Horizontal VirtualizedList
Published On: 2024-07-18
Posted By: Harish
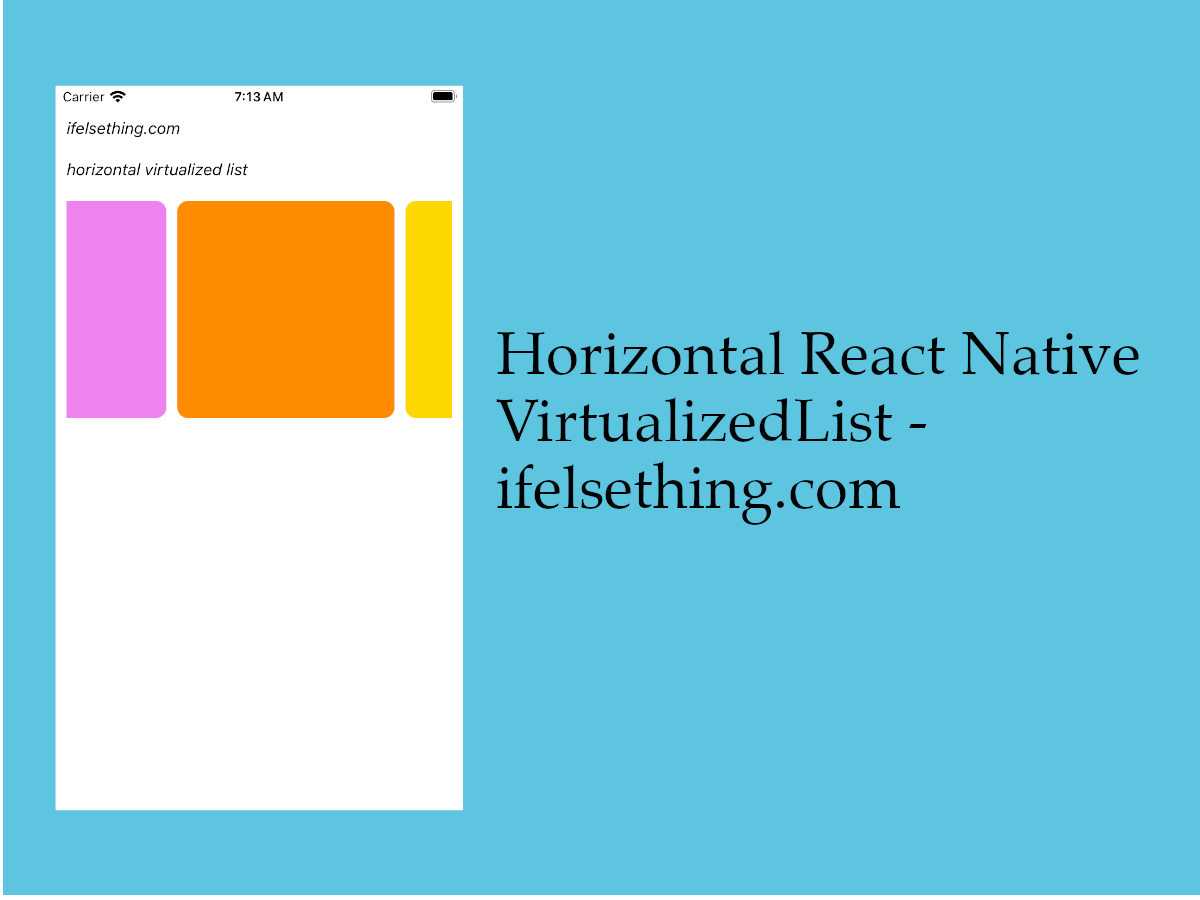
By default, a VirtualizedList component will output vertical scrollable list. We can make it horizontal by adding a simple horizontal prop.
This prop accepts boolean values and is false
by default. If true
, list will be horizontal and for false
, list will be vertical.
This is similar to ScrollView horizontal list prop.
Let's check this prop’s working with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init VListRN
Example Implementation
We will create a simple vertical VirtualizedList and convert it to horizontal.
Import and add VirtualizedList
. Add basic VirtualizedList with color blocks. Check basic VirtualizedList implementation for more info.
//App.tsx
...
import { VirtualizedList } from 'react-native';
...
<VirtualizedList
contentContainerStyle={styles.content_container}
data={colors}
getItemCount={getItemCount}
getItem={getItem}
keyExtractor={item => item.id}
renderItem={({ item }) => {
return (
<Item color={item.color} />
)
}}
/>
...
Run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
You will see a few colored blocks in a vertical scrollable list.
Now, to make this list as a horizontal scrollable list, add horizontal
prop with a true
value or simply add horizontal prop without any value. If only prop is added, its value is considered to be true.
And also we have to change the block's view size. Previously, we made its width 100%
to take the screen's width. As we changed its orientation to horizontal, we need to have a fixed block width. So, for this example, we fixed it to 200
. See styles block of complete code below.
...
<VirtualizedList
...
horizontal={true}
/>
...
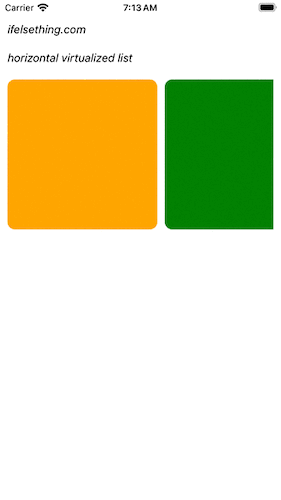
So, if we add horizontal prop, the list will be horizontal. If not, the list will be vertical.
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
VirtualizedList,
} from "react-native";
const colors = [
'orange',
'green',
'blue',
'maroon',
'violet',
'darkorange',
'gold',
'darkgreen',
'aquamarine',
'cadetblue'
];
type ItemProp = {
color: string;
};
type ItemDataProp = {
id: string;
color: string;
};
export default function App() {
const Item = (props: ItemProp) => {
const { color } = props;
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
/>
)
};
const getItem = (data: string[], index: number) => {
return {
id: Math.random().toString(12).substring(0),
color: data[index]
} as ItemDataProp
};
const getItemCount = (data: any) => data.length;
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
horizontal virtualized list
</Text>
<VirtualizedList
contentContainerStyle={styles.content_container}
data={colors}
horizontal
getItemCount={getItemCount}
getItem={getItem}
keyExtractor={item => item?.id}
renderItem={({ item }) => {
return (
<Item color={item.color} />
)
}}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
gap: 10
},
view: {
flexShrink: 1,
width: 200,
height: 200,
borderRadius: 10
}
});