Header and Footer for React Native FlatList
Published On: 2024-09-08
Posted By: Harish
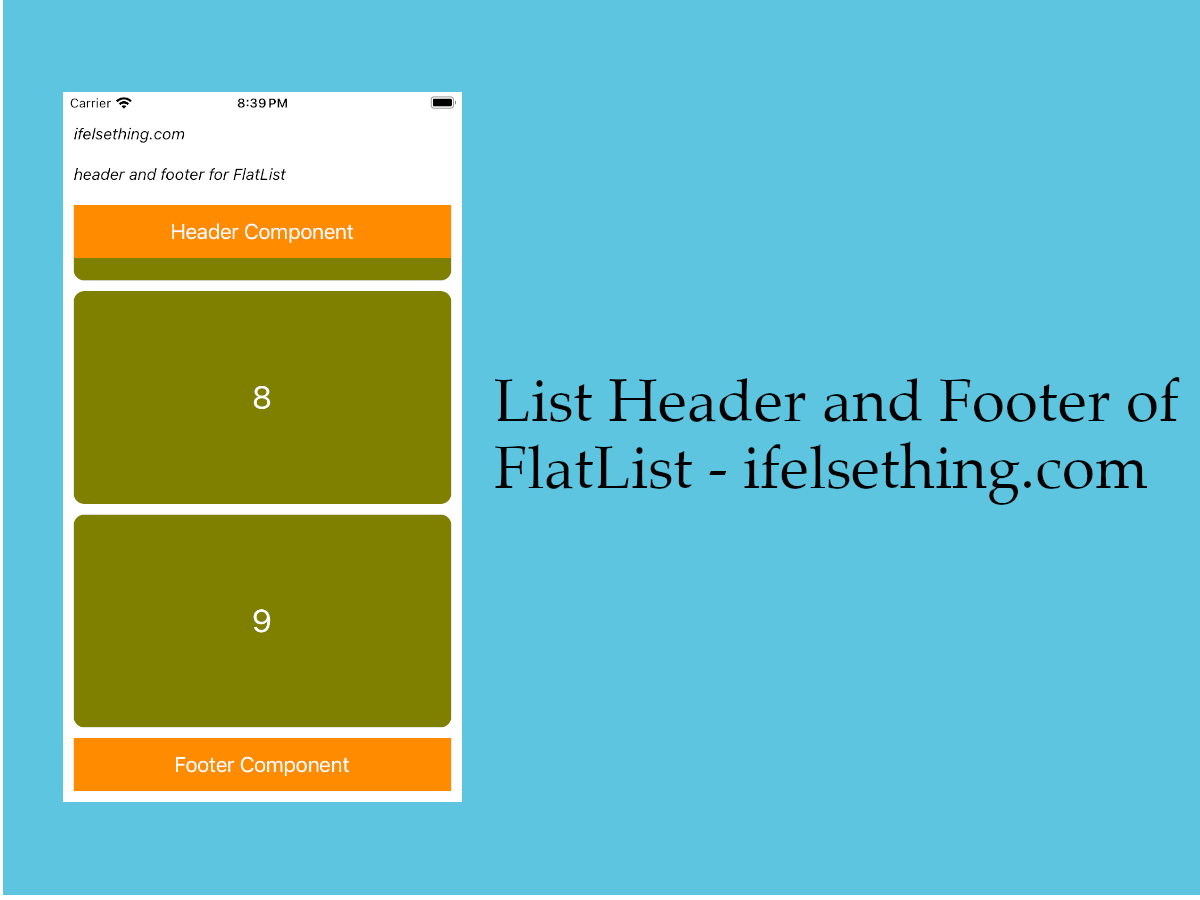
Sometimes a list may need a header or a footer or both. We have different types of headers like a sticky header in a ScrollViewr or multiple headers in a ScrollView. And when coming to list components like FlatList, we have a dedicated header and footer functional props to use.
In header and footer for VirtualizedList post, we have seen ListHeaderComponent
and ListFooterComponent
functional props to add header and footer to the list. As FlatList inherits VirtualizedList props, we can use those functional props in FlatList. So, with ListHeaderComponent, we can add a header to the FlatList and with ListFooterComponent, we can add a footer to the FlatList.
Let's see its usage with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init FlatListRN
Example Implementation
We will create a simple FlatList with blocks list, then we will add a header and a footer to the FlatList. We will also convert that header to a sticky header.
Import FlatList
from react-native and add to App.tsx
file. You can find more info about a basic FlatList by visiting FlatList with a basic example post.
