Enable or Disable Scroll of React Native ScrollView
Published On: 2024-06-24
Posted By: Harish
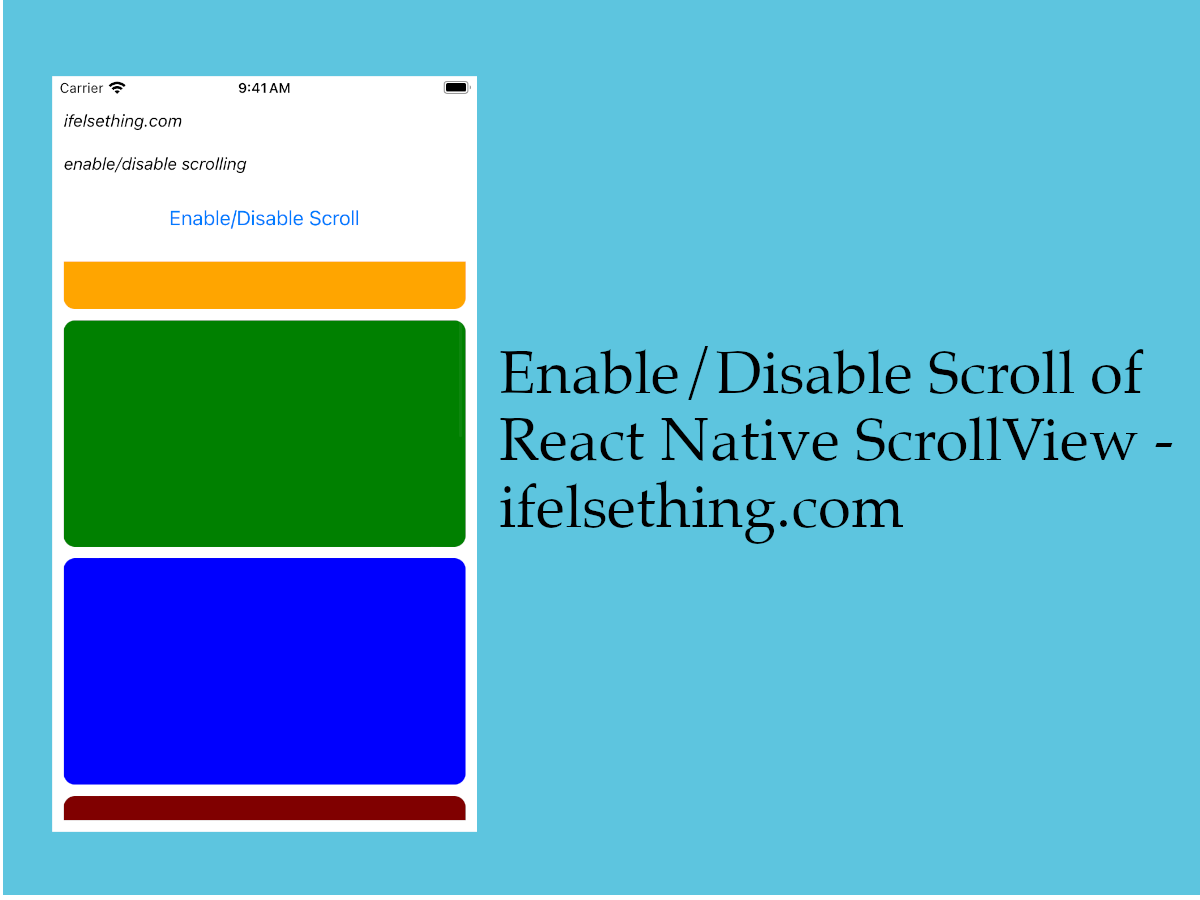
To make content scrollable, we use a ScrollView as a parent view. But what if you want a scrollview as the parent view and don't want its scrolling behavior?
In those scenarios, we can use scrollEnabled prop to enable or disable scrolling of scrollview. It accepts boolean values and the default value is true
. If the value false
is given, scrollview’s scroll gets disabled.
Let's check this prop's behavior with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ScrollViewRN
Example Implementation
We will create a simple scrollview with color blocks as content and we use scrollEnabled prop
to stop the scroll.
Import and add ScrollView
with few scrollable color blocks.
//App.tsx
...
import { View, ScrollView } from 'react-native';
...
<ScrollView
contentContainerStyle={styles.content_container}
>
{
colors
.map((color: string) => {
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
>
</View>
)
})
}
</ScrollView>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see a few scrollable color blocks.
Now add scrollEnabled={false}
to scrollview and try to scroll now.
...
<ScrollView
...
scrollEnabled={false}
>
...
</ScrollView>
...
You will notice that the content is locked and it does not scroll.
To have a clear understanding, we will use a state variable to enable or disable the scroll.
So, import useState
from react and a Button
component from react-native and create a variable to accept boolean values.
Toggle its value on press of the button and use that value to enable/disable the scroll. You can find the code implementation below.
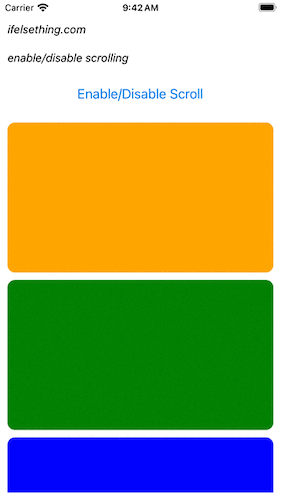
In this way we can disable the scrolling of scrollview content.
If you want to disable the scroll but want the content to start from a different position on load, use contentOffset prop.
Complete code of our example,
//App.tsx
import React, { useState } from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
ScrollView,
Button,
} from "react-native";
const colors = [
'orange',
'green',
'blue',
'maroon',
'violet',
'darkorange',
'gold',
'darkgreen',
'aquamarine',
'cadetblue'
];
export default function App() {
const [isScroll, setIsScroll] = useState<boolean>(true);
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
enable/disable scrolling
</Text>
<Button
onPress={() => setIsScroll((previous) => !previous)}
title="Enable/Disable Scroll"
/>
<ScrollView
scrollEnabled={isScroll}
contentContainerStyle={styles.content_container}
>
{
colors
.map((color: string) => {
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
>
</View>
)
})
}
</ScrollView>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
gap: 10
},
view: {
width: '100%',
height: 200,
borderRadius: 10
}
});