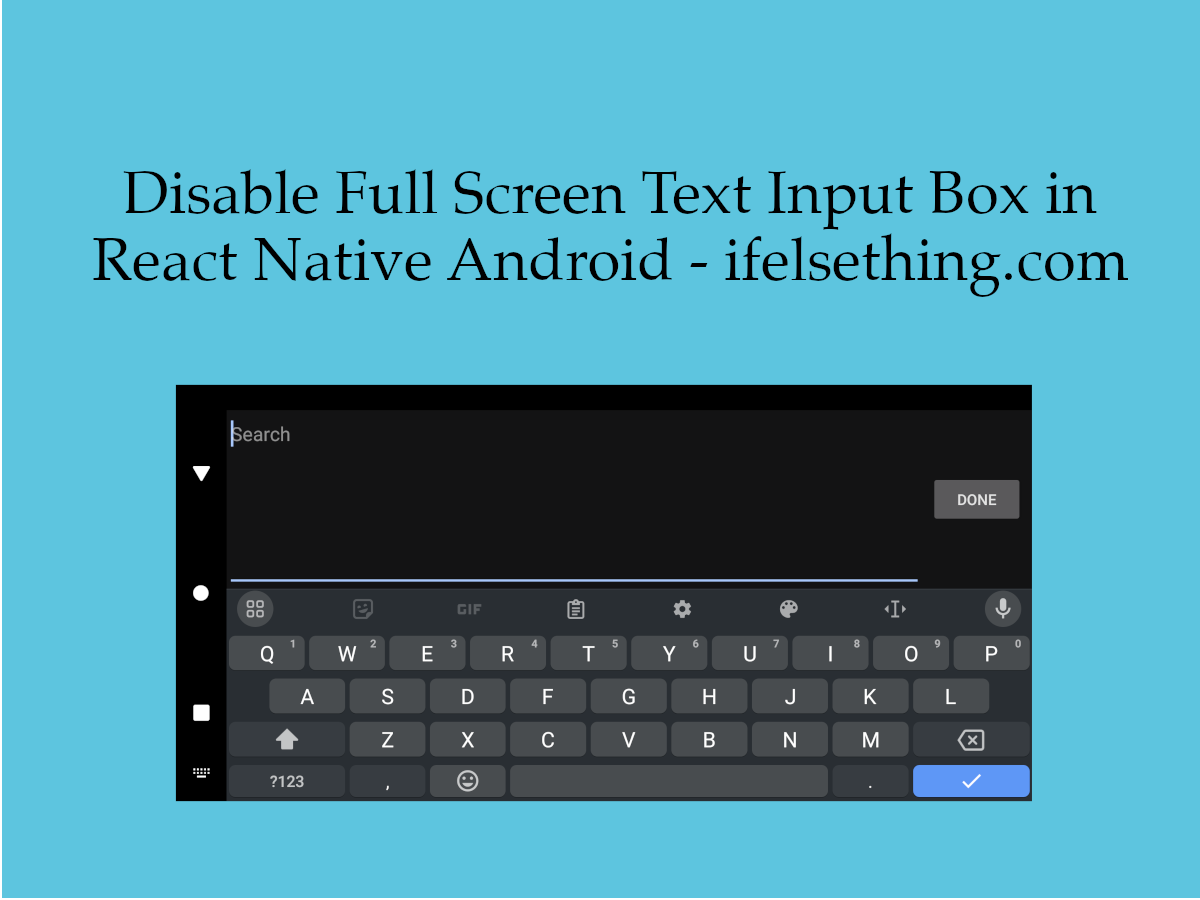
Whenever there is no extra space on screen after opening the keyboard, android will automatically fill the small space available to full text input irrespective of the text input styled size. This is like having a pop-over of a text box on the screen.
The best feature for small screen android devices or for screens when not much space is available to see and type. Best example is to enter text in landscape mode.
So, to disable this behavior, we can use disableFullscreenUI prop. This prop takes boolean values. If passed true
value, above behavior is disabled and vice versa. Default is false
. You can understand better by the below example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will implement a simple screen with a text input. We will run on an android emulator and rotate the screen to landscape mode.
Import TextInput
and add default props.
//App.tsx
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Search"
placeholderTextColor='gray'
autoCorrect={false}
/>
...
