Change Image Color in React Native
Published On: 2024-05-18
Posted By: Harish
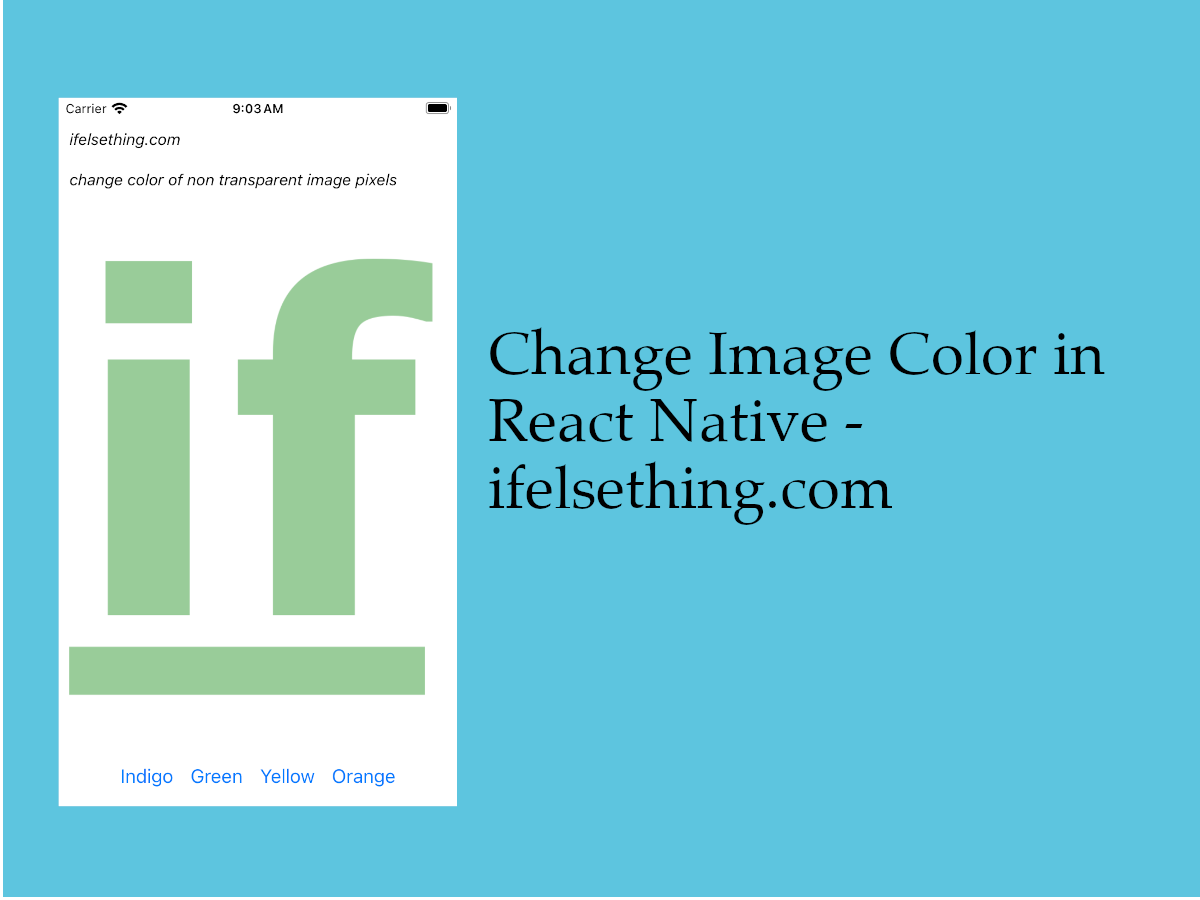
We can change the color of a non-transparent image pixels with tintColor prop.
For this to work, we should use a png image with a transparent background.
Let's see how it works.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ImageRN
Example Implementation
We will create a simple local image screen with different colors to change.
Import and add Image
component with a local transparent background image file.
//App.tsx
...
import { Image } from 'react-native';
...
<Image
style={styles.image}
resizeMode="cover"
source={require('./assets/fav-transparent.png')}
/>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
Original image will be loaded.
Now to change image color, add tintColor
prop and give a color value.
...
<Image
...
tintColor="indigo"
/>
...
If we reload the app, we can see the image with the given color. Please note that this will not apply any color to transparent pixels.
To apply different colors, I imported a Button
component and useState
to set the color on the press of a button.
We can also change the color intensity with an opacity style property.
You can find the complete code below.
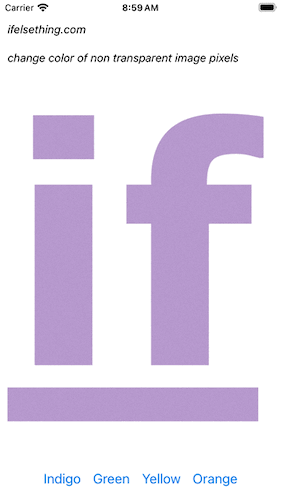
Complete code of our example,
//App.tsx
import React, { useState } from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
Image,
Button,
} from "react-native";
export default function App() {
const [tint, setTint] = useState('indigo');
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
change color of non transparent image pixels
</Text>
<Image
tintColor={tint}
style={styles.image}
resizeMode="cover"
source={require('./assets/fav-transparent.png')}
/>
<View style={styles.button_block}>
<Button
title="Indigo"
onPress={() => setTint("indigo")}
/>
<Button
title="Green"
onPress={() => setTint("green")}
/>
<Button
title="Yellow"
onPress={() => setTint("yellow")}
/>
<Button
title="Orange"
onPress={() => setTint("orange")}
/>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
image: {
flexShrink: 1,
width: '100%',
opacity: 0.4 //color intensity
},
button_block: {
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'center'
}
});