Enable or Disable React Native TextInput
Posted By: Harish
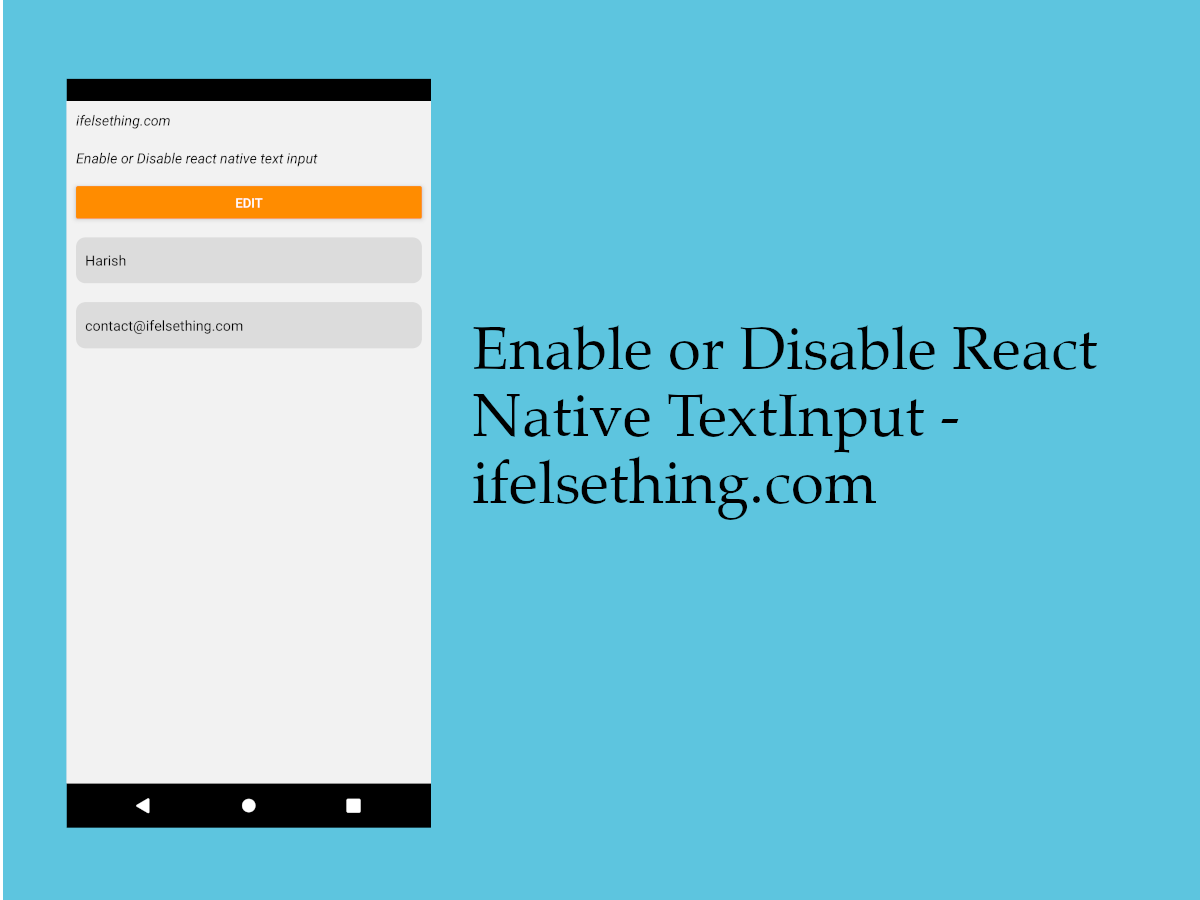
We have seen a way to disable copy and paste in text input. In this post we will see a way to directly disable text input, i.e., to disable typing in text input.
To disable a text input, we can use editable prop. This prop accepts boolean values. If the value is true
, text input can be editable, if false
, text input is disabled.
We also have readOnly prop, which does the same as editable
prop but values are opposite to editable prop. If readOnly
prop value is true
, text input is disabled, if false
input is enabled.
Only one of the above props should be used at a time. Lets implement a simple screen with editable prop.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will implement a simple profile screen, where programmatically enable or disable text input on the press of a button.
