Using keyboardShouldPersistTaps Prop of ScrollView
Published On: 2024-02-21
Posted By: Harish
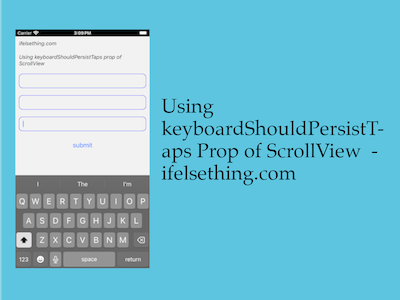
We already discussed about a way to dismiss keyboard when tapped outside of text input field. That is a preferred process when text input fields are not wrapped inside a ScrollView
component.
If text inputs are inside a scrollview, then the keyboard will be closed by default. But if scrollview has any other components like a submit
button, then we should use keyboardShouldPersistTaps
prop to register tha tap of the button before closing the opened keyboard.
Let's check the functionality in action.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Add TextInput Fields and a Button inside ScrollView Component
Import TextInput
and ScrollView
from react-native
, add scrollview, three TextInput
fields and a Button
in the app.tsx
file.
If we tap on the button when the keyboard is in an opened state, the button will not get triggered, instead it dismisses the opened keyboard. We have to tap again on the button to carry on its functionality.
To overcome this behavior, we have to use the keyboardShouldPersistTaps
prop. This prop accepts never
, always
and handled
values. You can find more info HERE.
In our case, add handled
value to get events from the submit button even when the keyboard is opened.
//app.tsx
import {
Button,
ScrollView
} from "react-native";
...
export const App = () => {
return (
<ScrollView
style={{ flex: 1 }}
keyboardShouldPersistTaps='handled'
>
...
Use KeyboardAvoidingView component to push the complete scrollview upwards when keyboard opens..
Complete code,
//app.tsx
import {
View,
Text,
StyleSheet,
TextInput,
Platform,
Button,
KeyboardAvoidingView,
ScrollView
} from "react-native";
export const App = () => {
return (
<KeyboardAvoidingView
style={{ flex: 1 }}
behavior={
Platform.OS === 'ios'
? 'padding'
: 'height'
}
>
<ScrollView
style={{ flex: 1 }}
keyboardShouldPersistTaps='handled'
>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Using keyboardShouldPersistTaps prop of ScrollView
</Text>
<TextInput
style={styles.input}
keyboardType='default'
/>
<TextInput
style={styles.input}
keyboardType='default'
/>
<TextInput
style={styles.input}
keyboardType='default'
/>
<Button title="submit" />
</View>
</ScrollView>
</KeyboardAvoidingView>
);
}
const styles = StyleSheet.create({
container: {
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});