Disable Return Key on Empty TextInput
Published On: 2024-03-27
Posted By: Harish
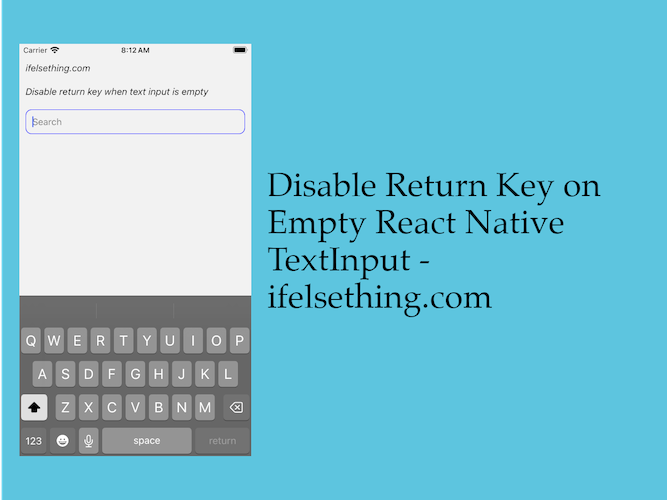
We generally check emptiness of text input in validations before doing any operations. Let's say we have a search input with a search button and we will search only when the text input is not empty after pressing the button. So in this case, we validate after pressing the button.
But what if your implementation does not have a search button and depends on the keyboard's return or enter key?
In this post, we will use enablesReturnKeyAutomatically prop to disable the return key when text input is empty in iOS. This prop is only available for iOS, so we will implement almost a similar behavior for android devices too.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will implement a simple search text input for this demo.
Import TextInput
component in App.tsx
file and add required props.
//App.tsx
...
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Search"
placeholderTextColor='gray'
showSoftInputOnFocus={true}
autoCorrect={false}
autoFocus={true}
/>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will notice that the return key for iOS and enter key for android are seen enabled even when text input is empty. We can validate the field inside the onSubmitEditing callback. But we can go a step further and disable the return
key when text input is empty.
This can be achieved by using enablesReturnKeyAutomatically
prop for iOS. It takes boolean values and default value is false
.
Add enablesReturnKeyAutomatically={true}
to text input and re-run the app in the iOS device/emulator.
...
<TextInput
...
enablesReturnKeyAutomatically={true}
/>
...
On load, you will notice the keyboard's return key will be in disabled state and automatically enabled when we enter text.
In this way, we can ignore empty text
validation because we can only submit when text is present.
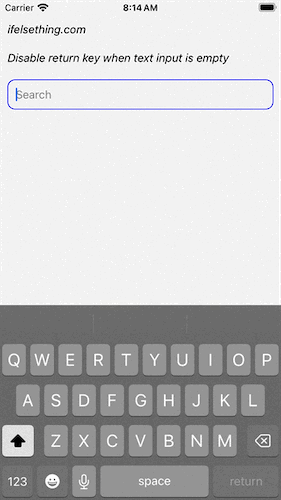
In android, we don't have any prop to disable enter key but we can achieve a similar behavior with the use of blurOnSubmit prop.
We will use and update blurOnSubmit value so that the keyboard stays open when text is empty and vice versa. And we will use onSubmitEditing callback only when the text input value is not empty.
Import useState
and declare a state to store text input value. Use this value to update blurOnSubmit prop. We update the state with the onChangeText
callback’s value and use this state to set blurOnSubmit
value.
Check below code for better understanding.
...
const [search, setSearch] = useState<string | undefined>(undefined);
...
<TextInput
...
value={search}
blurOnSubmit={search ? true : false}
onChangeText={setSearch}
onSubmitEditing={() => {
if (!search) return;
}}
/>
...
Above code acts like enablesReturnKeyAutomatically
prop for android devices. Optionally you can use Platform
to check and apply this code only for android devices but it's not necessary.
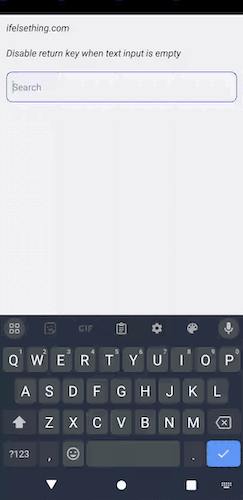
In the above gif, we can see that the keyboard does not close when we press enter for empty text input but closes when text input is not empty.
Complete code of our example,
//App.tsx
import { useState } from 'react';
import {
View,
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar
} from "react-native";
export default function App() {
const [search, setSearch] = useState<string | undefined>(undefined);
return (
<SafeAreaView style={{ flex: 1 }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Disable return key when text input is empty in iOS
</Text>
<View style={styles.input_block}>
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Search"
placeholderTextColor='gray'
showSoftInputOnFocus={true}
enablesReturnKeyAutomatically={true}
autoCorrect={false}
autoFocus={true}
value={search}
blurOnSubmit={search ? true : false}
onChangeText={setSearch}
onSubmitEditing={() => {
if (!search) return;
}}
/>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
flexGrow: 1,
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
borderColor: 'blue'
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
input_block: {
flexDirection: 'row',
alignItems: 'center',
},
});