If we run the app and rotate the screen to landscape mode, we can see that the screen space is less for the keyboard and it may be difficult to type in the text input.
npx react-native run-android
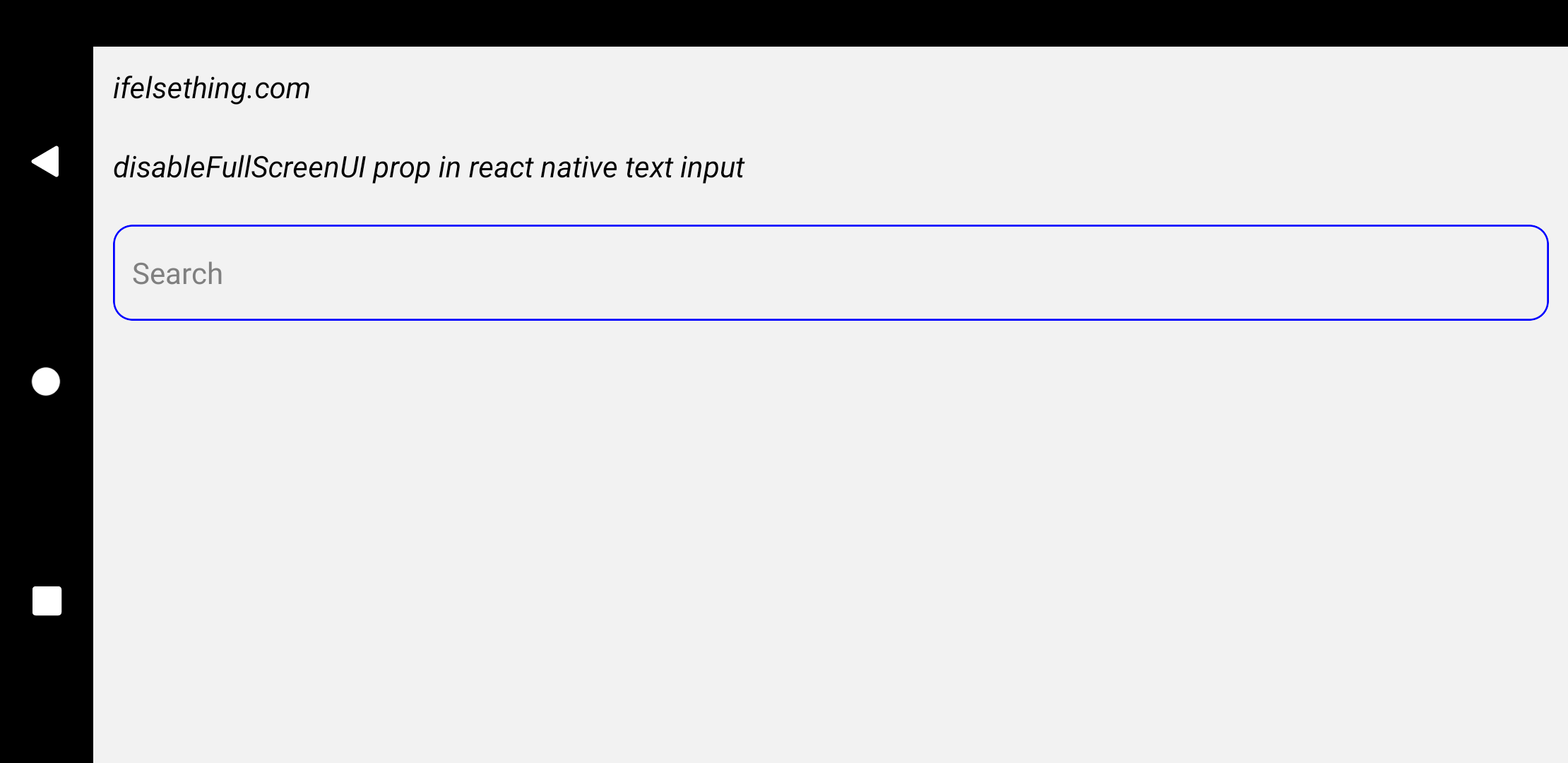
Now try to enter the text in text input. You will see that the text input becomes a text box and eases entering text into the text box. This is like a pop-over of the text input element but taking all keyboard-left screen space.