//App.tsx
...
import { FlatList } from 'react-native';
...
<FlatList
contentContainerStyle={styles.content_container}
data={data}
keyExtractor={item => item + ""}
renderItem={({ item }) => {
return (
<Item item={item} />
)
}}
/>
...
If we run the app now,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see a simple blocks list.
Now, add ListHeaderComponent
and ListFooterComponent
functional props to the FlatList
.
I created a simple header and footer components for this post. Check out complete code down below.
...
<FlatList
...
ListHeaderComponent={() => <HeaderComponent />}
ListFooterComponent={() => <FooterComponent />}
/>
...
Now re-run the app to see the header and footer of the FlatList.
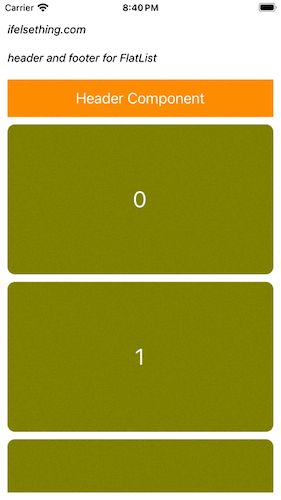
ListHeaderComponentStyle and ListFooterComponentStyle
If you observe our header and footer components, you will see a View wrapper around the text components. That view behaves as a container to text.
...
const HeaderComponent = () => {
return (
<View style={styles.header_footer}>
...
</View>
)
};
const FooterComponent = () => {
return (
<View style={styles.header_footer}>
...
</View>
)
};
...
But we can remove that View container by using ListHeaderComponentStyle and ListFooterComponentStyle style props.
When we add above props to FlatList, a view container is formed around header and footer automatically and mentioned styles will be applied to that container.
...
const HeaderComponent = () => {
return (
<Text style={styles.header_footer_text}>
Header Component
</Text>
)
};
const FooterComponent = () => {
return (
<Text style={styles.header_footer_text}>
Footer Component
</Text>
)
};
...
<FlatList
...
ListHeaderComponentStyle={styles.header_footer}
ListFooterComponentStyle={styles.header_footer}
...
/>
Sticky Header in FlatList
We can also make ListHeaderComponent a sticky header by using stickyHeaderIndices prop. By passing the header component's index to the prop, it becomes sticky. As the header is the starting item of the list, its index will be 0
.
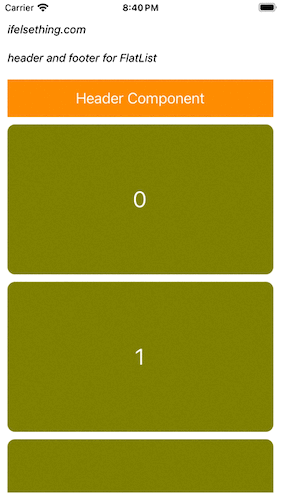
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
FlatList
} from "react-native";
export default function App() {
const data = [...Array(10).keys()];
const Item = ({ item }: { item: number }) => {
return (
<View
key={item}
style={styles.item_view}
>
<Text style={styles.item_text}>{item}</Text>
</View>
)
};
const HeaderComponent = () => {
return (
<View style={styles.header_footer}>
<Text style={styles.header_footer_text}>
Header Component
</Text>
</View>
)
};
const FooterComponent = () => {
return (
<View style={styles.header_footer}>
<Text style={styles.header_footer_text}>
Footer Component
</Text>
</View>
)
};
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
header and footer for FlatList
</Text>
<FlatList
contentContainerStyle={styles.content_container}
stickyHeaderIndices={[0]}
data={data}
keyExtractor={item => item + ""}
//ListHeaderComponentStyle={styles.header_footer}
//ListFooterComponentStyle={styles.header_footer}
ListHeaderComponent={() => <HeaderComponent />}
ListFooterComponent={() => <FooterComponent />}
renderItem={({ item }) => {
return (
<Item item={item} />
)
}}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
flexGrow: 1,
gap: 10
},
item_view: {
width: '100%',
height: 200,
borderRadius: 10,
backgroundColor: 'olive',
alignItems: 'center',
justifyContent: 'center'
},
item_text: {
color: 'white',
fontSize: 30,
},
header_footer: {
flex: 1,
backgroundColor: 'darkorange',
justifyContent: 'center',
alignItems: 'center',
height: 50
},
header_footer_text: {
fontSize: 20,
color: 'white',
},
});