Flash Scrollbar without Scrolling in React Native
Published On: 2024-06-26
Posted By: Harish
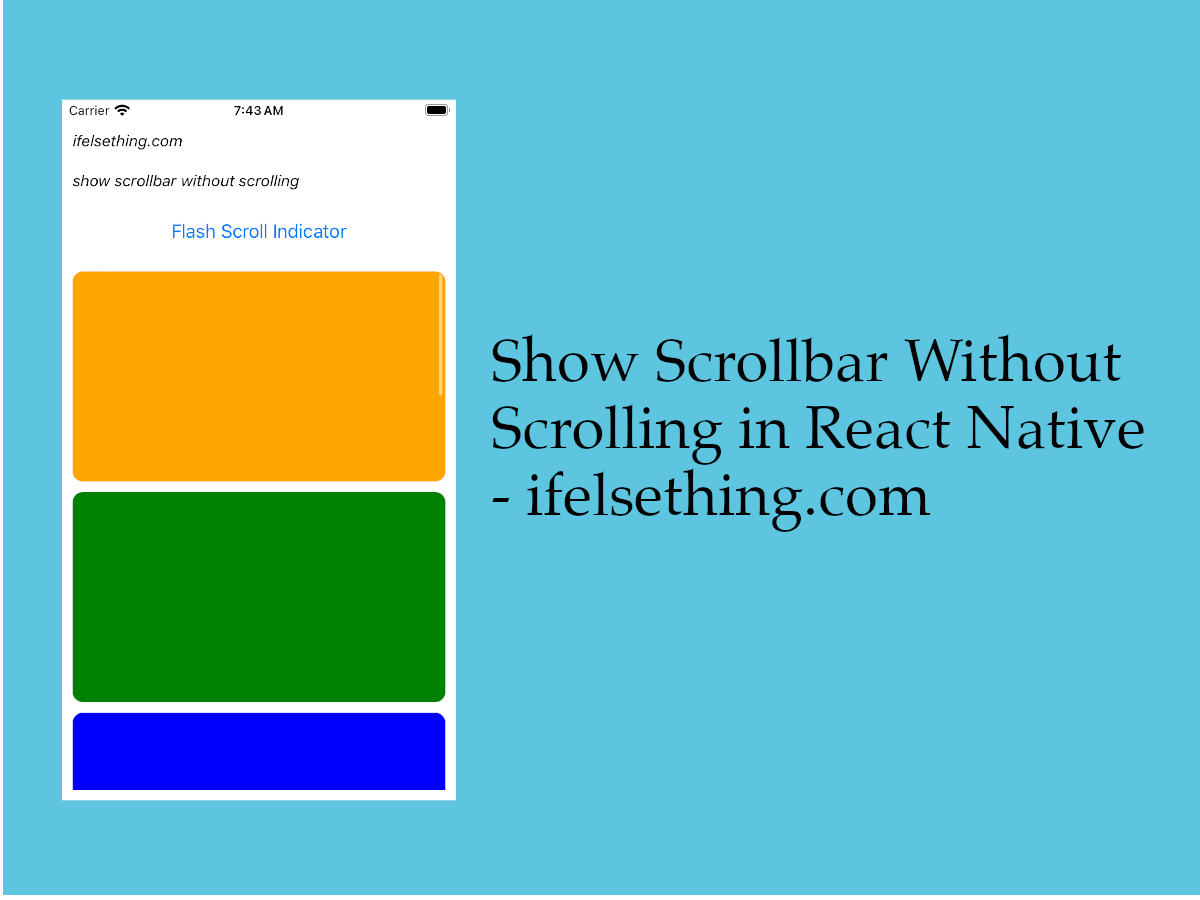
Scrollbar gives an idea of list length and item's position. But this scrollbar only shows on scroll and hides when scrolling momentum stops. And to see the scrollbar, we have to scroll the list again.
So, to show the scrollbar without scrolling, we have a scrollview method called flashScrollIndicators() which flashes the scrollbar when called.
When this method is called, the scrollbar will flash once and hide again. This can be useful to flash a scrollbar when new content is added to the current list and to let users know about the extra content to scroll. Let's see how this method flashes the scrollbar with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ScrollViewRN
Example Implementation
We will create a simple vertical scrollview with color blocks and show the scrollbar whenever we press a button.
Import and add ScrollView
with few scrollable color blocks.
//App.tsx
...
import { View, ScrollView } from 'react-native';
...
<ScrollView
contentContainerStyle={styles.content_container}
>
{
colors
.map((color: string) => {
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
>
</View>
)
})
}
</ScrollView>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see a few scrollable color blocks. If you scroll, you will see a light scrollbar at the right side of the list and hide when scrolling stops. Again to see the scrollbar, we have to scroll again.
So, to show the scrollbar without scrolling, we will use the flashScrollIndicators()
method.
To use that method, first we have to get the reference of the scrollview using useRef hook and use that hook to call the method on click of a button.
Import useRef
hook from react to create a null ScrollView reference and Import a Button
component to call the method on button press.
...
const ref = useRef<ScrollView>(null);
...
<Button
...
onPress={() => ref.current?.flashScrollIndicators()}
/>
...
<ScrollView
...
ref={ref}
>
...
</ScrollView>
...
After reloading, if we press the button, the scrollbar will be flashed.
If we call this method inside ScrollView’s onContentSizeChange method, the scrollbar will be flashed whenever new content is added or old content is removed.
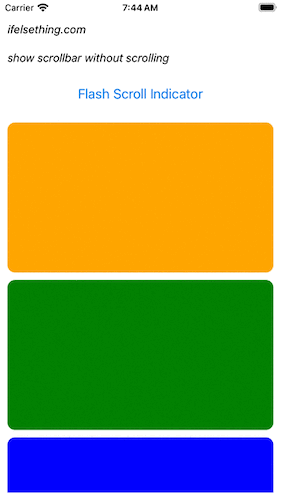
Complete code of our example,
//App.tsx
import React, { useRef } from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
ScrollView,
Button,
} from "react-native";
const colors = [
'orange',
'green',
'blue',
'maroon',
'violet',
'darkorange',
'gold',
'darkgreen',
'aquamarine',
'cadetblue'
];
export default function App() {
const ref = useRef<ScrollView>(null);
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
show scrollbar without scrolling
</Text>
<Button
onPress={() => ref.current?.flashScrollIndicators()}
title="Flash Scroll Indicator"
/>
<ScrollView
ref={ref}
contentContainerStyle={styles.content_container}
>
{
colors
.map((color: string) => {
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
>
</View>
)
})
}
</ScrollView>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
gap: 10
},
view: {
width: '100%',
height: 200,
borderRadius: 10
}
});