Get React Native TextInput Width and Height on Render
Published On: 2024-04-12
Posted By: Harish
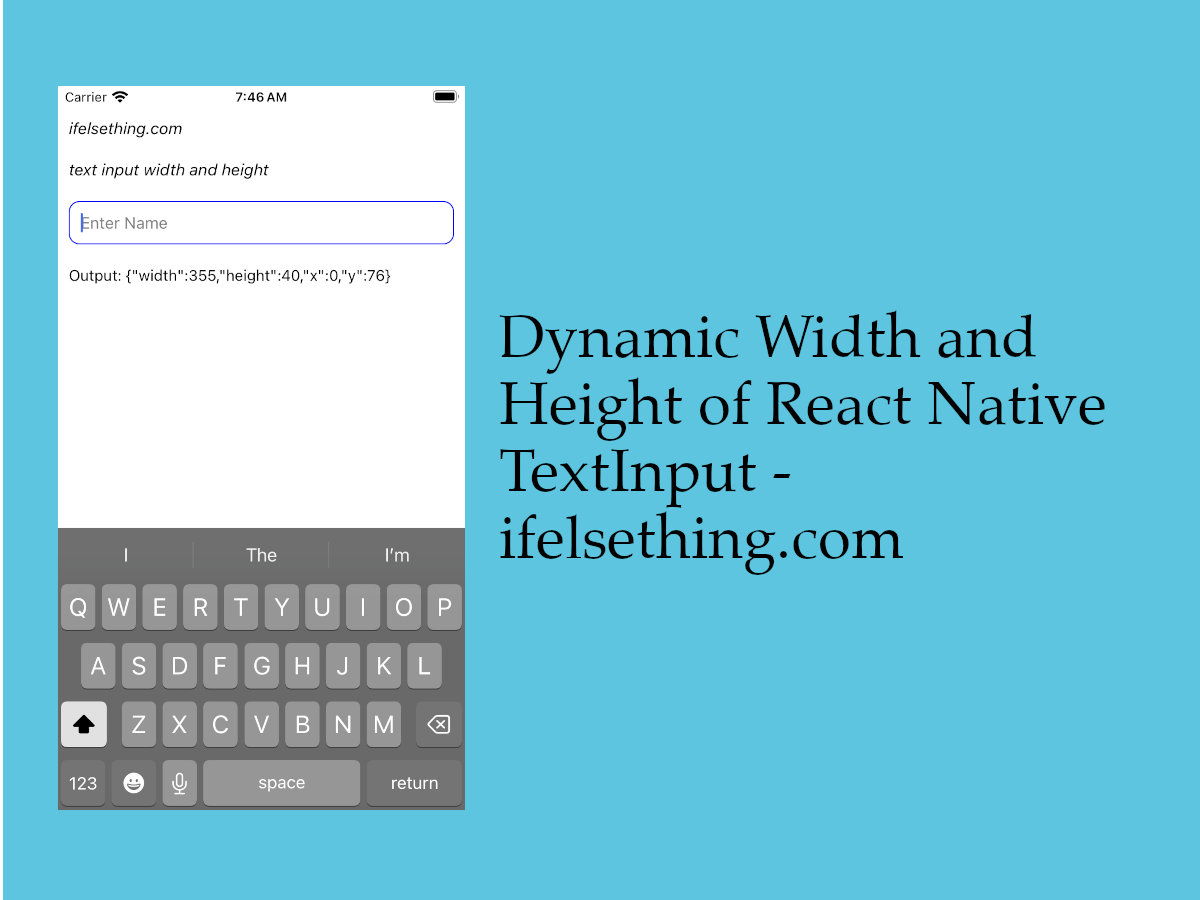
If we don't specify the width and height of the text input, then it may adapt default or dynamic size which adapts based on other screen UI components.
So, in this scenario we don't know the rendered size of the text input component by default but we can get the rendered width and height of the text input with the help of onLayout callback.
This callback returns an event with width, height and position properties. This callback not only triggers on launch, but also triggers whenever the size of the layout changes i.e., either width or height of input changes.
Let's see this callback example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will create a simple description text input.
Import the TextInput
component in the App.tsx
file and add basic required props for the description input.
And also onLayout
callback to it.
//App.tsx
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Description'
placeholderTextColor='gray'
onLayout={event => {
console.log(event.nativeEvent);
}}
/>
...
Run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
After rendering, you can see a log with details of width, height and position of the text input.
#iOS Log
LOG {"layout": {"height": 35, "width": 355, "x": 0, "y": 76}, "target": 405}
Above log is the default width and height of the input when the component renders.
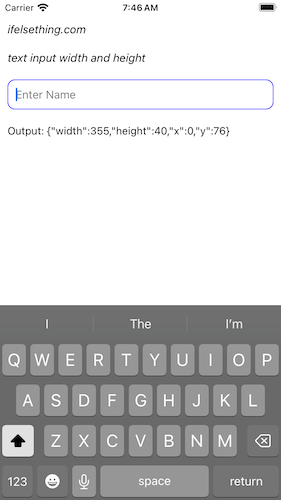
If the size of the input changes for any reason, this callback triggers again. To see this scenario, we can make text input as multiline which is similar to the textarea of html.
Add multiline={true}
to text input. To make text input grow width with content, make changes to input style.
...
<TextInput
...
style={styles.input}
multiline={true}
/>
...
input: {
...
alignSelf: 'flex-start',
}
...
With the above changes, text input will become small in width. Now enter text inside text input and you will see that the onLayout callback is triggered whenever input is increasing.
We have seen this type of behavior for onContentSizeChange callback which triggers when content inside text input changes.
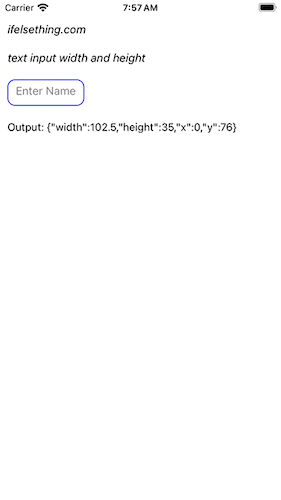
Complete code of our example,
//App.tsx
import React, { useState } from "react";
import {
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
View,
LayoutRectangle
} from "react-native";
export default function App() {
const [inputSize, setInputSize] = useState<LayoutRectangle | undefined>();
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
text input width and height
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Name'
placeholderTextColor='gray'
multiline={true}
onLayout={event => {
let size = event.nativeEvent.layout;
setInputSize(size);
}}
/>
<Text>
Output: {inputSize ? JSON.stringify(inputSize) : "Not Available"}
</Text>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
alignSelf: 'flex-start',
borderColor: 'blue',
borderRadius: 10,
borderWidth: 1,
fontSize: 15,
padding: 10,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});