Difference Between onEndEditing and onSubmitEditing in React Native
Published On: 2024-02-29
Posted By: Harish
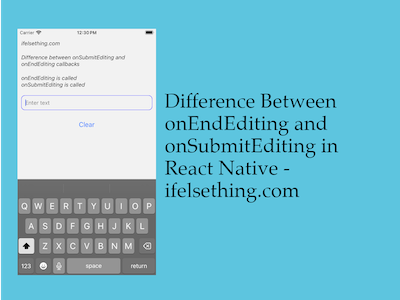
We have two different callbacks to know when the user finished entering text input value. One is the onSubmitEditing
callback and another one is the onEndEditing
callback.
onSubmitEnditing callback is fired when the user presses the keyboard's submit button. onEndEditing callback is fired when the user completely finishes editing, in other words when text input loses its focus because of the submit button or clearing the focus by tapping outside of text input etc.
In this post, we will see that in action.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Import TextInput Component
Import TextInput
component and add basic props to it. Now add onSubmitEditing
callback and console log the event.
//app.tsx
import {
StyleSheet,
TextInput,
} from "react-native";
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
onSubmitEditing={() => {
console.log("onSubmitEditing is called")
}}
autoCorrect={false}
showSoftInputOnFocus={true}
autoFocus={true}
/>
...
Now run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
If we press enter/submit/return button, we will see that onSubmitEditing callback will be called. Now add onEndEditing
callback and console log the event.
Now again run the app and after opening the keyboard, press the submit button again. Now, you will see that both onSubmitEditing and onEndEditing callbacks are fired.
This is happening because on pressing submit button, onSubmitEditing callback is fired normally because it will call on submit button press. And as blurOnSubmit
is true
by default, this removes focus from the text input on submit button press which confirms the completion of entering the value in text input, so onEndEditing
callback is fired.
If we add blurOnSubmit
prop with false
value, keyboard will not remove focus on submit which results in firing only onSubmitEditing callback.
To remove focus, we will use Pressable to close the keyboard on the outside tap. So, import the Pressable
component and Keyboard
module. And dismiss the keyboard on the Pressable onPress
callback by adding Keyboard.dismiss()
method.
import {
...
Keyboard,
Pressable
} from "react-native";
<Pressable
style={styles.container}
onPress={Keyboard.dismiss}
>
<TextInput
...
/>
</Pressable>
If we run the app again, and press submit, we will notice that the keyboard stays open with focus on the text input.
And only onSubmitEditing will be called. onEndEditing callback is only called when tapped outside of the text input.
In our example, we are using a text input reference using the useRef hook to clear the focus using the blur()
method. Even if we press the clear button, the onEndEditing callback will be fired because we are removing focus using the blur()
method.
So, in general understanding, onSubmitEditing callback is called when the keyboard's submit button is pressed. onEndEditing callback is called when text input focus is removed.
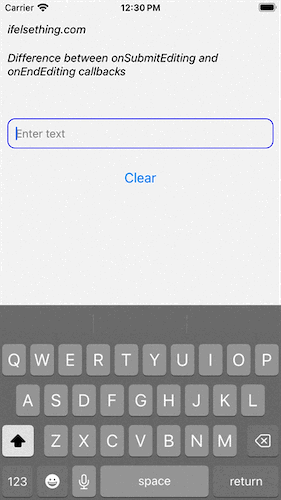
Complete code,
//App.tsx
import { useRef, useState } from "react";
import {
Text,
StyleSheet,
TextInput,
Button,
Keyboard,
Pressable
} from "react-native";
export const App = () => {
const [onEndValue, setOnEndValue] = useState<boolean | undefined>();
const [onSubmitValue, setOnSubmitValue] = useState<boolean | undefined>();
const ref = useRef(null);
return (
<Pressable
style={styles.container}
onPress={Keyboard.dismiss}
>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Difference between onSubmitEditing and onEndEditing callbacks
</Text>
<Text style={styles.text}>
{
onEndValue
&& "onEndEditing is called \n"
}
{
onSubmitValue
&& "onSubmitEditing is called"
}
</Text>
<TextInput
style={styles.input}
ref={ref}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
onSubmitEditing={() => {
console.log("onSubmitEditing is called")
setOnSubmitValue(true);
}}
onEndEditing={() => {
console.log("onEndEditing is called")
setOnEndValue(true);
}}
autoCorrect={false}
showSoftInputOnFocus={true}
autoFocus={true}
/>
<Button
title="Clear"
onPress={() => {
ref.current?.blur();
ref.current?.clear();
setOnSubmitValue(false);
setOnEndValue(false);
}}
/>
</Pressable>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});