Hide Keyboard on Focus of React Native TextInput
Published On: 2024-04-13
Posted By: Harish
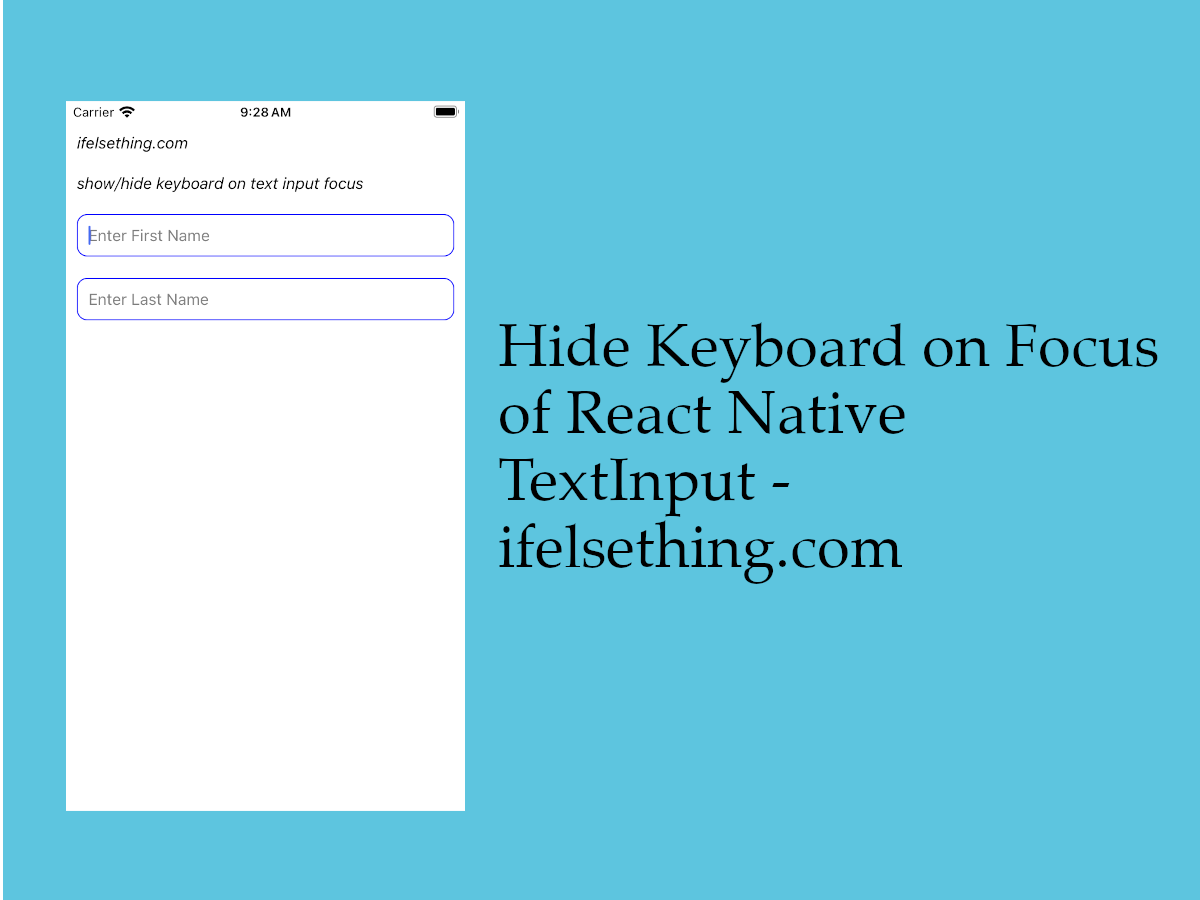
In general, the soft keyboard will open when text input is focused. But we can also control the text input keyboard state using showSoftInputOnFocus prop.
This prop accepts boolean values and default value is true
. If false
, even when text input is focused, the soft keyboard will not open.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will create two text inputs with one false value and another one true value.
Import the TextInput
component in the App.tsx
file and add two text inputs with basic required props.
Add showSoftInputOnFocus
prop with false
value for the first input field and with true
value for the second field. We don't need to add this prop if we want to open the keyboard on focus as the default value is true
. But for this post example we will be using this prop even for true value.
//App.tsx
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter First Name'
placeholderTextColor='gray'
showSoftInputOnFocus={false}
/>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Last Name'
placeholderTextColor='gray'
showSoftInputOnFocus={true}
/>
...
Run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
After the render, if you tap on the first field, the keyboard will not open even if the cursor starts blinking. But if you focus on the second field, the keyboard will open with a blinking cursor.
Even if we programmatically focus text input using the ref or by tapping, when the showSoftInputOnFocus
value is false, the keyboard will not open.
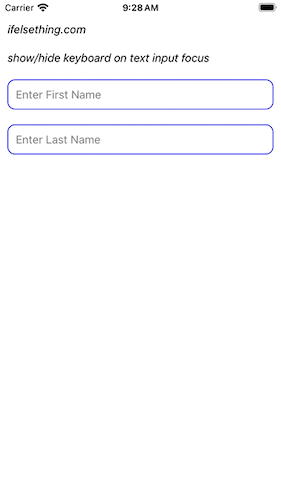
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
View
} from "react-native";
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
show/hide keyboard on text input focus
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter First Name'
placeholderTextColor='gray'
showSoftInputOnFocus={false}
/>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Last Name'
placeholderTextColor='gray'
showSoftInputOnFocus={true}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderRadius: 10,
borderWidth: 1,
fontSize: 15,
padding: 10,
color: 'black'
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});