Invert VirtualizedList
Published On: 2024-07-18
Posted By: Harish
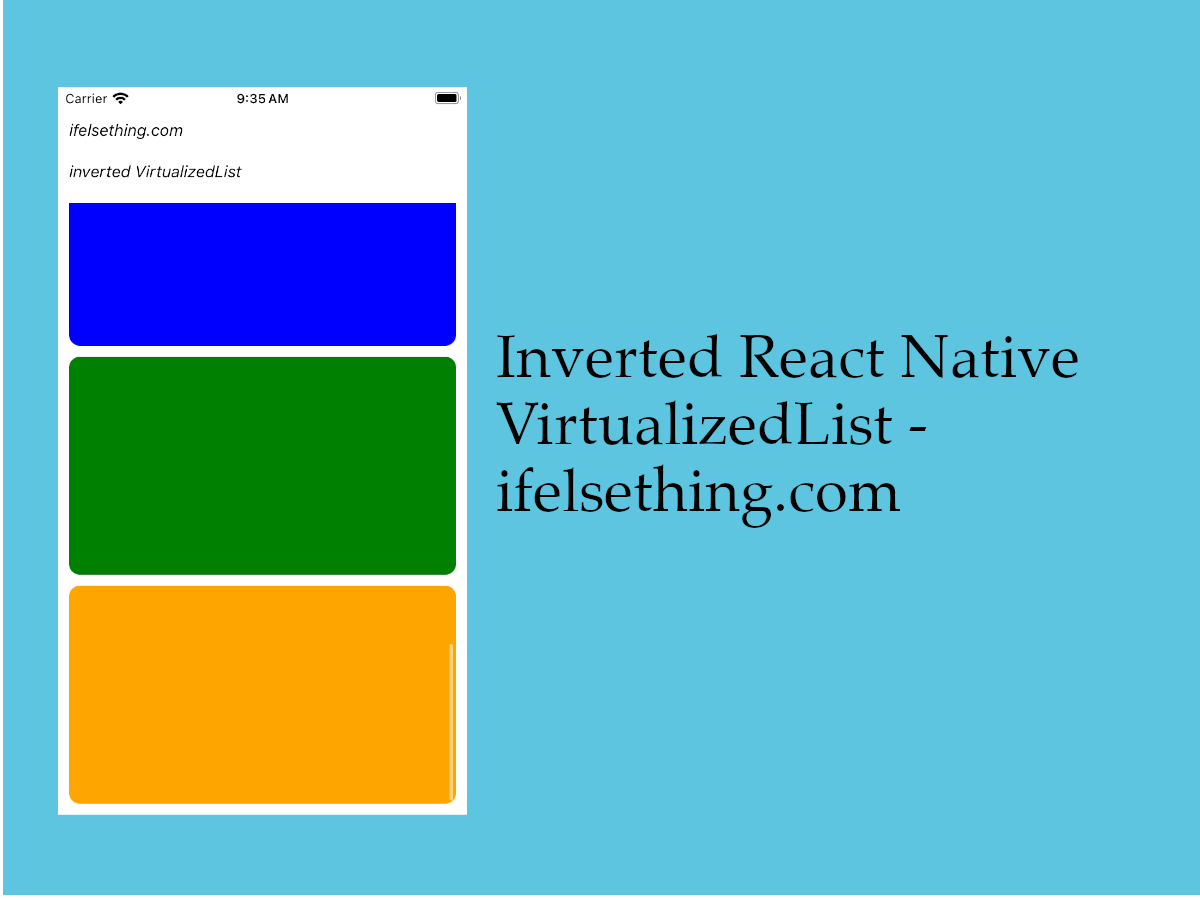
We can reverse the list data by using the .reverse()
method of the javascript array. Passing data to the VirtualizedList with reversed array, shows the list in reverse order.
Let's take a scenario of chat messages. By default, the latest messages will be seen at the bottom of the screen, which can be achieved with a reversed messages list. But this is a bad practice and this even results in bad performance when 100's of list items are present.
So, in those cases, we can use inverted prop to reverse the list order and to start the scroll from bottom of the list.
Think of the above mentioned chat messages example, with inverted prop, the latest messages will be seen at the bottom of the list and the scrolling starts from bottom too. Here, we assume that the array has the latest messages as starting elements in the array, so that the latest messages come to bottom when inverted prop is used.
Let's check this prop’s working with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init VListRN
Example Implementation
We will create a simple vertical VirtualizedList and invert them using the prop.
Import and add VirtualizedList
. Add basic VirtualizedList with color blocks. Check basic VirtualizedList implementation for more info.
//App.tsx
...
import { VirtualizedList } from 'react-native';
...
<VirtualizedList
contentContainerStyle={styles.content_container}
data={colors}
getItemCount={getItemCount}
getItem={getItem}
keyExtractor={item => item.id}
renderItem={({ item }) => {
return (
<Item color={item.color} />
)
}}
/>
...
If you run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
You will see a few colored blocks in a vertical scrollable list.
From our colors array list, we can see that the starting color to render is orange
followed by a green
color.
Now, add inverted
prop to VirtualizedList and re-run the metro builder.
...
<VirtualizedList
...
inverted={true}
/>
...
On render, you will notice that the orange
is at the bottom of the list and the scroll starts from bottom of the list.
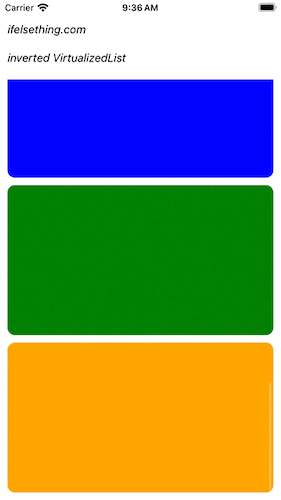
So, inverted prop reverses the list and starts the scroll from bottom of the list.
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
VirtualizedList,
} from "react-native";
const colors = [
'orange',
'green',
'blue',
'maroon',
'violet',
'darkorange',
'gold',
'darkgreen',
'aquamarine',
'cadetblue'
];
type ItemProp = {
color: string;
};
type ItemDataProp = {
id: string;
color: string;
};
export default function App() {
const Item = (props: ItemProp) => {
const { color } = props;
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
/>
)
};
const getItem = (data: string[], index: number) => {
return {
id: Math.random().toString(12).substring(0),
color: data[index]
} as ItemDataProp
};
const getItemCount = (data: any) => data.length;
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
inverted VirtualizedList
</Text>
<VirtualizedList
contentContainerStyle={styles.content_container}
data={colors}
getItemCount={getItemCount}
getItem={getItem}
inverted
keyExtractor={item => item?.id}
renderItem={({ item }) => {
return (
<Item color={item.color} />
)
}}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
gap: 10
},
view: {
width: '100%',
height: 200,
borderRadius: 10
}
});