Keep React Native Keyboard Open Even After Submit
Published On: 2024-02-29
Posted By: Harish
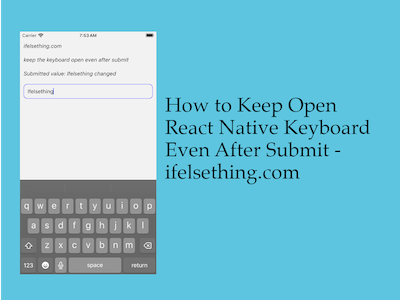
Generally react native’s text input keyboard closes when we submit the value pressing enter/return key. But what if we don't want to close the keyboard even after submitting the value?
In those scenarios, we can use blurOnSubmit prop. This prop is responsible for adding or removing focus from the text input when pressed submit button. By default its value is true
. That's the reason the keyboard closes automatically after submission.
Let's check with implementation.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init KeyboardRN
Import TextInput Component
Import TextInput
component and add basic props to it.
//app.tsx
import {
StyleSheet,
TextInput,
} from "react-native";
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter Text"
placeholderTextColor='gray'
/>
...
To check the blurOnSubmit property, we need a way to know when the next/return key is tapped to submit the value. For that, we have onSubmitEditing callback, which returns the submit event when pressed the enter key.
So, add onSubmitEditing callback to the text input and console log the text value from the NativeSyntheticEvent event return type. Please note that onSubmitEditing callback is not available in iOS if keyboardType="phone-pad".
...
<TextInput
...
onSubmitEditing={(e: NativeSyntheticEvent<TextInputSubmitEditingEventData>) => {
const value = e.nativeEvent.text;
console.log("Submitted Value: ", value)
}}
/>
...
Now run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
If we enter some text and press submit, we will see that the callback is called and keyboard is closed automatically by removing focus.
Now, to keep the keyboard open, add blurOnSubmit prop to text input with false
value.
...
<TextInput
...
blurOnSubmit={false}
/>
...
If we run the app again, and press submit button, the value will be logged inside the onSubmitEditing callback, but the keyboard stays open with focus on the text input.
I used the onSubmitEditing callback for explanation purposes. Not needed with blurOnSubmit
prop if you don't want any submit event.
From the gif below, you can see that the keyboard does not close even after pressing the submit/return button.
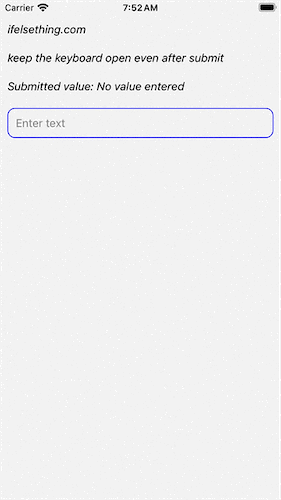
Complete code,
//App.tsx
import { useState } from "react";
import {
View,
Text,
StyleSheet,
TextInput
} from "react-native";
export const App = () => {
const [value, setValue] = useState<string | undefined>();
return (
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
keep the keyboard open even after submit
</Text>
<Text style={styles.text}>
Submitted value: {value ? value : "No value entered"}
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
onSubmitEditing={(e) => {
const v = e.nativeEvent.text;
setValue(v);
}}
blurOnSubmit={false}
autoCorrect={false}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});