Paging in React Native ScrollView
Published On: 2024-06-24
Posted By: Harish
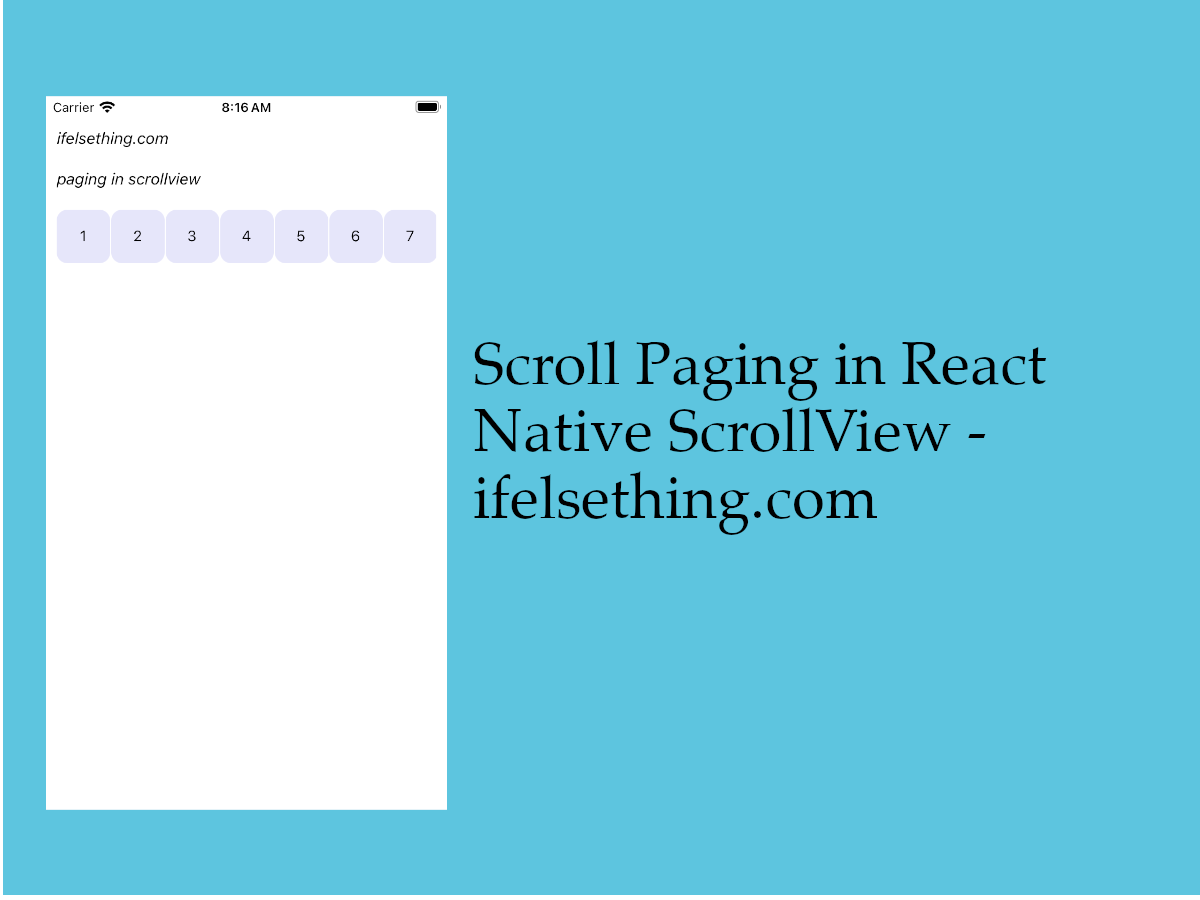
We have seen a way to stop scroll in intervals of specific value in react native scrollview using snapToInterval prop. In that, irrespective of the speed of the scroll, it stops at multiples of specific value.
Paging in scrollview is similar to snapToInterval prop but with one fixed value and that is the device's width or height. With this, content is shown till the width or height of the device and the next scroll shows other followed portions till the width or height of the device.
For paging, we have to use pagingEnabled prop and it accepts boolean values. Default value is false
. If true
, scroll stops in the intervals of the device’s width or height.
Lets see this in action.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ScrollViewRN
Example Implementation
We will create a simple scrollview with number blocks. Think of this as a pagination. For each scroll, the next set of page numbers will be shown.
Import and add ScrollView
with some number blocks. As we are assuming it as a pagination block, we will convert it to a horizontal scrollable scrollview.
//App.tsx
...
import { View, ScrollView, Text } from 'react-native';
...
<ScrollView
horizontal
contentContainerStyle={styles.content_container}
>
{
[...Array(20).keys()]
.map((n: number) => {
return (
<View
key={n}
style={styles.view}
>
<Text>{n + 1}</Text>
</View>
)
})
}
</ScrollView>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see some number blocks similar to pagination. When you scroll, you will see the normal scrolling behavior.
Now add pagingEnabled={true}
to scrollview and try to scroll now.
...
<ScrollView
...
pagingEnabled={true}
>
...
</ScrollView>
...
You will see that the scroll stops at visible intervals of the content. Like in our example, on load, the first 7 blocks are shown and on the next scroll, next 7 blocks are shown starting from number 8th block.
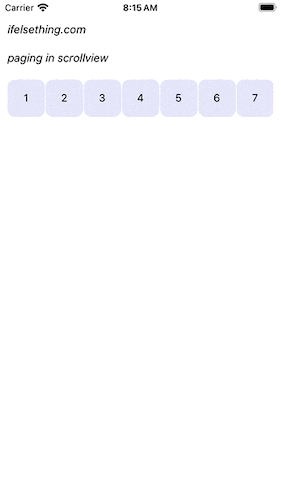
This shows similar behavior as snapToInterval prop which stops at specific intervals. But in this pagingEnabled prop, we have only one interval and that is the device's width or height.
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
ScrollView
} from "react-native";
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
paging in scrollview
</Text>
<ScrollView
pagingEnabled={true}
horizontal
contentContainerStyle={styles.content_container}
>
{
[...Array(20).keys()]
.map((n: number) => {
return (
<View
key={n}
style={styles.view}
>
<Text>{n + 1}</Text>
</View>
)
})
}
</ScrollView>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
gap: 1
},
view: {
width: 50,
height: 50,
borderRadius: 10,
backgroundColor: 'lavender',
justifyContent: 'center',
alignItems: 'center'
}
});