Hide Keyboard of TextInput on Outside Tap
Posted By: Harish
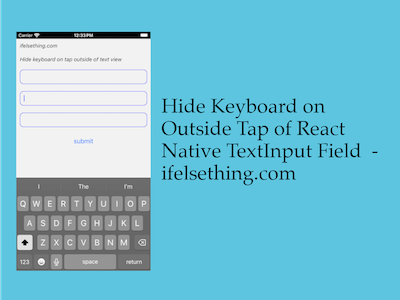
We generally tap outside of the text input to dismiss the keyboard. In this post, we will see a way to dismiss the opened keyboard on tap outside of the react native text input field.
This is for the text input field(s) inside a View
without a ScrollView
component. You can easily hide the keyboard inside ScrollView
by using its keyboardShouldPersistTaps
prop. You can find our example HERE.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Add TextInput Fields and Pressable Component
Add three TextInput
fields in the app.tsx
file. For closing the keyboard on the outside tap of text input, we have to import the Keyboard
module from react-native
and use Keyboard.dismiss()
function to dismiss it.
To use the Keyboard.dismiss()
function, we need a way to capture outside clicks. We can get feedback by using the Pressable
component. Pressable is a core component for getting touch events feedback. We can also use the TouchableWithoutFeedback
component instead of pressable, but using Pressable
is good.
Wrap the text input field inside Pressable
after importing. And call the Keyboard.dismiss()
function inside the onPress
callback. This onPress applies to the complete view, so if tapped anywhere inside the pressable view, the keyboard will close.
If there is a functionality of using many text inputs without the need of ScrollView
, then wrap all text inputs inside a single View
and wrap this view with a Pressable
component.
If inputs are inside the scrollview, then the opened keyboard will close by default on tap, but we have to use a prop for better functionality. Check out this post for more info.
//app.tsx
import {TextInput, Pressable, Keyboard} from 'react-native';
...
<Pressable style={{ flex: 1 }} onPress={Keyboard.dismiss}>
<View style={styles.container}>
<TextInput
style={styles.input}
keyboardType='default'
/>
...
If text inputs of the screen are hidden behind the opened keyboard because of their placement, then you should use KeyboardAvoidingView component to automatically adjust the view.
Complete code,
//app.tsx
import {
View,
Text,
StyleSheet,
TextInput,
Platform,
Button,
KeyboardAvoidingView,
Keyboard,
Pressable
} from "react-native";
export const App = () => {
return (
<KeyboardAvoidingView
style={{ flex: 1 }}
behavior={
Platform.OS === 'ios'
? 'padding'
: 'height'
}
>
<Pressable style={{ flex: 1 }} onPress={Keyboard.dismiss}>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Hide keyboard on tap outside of text view
</Text>
<TextInput
style={styles.input}
keyboardType='default'
/>
<TextInput
style={styles.input}
keyboardType='default'
/>
<TextInput
style={styles.input}
keyboardType='default'
/>
<Button title="submit" />
</View>
</Pressable>
</KeyboardAvoidingView>
);
}
const styles = StyleSheet.create({
container: {
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});