How to Focus Next React Native TextInput on Enter/Next Key Press?
Published On: 2024-02-20
Posted By: Harish
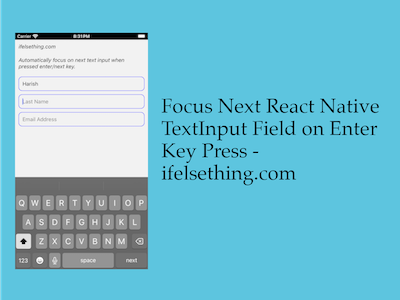
We have seen a way to focus on the next text input automatically based on length of characters.
In this post, we will see a way to focus on the next text input when a soft keyboard's next key is pressed. Here we will again use the useRef
hook to focus on text input programmatically.
For this example, we will create simple fields which we generally see in a registration form.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Add TextInputs
Add three <TextInput>
fields in the app.tsx
file. Add required props and the main props to add are autoFocus
and showSoftInputOnFocus
props for the first input field and others are applicable to all fields.
returnKeyType
prop changes the name of the keyboard's return key to applied value. placeholder
prop is to show placeholder text inside react native text input field, and placeholderTextColor
prop is to change the text color of the placeholder.
blurOnSubmit
prop removes the focus of the input field on pressing next key. This is important, because the keyboard closes when the key is pressed and again opens when the next input field is focused. So, blurOnSubmit={false}
is important to all fields other than the last field.
//app.tsx
import {TextInput} from 'react-native';
...
<TextInput
style={styles.input}
autoFocus={true}
showSoftInputOnFocus={true}
keyboardType='default'
returnKeyType='next'
blurOnSubmit={false}
placeholder="First Name"
placeholderTextColor='gray'
autoCapitalize='words'
autoCorrect={false}
/>
...
Now, create references to all <TextInput>
fields to focus programmatically using the useRef
hook.
import { useRef } from 'react';
...
export const App = () => {
const firstRef = useRef<any>(null);
const secondRef = useRef<any>(null);
const thirdRef = useRef<any>(null);
...
...
<TextInput
style={styles.input}
ref={firstRef}
...
/>
...
Using onSubmitEditing Callback
We have seen a way to focus on next or previous input programmatically using onKeyPress
and onChangeText
callbacks. But for this scenario, we can use onSubmitEditing
callback.
This callback is called when a next
key is pressed stating that the editing is completed. We will call the focus()
method in this onSubmitEditing callback.
...
//First text input will focus on second input
<TextInput
...
onSubmitEditing={(e) => {
const text = e.nativeEvent.text;
if(!text) return;
secondRef.current.focus();
}}
/>
...
Now after pressing the next key, the second input will get focused. Video below shows this functionality.
Complete code,
//app.tsx
import { useRef } from 'react';
import {
View,
Text,
StyleSheet,
TextInput,
} from "react-native";
export const App = () => {
const firstRef = useRef<any>(null);
const secondRef = useRef<any>(null);
const thirdRef = useRef<any>(null);
return (
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Automatically focus on next text input when pressed enter/next key.
</Text>
<View style={styles.block}>
<TextInput
ref={firstRef}
style={styles.input}
autoFocus={true}
showSoftInputOnFocus={true}
keyboardType='default'
returnKeyType='next'
blurOnSubmit={false}
placeholder="First Name"
placeholderTextColor='gray'
autoCapitalize='words'
autoCorrect={false}
onSubmitEditing={(e) => {
const text = e.nativeEvent.text;
if(!text) return;
secondRef.current.focus();
}}
/>
<TextInput
ref={secondRef}
style={styles.input}
keyboardType='default'
returnKeyType='next'
blurOnSubmit={false}
placeholder="Last Name"
autoCorrect={false}
placeholderTextColor='gray'
autoCapitalize='words'
onSubmitEditing={(e) => {
const text = e.nativeEvent.text;
if(!text) return;
thirdRef.current.focus();
}}
/>
<TextInput
ref={thirdRef}
style={styles.input}
keyboardType='email-address'
placeholder="Email Address"
placeholderTextColor='gray'
autoCapitalize='none'
returnKeyType='next'
autoCorrect={false}
onSubmitEditing={(e) => {
const text = e.nativeEvent.text;
if(!text) return;
}}
/>
</View>
</View>
);
}
const styles = StyleSheet.create({
container: {
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
block: {
flexGrow: 1,
gap: 10,
}
});