Get Error Event when Image Fails to Load in React Native
Published On: 2024-05-16
Posted By: Harish
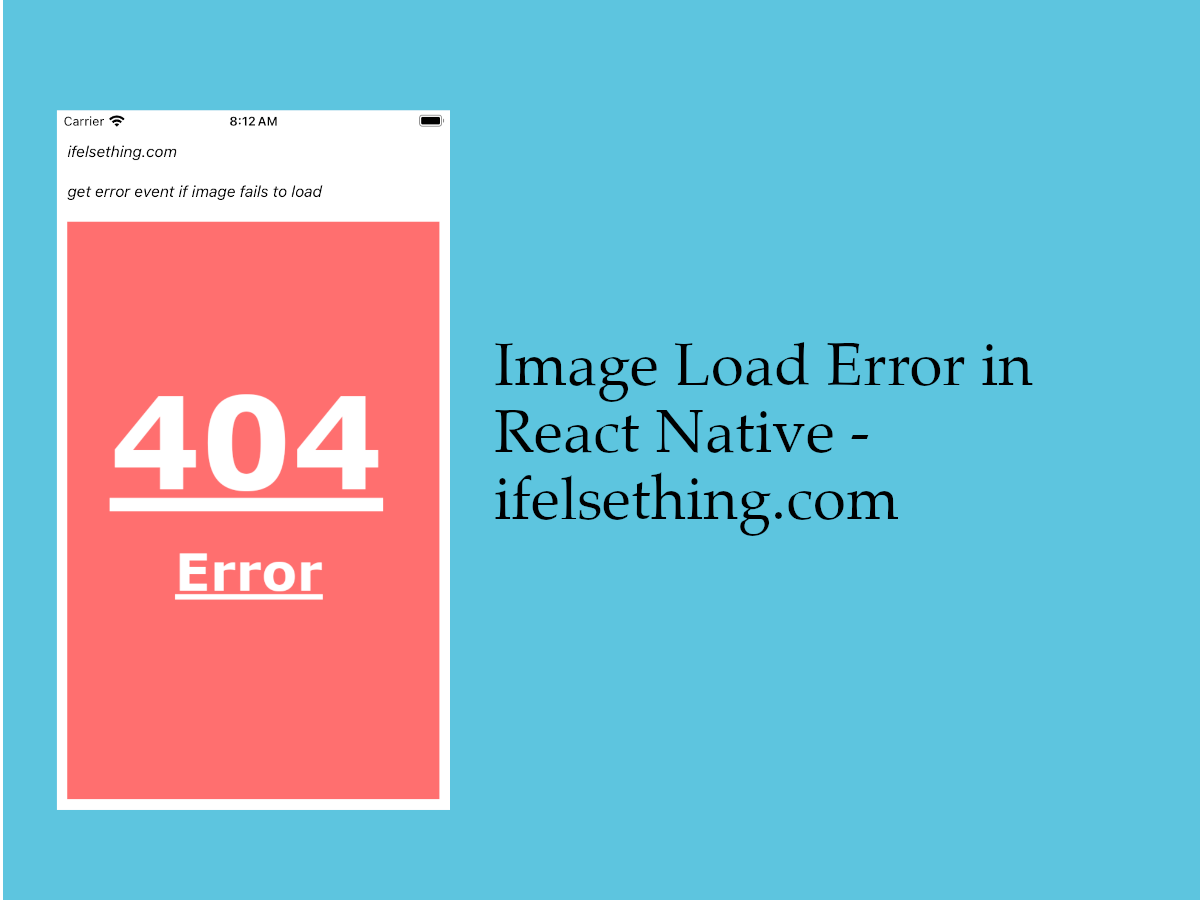
We have seen how to show a remote image in react native. If the image loads successfully, we can see the image. But what if it fails?
For local image errors, we will get build errors even before building the app. But this is not the case for remote images. So, we need to know when the load fails to show an error image.
We can get the error event with onError callback. This callback returns an image error event when a given remote URL image fails to load.
Lets see this in action.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ImageRN
Example Implementation
We will create a simple image component with the wrong remote image url.
Import and add Image
component with a source and a default image. First we will load a working url to check its behavior.
//App.tsx
...
import { Image } from 'react-native';
...
<Image
style={styles.image}
source={{
uri: 'https://iet-images.s3.ap-south-1.amazonaws.com/fav.png',
}}
defaultSource={require('./assets/default-image.png')}
resizeMode="cover"
/>
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see a default image at first and the remote image after it loads.
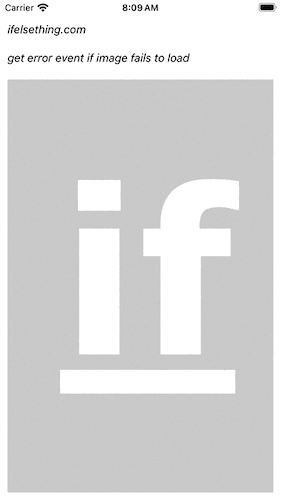
Now we will add an onError
callback to the image component and purposely give a broken remote url to get load error.
...
<Image
...
source={{
uri: 'https://iet-images.s3.ap-south-1.amazonaws.com/fav' //removed .png extension
}}
onError={() => {
console.log("Image Load Error");
}}
/>
...
If we reload the app, first we will see the default image and error console log.
We can use this error callback to show a different image if needed.
For this post, we will show an error image.
Import useState
from react and take a state variable for boolean values. Initially, this value will be false
and later when onError
callback is invoked, we will set the value to true
.
We will use this boolean value to show a local error image file.
...
const [error, setError] = useState<boolean>(false);
...
<Image
...
source={
error
? require('./assets/404-error.png')
: {
uri: 'https://iet-images.s3.ap-south-1.amazonaws.com/fav'
}
}
onError={(e) => {
setError(true);
}}
/>
...
If we reload the app, we can see the default image and later error image because of wrong remote image url.
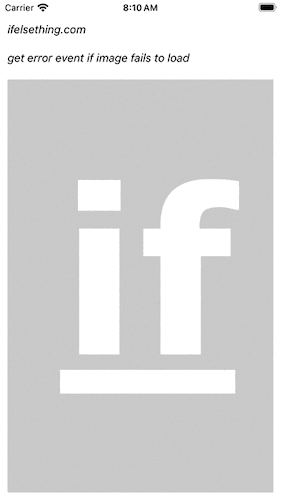
Complete code of our example,
//App.tsx
import React, { useState } from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
Image,
} from "react-native";
export default function App() {
const [error, setError] = useState<boolean>(false);
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
get error event if image fails to load
</Text>
<Image
style={styles.image}
source={
error
? require('./assets/404-error.png')
: {
uri: 'https://iet-images.s3.ap-south-1.amazonaws.com/fav'
}
}
resizeMode="cover"
defaultSource={require('./assets/default-image.png')}
onError={(e) => {
setError(true);
}}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
image: {
flex: 1,
width: '100%'
}
});