Horizontal FlatList
Published On: 2024-09-11
Posted By: Harish
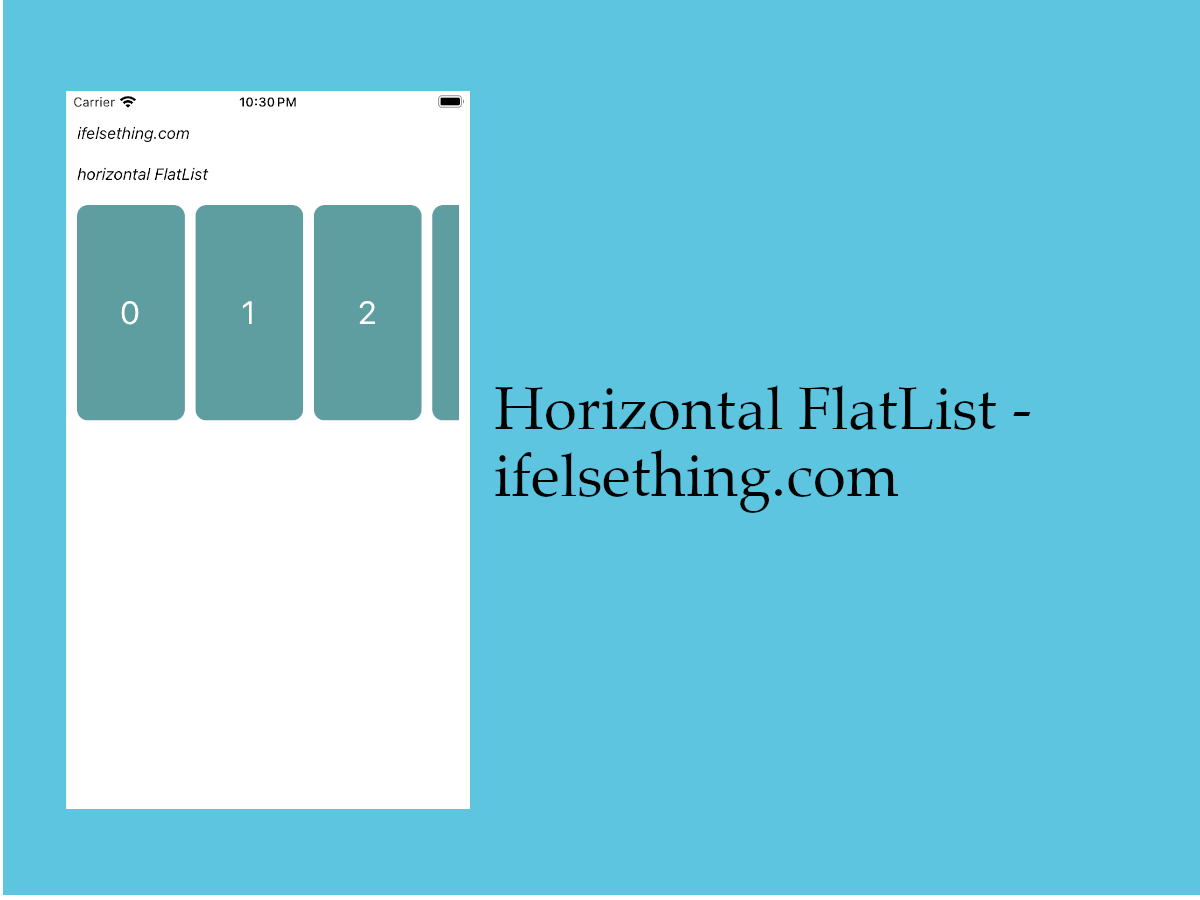
By default, a FlatList is a vertical list. But we can convert that list into a horizontal list by just using a prop.
With a horizontal prop, we can have a horizontal FlatList. We have seen this same prop to convert a vertical VirtualizedList into horizontal list or a vertical ScrollView to horizontal ScrollView.
This prop accepts a boolean value and false
is the default value. If we want a horizontal list, just pass a true
value or simply add a horizontal
prop without any value.
Let's convert a vertical FlatList into horizontal FlatList.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init FlatListRN
Example Implementation
We will create a simple vertical FlatList and convert that to a horizontal FlatList.
Import FlatList
from react-native and add to App.tsx
file. You can find more info about a basic FlatList by visiting FlatList with a basic example post.
//App.tsx
...
import { FlatList } from 'react-native';
...
<FlatList
contentContainerStyle={styles.content_container}
data={data}
keyExtractor={item => item + ""}
renderItem={({ item }) => {
return (
<Item item={item} />
)
}}
/>
...
If we run the app now,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see a simple vertical list.
Now, add horizontal
prop to the FlatList
with true
value.
...
<FlatList
...
horizontal={true}
/>
...
For our example, we have to make changes to the style of the block too. We used 100%
width of the block’s container to display in vertical orientation.
But in horizontal orientation, as the content scrolls horizontally, actual width will not be the container's width. So, we will fix the width of the blocks to 100
.
Now re-run the app to see a horizontal list.
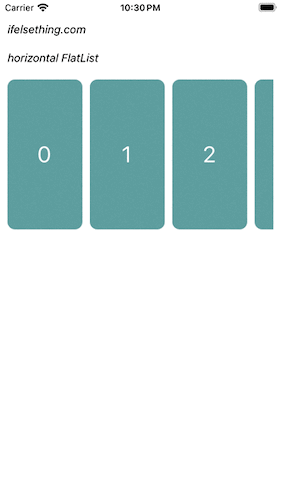
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
FlatList
} from "react-native";
export default function App() {
const data = [...Array(10).keys()];
const Item = ({ item }: { item: number }) => {
return (
<View
key={item}
style={styles.item_view}
>
<Text style={styles.item_text}>{item}</Text>
</View>
)
};
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
horizontal FlatList
</Text>
<FlatList
contentContainerStyle={styles.content_container}
data={data}
keyExtractor={item => item + ""}
horizontal //or horizontal={true}
renderItem={({ item }) => {
return (
<Item item={item} />
)
}}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
gap: 10
},
item_view: {
width: 100,
height: 200,
borderRadius: 10,
backgroundColor: 'cadetblue',
alignItems: 'center',
justifyContent: 'center'
},
item_text: {
color: 'white',
fontSize: 30,
}
});