Limit Text Length of React Native TextInput
Published On: 2024-04-09
Posted By: Harish
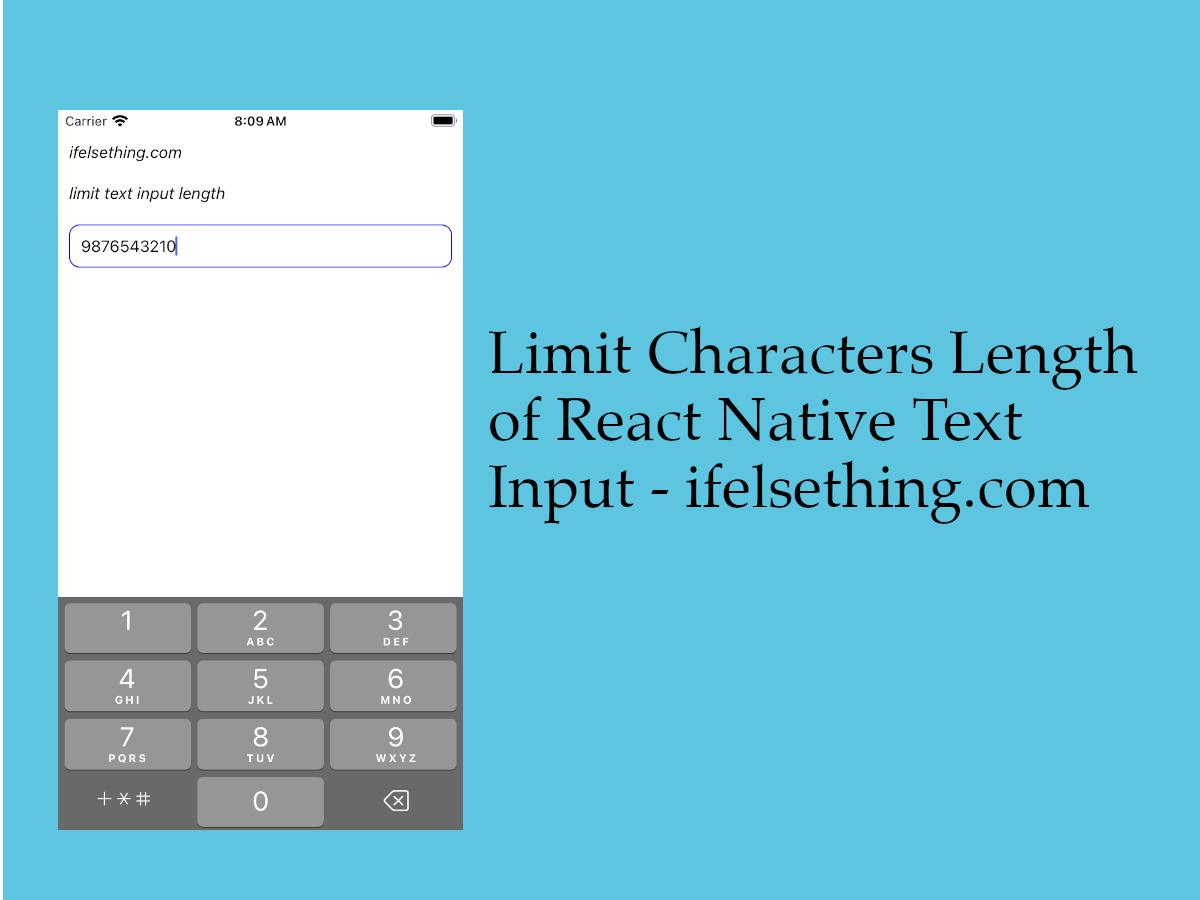
Sometimes we may have a requirement to not allow characters after a certain length in text input. For example, the most common phone number length contains 10 digits. So, if we have a registration page of an app with a phone number input, then we can limit the text input to accept only 10 numbers.
So, to limit characters, we can use maxLength text input prop.
Lets see a simple phone number implementation.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will create a simple text input for the phone number.
Import the TextInput
component in the App.tsx
file with basic required props for a phone number.
//App.tsx
...
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='phone-pad'
placeholder='Enter Phone Number'
placeholderTextColor='gray'
/>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We can see that the text input accepts many numbers even after 10 characters for a phone number. But we want only 10 numbers for a mobile number.
So, add maxLength={10}
to the text input.
...
<TextInput
...
maxLength={10}
/>
...
If we re-run the app and try to enter numbers, we can see that the input accepts only 10 numbers and not beyond that.
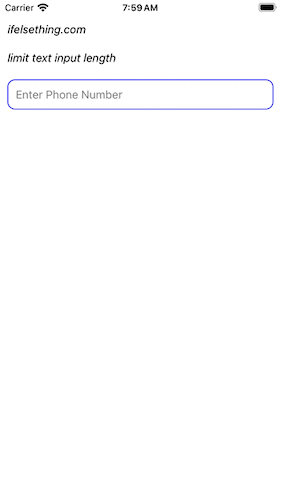
Complete code of our example,
//App.tsx
import {
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
View,
} from "react-native";
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
limit text input length
</Text>
<TextInput
style={styles.input}
keyboardType='phone-pad'
placeholder='Enter Phone Number'
placeholderTextColor='gray'
maxLength={10}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderRadius: 10,
borderWidth: 1,
padding: 10,
fontSize: 15,
color: 'black'
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});