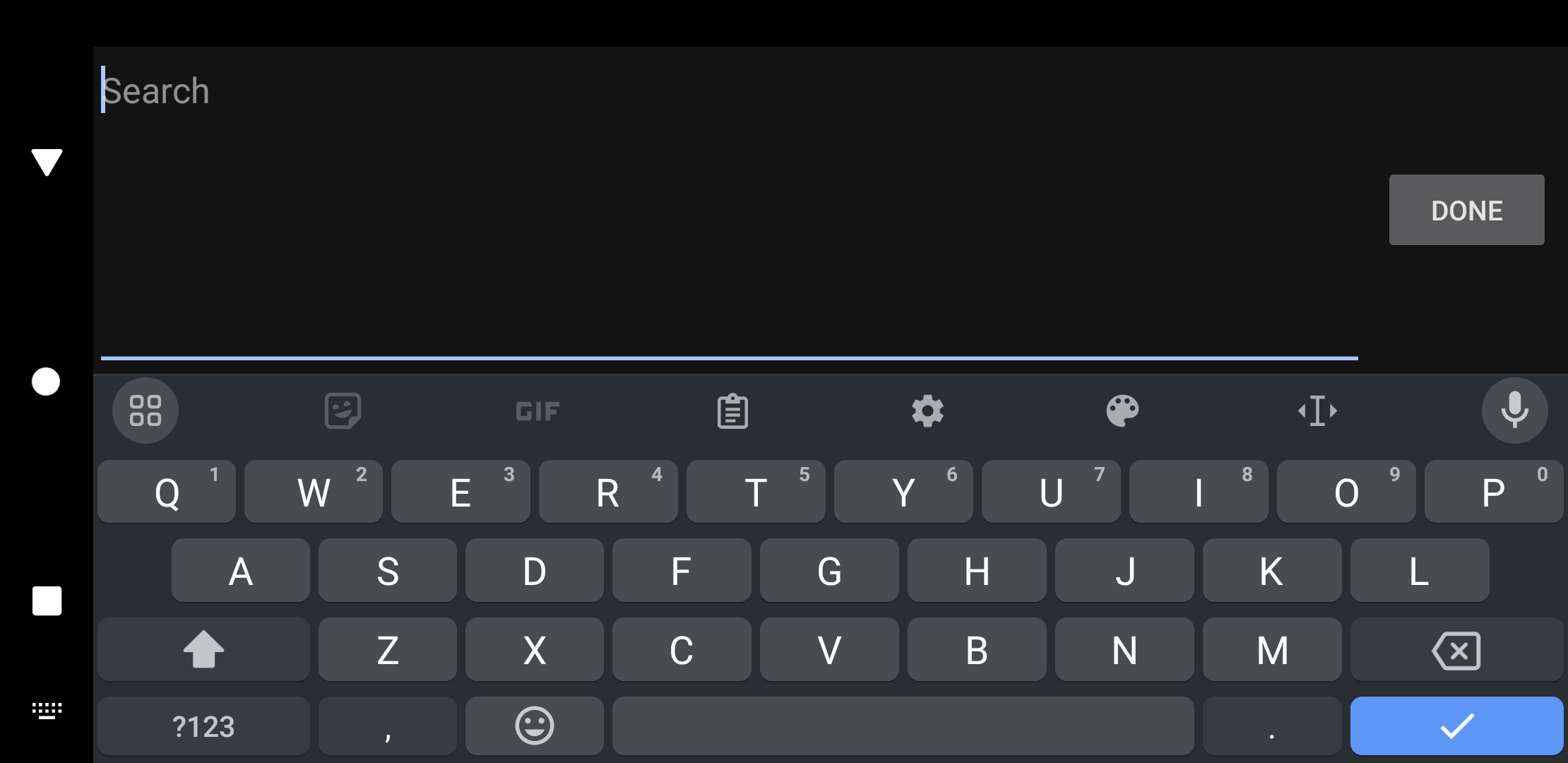
And to disable that functionality, we can use disableFullscreenUI
prop with true
value. If we add the prop and re-run the app, we will see the normal behavior of showing original text input.
...
<TextInput
...
disableFullscreenUI={true}
/>
...