Import TextInput
and add two text input fields with default props. And to show default values, add defaultValue
prop with hard coded value. I'm hardcoding the value for demonstration purposes, generally we have to store the value in a state variable and pass that variable to value
prop.
And add editable={false}
prop to the first text input.
//App.tsx
...
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter Name"
placeholderTextColor='gray'
autoCorrect={false}
defaultValue="Harish"
autoComplete="off"
editable={false}
/>
<TextInput
style={styles.input}
keyboardType='email-address'
placeholder="Enter Email"
placeholderTextColor='gray'
autoCapitalize="none"
autoCorrect={false}
autoComplete="off"
defaultValue="contact@ifelsething.com"
/>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
You will notice that the first text input will not let you edit the text, meaning it is in disabled state.
Generally, for disabled states in HTML, input will change its color stating the user that it is in disabled state. But in react native, we don't have that functionality, we have to implement ourselves.
So, we will take a button and state variable. With on press action, the state variable will change its value. We then pass the value to editable prop to programmatically enable or disable the input.
Import Button
component from react-native
and useState
hook from react
. So, we will use a button to toggle boolean values and assign to a state variable to disable or enable input programmatically.
import { useState } from 'react';
import { Button } from 'react-native';
...
const [isEditable, setIsEditable] = useState(false);
...
<Button
onPress={() => setIsEditable(previousValue => !previousValue)}
/>
<TextInput
...
editable={isEditable}
/>
<TextInput
...
editable={isEditable}
/>
...
Editable value is set to false as we are showing a profile screen with data. They become editable when the user presses on the edit button.
We are also using this state variable to change colors of the button, and have a disable-state-look to text input. Check below code and gif for complete understanding.
If we replace editable
prop with readOnly
and interchange the boolean values, output will be the same.
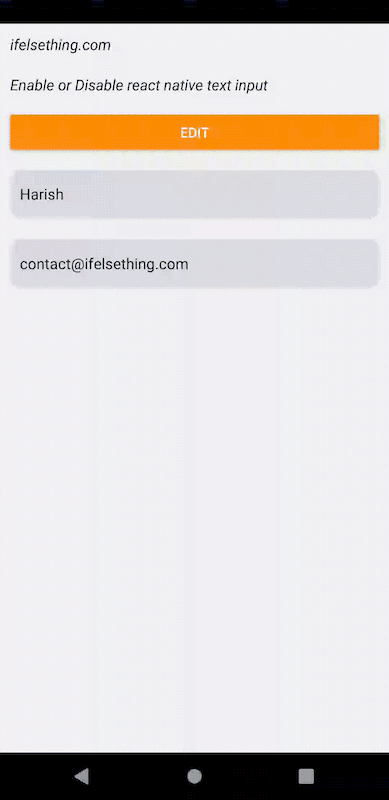
Complete code of our example,
//App.tsx
import { useState } from 'react';
import {
View,
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
Button
} from "react-native";
export default function App() {
const [isEditable, setIsEditable] = useState(false);
const getButtonTitle = () => {
return isEditable ? "Save" : "Edit";
};
const getButtonColor = () => {
return isEditable ? 'green' : 'darkorange';
};
const setBackgroundColor = () => {
return {
backgroundColor: !isEditable ? 'gainsboro' : 'transparent',
borderColor: !isEditable ? 'gainsboro' : 'blue'
};
};
return (
<SafeAreaView style={{ flex: 1 }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Enable or Disable react native text input
</Text>
<Button
onPress={() => setIsEditable(previousValue => !previousValue)}
title={getButtonTitle()}
color={getButtonColor()}
/>
<TextInput
style={[
styles.input,
setBackgroundColor()
]}
keyboardType='default'
placeholder="Enter Name"
placeholderTextColor='gray'
autoCorrect={false}
autoComplete="off"
editable={isEditable}
defaultValue="Harish"
/>
<TextInput
style={[
styles.input,
setBackgroundColor()
]}
keyboardType='email-address'
placeholder="Enter Email"
placeholderTextColor='gray'
autoCorrect={false}
autoComplete="off"
editable={isEditable}
autoCapitalize="none"
defaultValue='contact@ifelsething.com'
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
}
});