How to Focus on React Native TextInput using useRef Hook?
Published On: 2024-02-18
Posted By: Harish
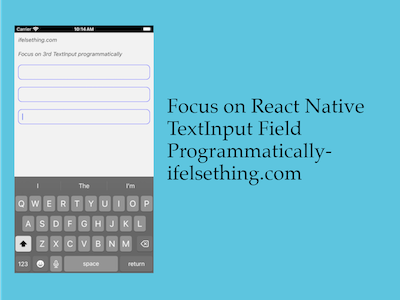
We have a way to auto focus on a react native text input field by using autoFocus
prop. But what if there is a need of conditional focusing on a TextInput field? We cannot use autoFocus
prop as we don't know when to apply and which text input to apply.
In these situations, for focusing on a text input field programmatically, we can use the useRef
hook.
Let's see an example,
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Add TextInput Fields
Let's import TextInput
from react and add three <TextInput>
fields in the app.tsx
file.
If we run the app, we can see that there is no focus on any of the text input fields.
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
Now, without using autoFocus
prop we will be using useRef hook to focus on the desired text input field.
Import useEffect and useRef from react. Initialize useRef with null value.
//app.tsx
import {useEffect, useRef} from 'react';
export const App = () => {
const textInputRef = useRef<any>(null);
...
After initialization, set the ref on the desired text input field. Here ref is nothing but a reference to the field. We can assign a ref to each field if needed.
We will assign this ref to the 3rd text input field.
...
<TextInput
ref={textInputRef}
style={styles.input}
/>
...
We got the reference of the text input field, now we can call the focus()
function of <TextInput>
to programmatically focus on the text input field.
Now, call the focus()
function inside the useEffect
hook.
...
useEffect(() => {
if (!textInputRef) return;
textInputRef.current.focus();
}, [textInputRef]);
...
In useEffect, we are calling the focus()
function to start focusing on the text input when the screen renders.
In this way we can see the same behavior of autoFocus
prop.
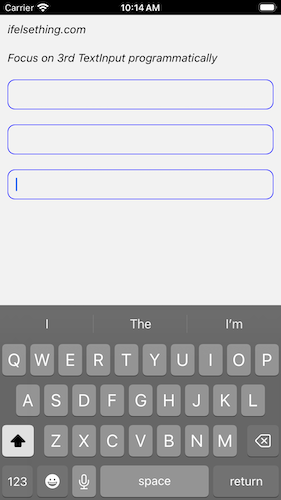
complete code,
//app.tsx
import { useEffect, useRef } from 'react';
import {
View,
Text,
StyleSheet,
TextInput
} from "react-native";
export const App = () => {
const textInputRef = useRef<any>(null);
useEffect(() => {
if (!textInputRef) return;
textInputRef.current.focus();
}, [textInputRef]);
return (
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Focus on 3rd TextInput programmatically
</Text>
<TextInput
style={styles.input}
/>
<TextInput
style={styles.input}
/>
<TextInput
ref={textInputRef}
style={styles.input}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'flex-start',
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
}
});