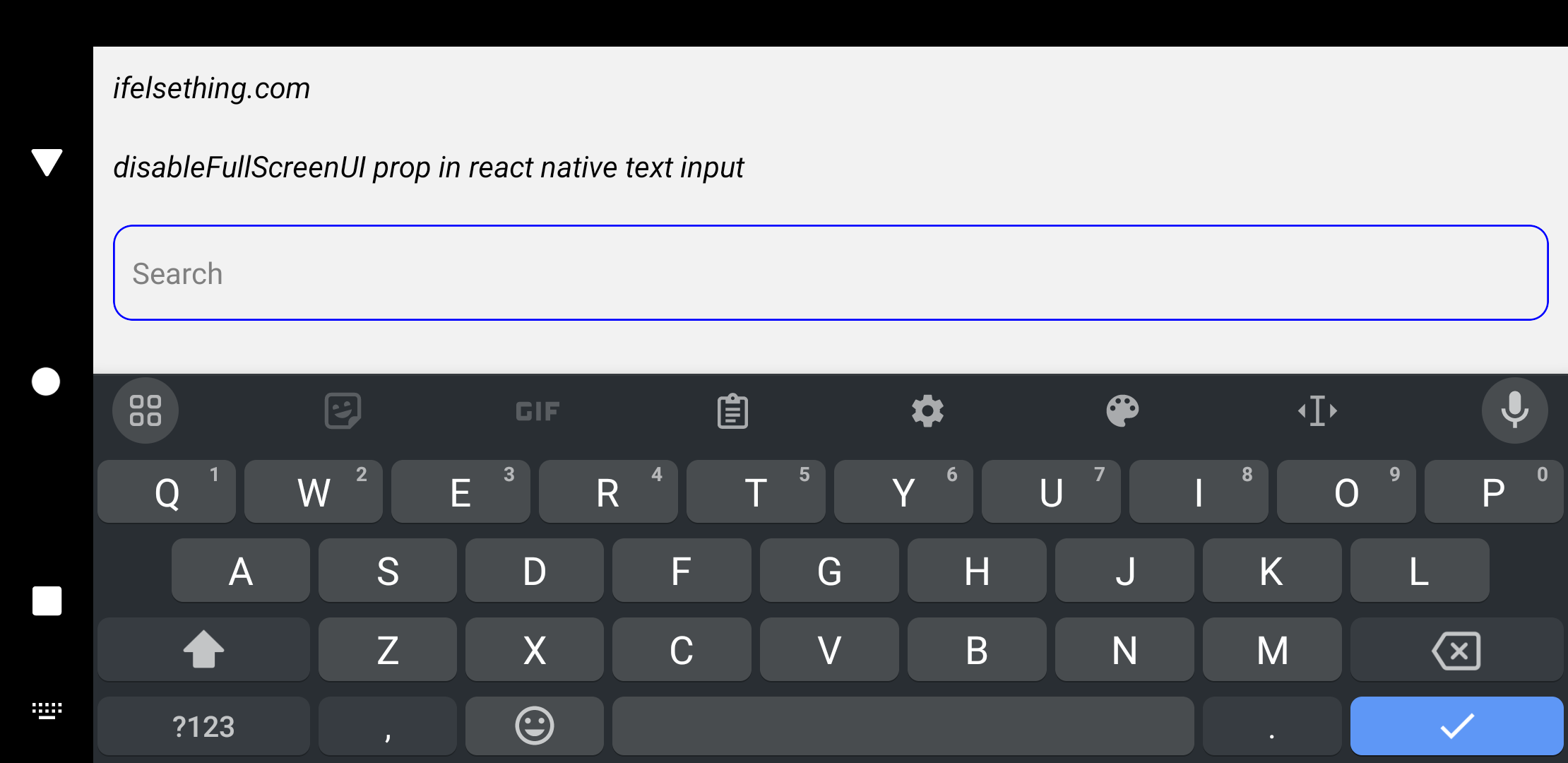
Complete code of our example,
//App.tsx
import {
View,
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
} from "react-native";
export default function App() {
return (
<SafeAreaView style={{ flex: 1 }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
disableFullScreenUI prop in react native text input
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Search"
placeholderTextColor='gray'
autoCorrect={false}
disableFullscreenUI={true}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
}
});