Get Value of React Native TextInput
Published On: 2024-04-10
Posted By: Harish
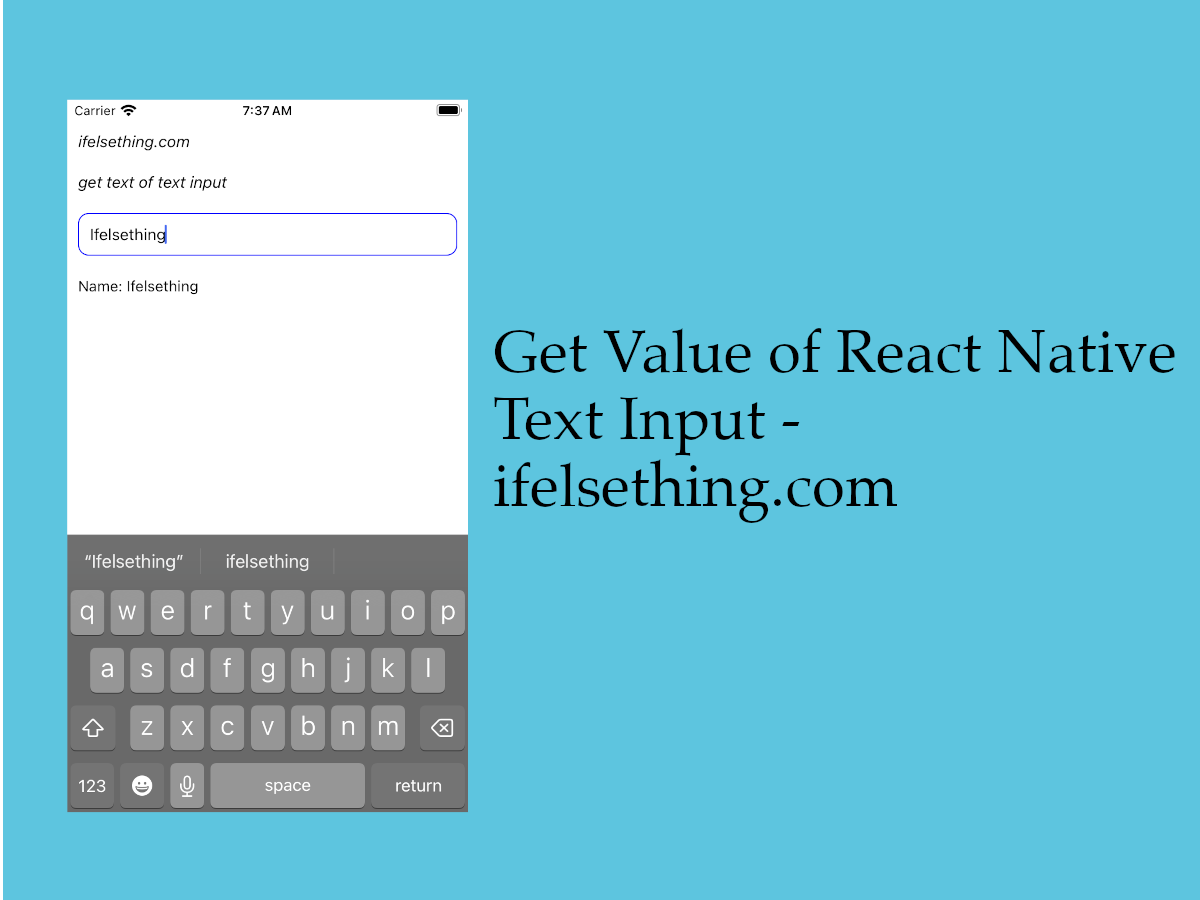
We can get the value of react native text input by using onChangeText callback or onChange callback.
The onChangeText
callback returns only text, whereas the onChange
callback returns a complete event of text input which includes text input value.
Let's see their implementation.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will create a simple text input for the name.
Import the TextInput
component in the App.tsx
file and add basic required props for name.
To get text input value, add onChangeText
callback function and log the value.
//App.tsx
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Name'
autoCorrect={false}
placeholderTextColor='gray'
onChangeText={(text: string) => {
console.log(text);
}}
/>
...
Run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
Now enter some text inside the text input to see the log of the value. So, we can see that the onChangeText
outputs text input value directly.
We can also get value from the complete event of onChange
callback.
Add onChange callback to text input.
...
<TextInput
...
onChange={event => {
const text = event.nativeEvent.text;
console.log(text);
}}
/>
...
As you can see from the above code, we can get text from the event's nativeEvent
object.
If we re-run the app and enter text, we can see both callbacks will log text input value. For this example, we will store the value in the state variable to show.
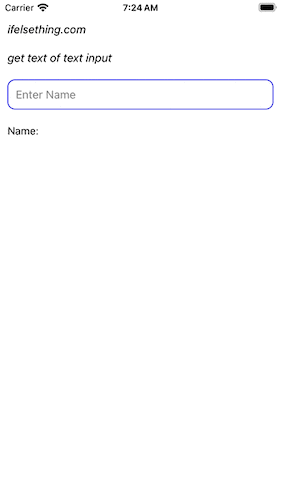
Complete code of our example,
//App.tsx
import { useState } from 'react';
import {
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
View,
} from "react-native";
export default function App() {
const [value, setValue] = useState<string | undefined>();
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
get text of text input
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Name'
placeholderTextColor='gray'
autoCorrect={false}
onChange={event => {
const text = event.nativeEvent.text;
setValue(text);
console.log(text);
}}
onChangeText={(text: string) => {
setValue(text);
console.log(text);
}}
/>
<Text>
Name: {value}
</Text>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderRadius: 10,
borderWidth: 1,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});