Hide Keyboard on Scroll in React Native
Posted By: Harish
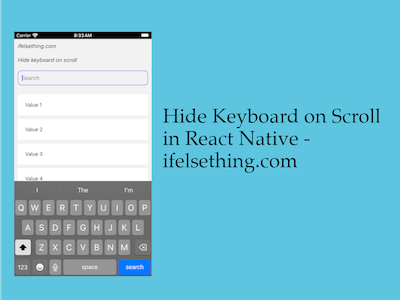
We discussed a way to dismiss keyboard when tapped outside of text input field. In this post we will see a way to dismiss the opened keyboard when scroll begins.
For this example, we will implement a simple search page to show the behavior of dismissing the keyboard when scroll begins.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Add a Search Field and a List
Import TextInput
, TouchableOpacity
and ScrollView
from react-native
, add text input for search, scrollview for scrollable list in the app.tsx
file.
Now to close the keyboard when scroll begins, we have to use onScrollBeginDrag
callback. This callback is called when scroll drag starts.
Import the Keyboard
module from react-native
and call Keyboard.dismiss()
function inside onScrollBeginDrag callback.
To get touch events when the keyboard is opened in scrollview, we have to use keyboardShouldPersistTaps
prop which we discussed in the keyboard inside scrollview post example using a button.
//app.tsx
import {
...
Keyboard
} from "react-native";
...
<ScrollView
style={{ flex: 1 }}
keyboardShouldPersistTaps='handled'
onScrollBeginDrag={Keyboard.dismiss}
>
...
...
</ScrollView>
...
Now, the keyboard will dismiss when scrolling of the list begins. This can be seen from the video below.
Complete code,
//app.tsx
import {
View,
Text,
StyleSheet,
TextInput,
ScrollView,
Keyboard,
TouchableOpacity
} from "react-native";
export const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Hide keyboard on scroll
</Text>
<TextInput
style={styles.input}
keyboardType='default'
returnKeyType='search'
placeholder="Search"
placeholderTextColor='gray'
autoFocus={true}
showSoftInputOnFocus={true}
/>
<ScrollView
style={{ flex: 1 }}
keyboardShouldPersistTaps='handled'
onScrollBeginDrag={Keyboard.dismiss}
>
<View style={{ flex: 1 }}>
{
[...Array(20).keys()].map((v: number) => {
return (
<TouchableOpacity key={v}>
<Text style={styles.item_text}>
Value {v+1}
</Text>
</TouchableOpacity>
)
})
}
</View>
</ScrollView>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
item_text: {
backgroundColor: 'white',
padding: 20,
marginVertical: 5
}
});