Get Event After Keyboard Opened or Closed in React Native
Published On: 2024-02-25
Posted By: Harish
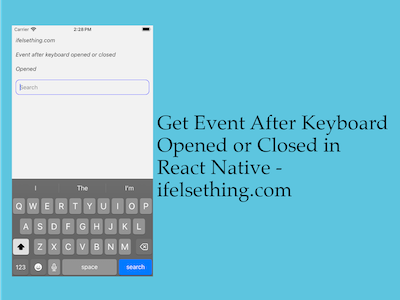
We have seen a way to get a keyboard event when it opens or hides. We used that event in our floating done button example to close the keyboard. But what if we want to know the event after it is completely opened or closed?
In this post we will create a simple screen where the user gets to see the result when the keyboard is completely opened or closed.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init KeyboardRN
Import Keyboard Module
We need to import a TextInput
, Text
and Keyboard
module.
To get events after the action, we need to call Keyboard.addListener()
with the event name and a callback function.
Here, we will show the status when the keyboard completely opens or closes. So let's add a text input field and a text to show the status.
//app.tsx
import { useState } from "react";
import {
Text,
TextInput,
...
} from "react-native";
...
export const App = () => {
const [isOpened, setIsOpened] = useState(false);
...
<Text style={styles.text}>
{ isOpened ? "Opened" : "Closed"}
</Text>
<TextInput
style={styles.input}
keyboardType='default'
returnKeyType='search'
placeholder="Search"
placeholderTextColor='gray'
autoFocus={true}
showSoftInputOnFocus={true}
/>
...
Created a isOpened
boolean to update according to events.
Now import useEffect
from react and add listeners to call on render. keyboardDidShow
and keyboardDidHide
are the event names which are called when opened or closed.
//app.tsx
...
useEffect(() => {
const showSub = Keyboard.addListener('keyboardDidShow', showKeyboard);
const hideSub = Keyboard.addListener('keyboardDidHide', hideKeyboard);
return () => {
showSub.remove();
hideSub.remove();
};
}, []);
const showKeyboard = (e: KeyboardEvent) => {
console.log("After Completely Show: ", e);
setIsOpened(true);
};
const hideKeyboard = (e: KeyboardEvent) => {
console.log("After Completely Hide: ", e);
setIsOpened(false);
};
...
We will get the events in showKeyboard
and hideKeyboard
functions. And we are updating the state value to true
or false
accordingly.
#logs
After Completely Show: {"duration": 0, "easing": "keyboard", "endCoordinates": {"height": 260, "screenX": 0, "screenY": 407, "width": 375}, "isEventFromThisApp": true, "startCoordinates": {"height": 260, "screenX": 0, "screenY": 407, "width": 375}}
After Completely Hide: {"duration": 250, "easing": "keyboard", "endCoordinates": {"height": 260, "screenX": 0, "screenY": 667, "width": 375}, "isEventFromThisApp": true, "startCoordinates": {"height": 260, "screenX": 0, "screenY": 407, "width": 375}}
We can see the status of the keyboard in the video below.
Complete code,
//app.tsx
import { useEffect, useState } from "react";
import {
View,
Text,
StyleSheet,
TextInput,
Keyboard,
KeyboardEvent
} from "react-native";
export const App = () => {
const [isOpened, setIsOpened] = useState(false);
useEffect(() => {
const showSub = Keyboard.addListener('keyboardDidShow', showKeyboard);
const hideSub = Keyboard.addListener('keyboardDidHide', hideKeyboard);
return () => {
showSub.remove();
hideSub.remove();
};
}, []);
const showKeyboard = (e: KeyboardEvent) => {
console.log("After Completely Show: ", e);
setIsOpened(true);
};
const hideKeyboard = (e: KeyboardEvent) => {
console.log("After Completely Hide: ", e);
setIsOpened(false);
};
return (
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Event after keyboard opened or closed
</Text>
<Text style={styles.text}>
{isOpened ? "Opened" : "Closed"}
</Text>
<TextInput
style={styles.input}
keyboardType='default'
returnKeyType='search'
placeholder="Search"
placeholderTextColor='gray'
autoFocus={true}
showSoftInputOnFocus={true}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});