Hide Content of React Native TextInput
Posted By: Harish
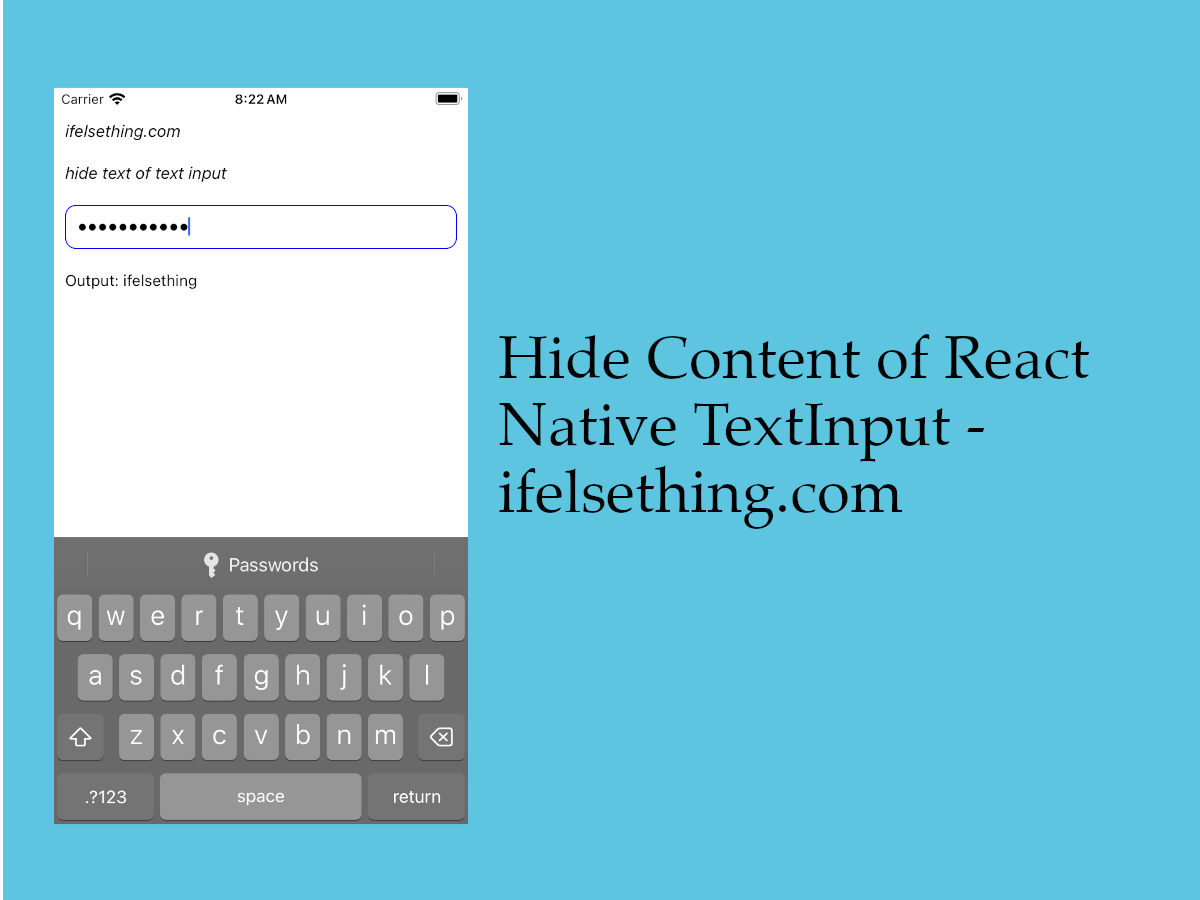
If you have a text input which takes confidential info, we can hide that content using a secureTextEntry prop.
Most commonly used confidential input is the password. So, we can hide the entered password in text input by using secureTextEntry
prop with true
value. Default value is false
.
Lets see this prop with an example. More functional hide/show password example implementation can be seen from how to use icons inside react native text input post.
In this post, I will only implement a simple hidden password entry field.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will create a simple password text input with the hidden value.
Import the TextInput
component in the App.tsx
file and add basic required props for the password input.
Add secureTextEntry
prop with true
value.
//App.tsx
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
autoCorrect={false}
autoCapitalize="none"
placeholder='Enter Password'
placeholderTextColor='gray'
secureTextEntry={true}
/>
...
Run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
After the render, enter random text inside text input and you will see that the text is replaced with dots.
Everything is similar to normal text input like we can get text value using onChangeText callback. Only visual text will be hidden for the user.
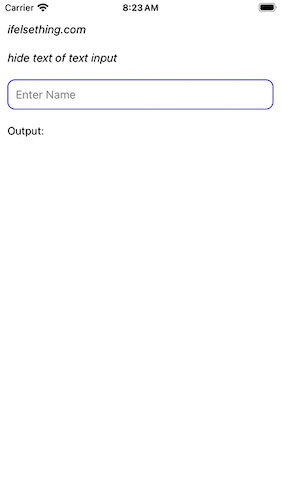
Complete code of our example,
//App.tsx
import React, { useState } from "react";
import {
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
View
} from "react-native";
export default function App() {
const [value, setValue] = useState<string | undefined>();
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
hide text of text input
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Name'
placeholderTextColor='gray'
secureTextEntry={true}
value={value}
onChangeText={setValue}
/>
<Text>
Output: {value}
</Text>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderRadius: 10,
borderWidth: 1,
fontSize: 15,
padding: 10,
color: 'black'
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});