Press Events of React Native TextInput
Published On: 2024-04-11
Posted By: Harish
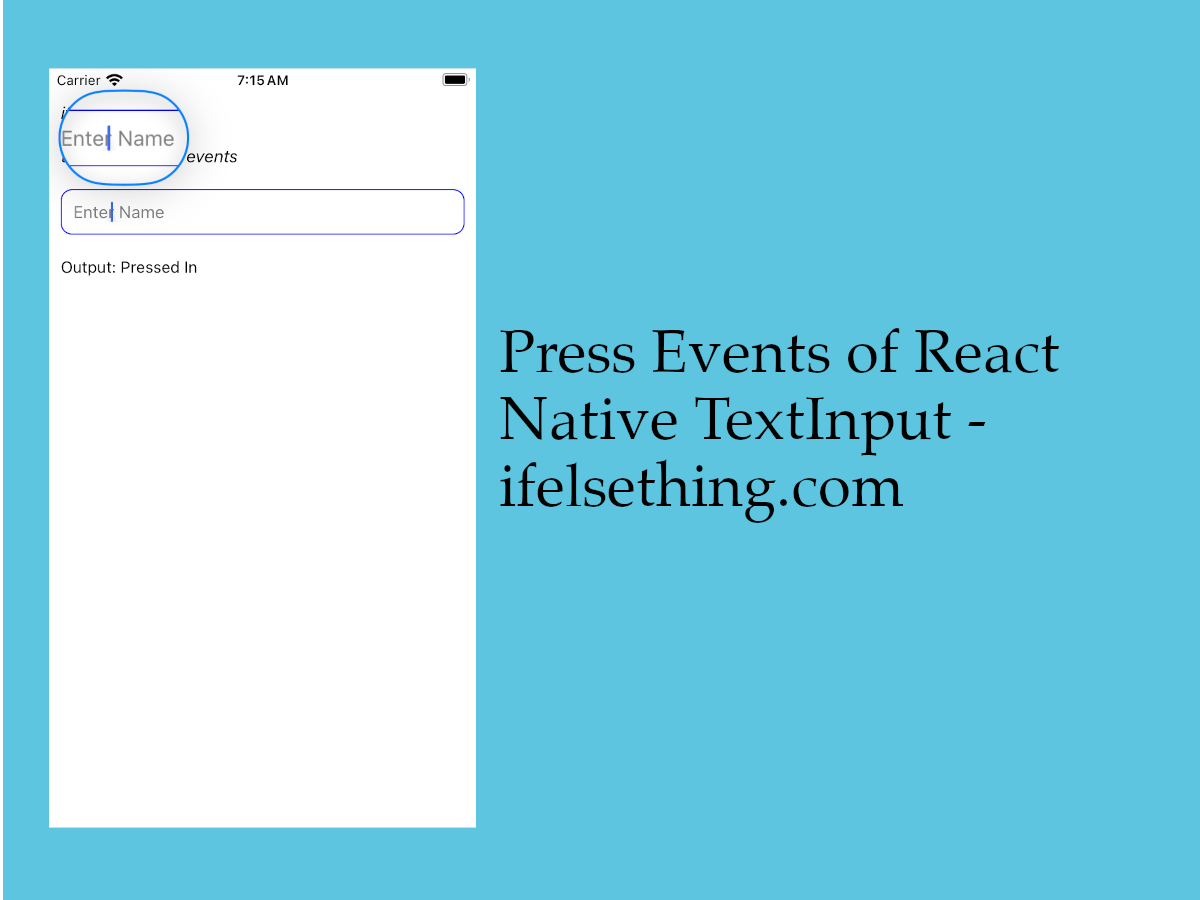
React native textinput component has press events to know when input is tapped or released. For that, we can use onPressIn and onPressOut callbacks.
On tap inside text input, onPressIn callback is triggered and onPressOut is triggered when the tap is released.
Let's see these callbacks in action.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will create a simple text input for the name.
Import the TextInput
component in the App.tsx
file and add basic required props for name.
Now add onPressIn
and onPressOut
callbacks to name input.
//App.tsx
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Name'
placeholderTextColor='gray'
onPressIn={event => {
console.log("Press In: ", event.nativeEvent);
}}
onPressOut={event => {
console.log("Press Out: ", event.nativeEvent);
}}
/>
...
Run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
After rendering, if you tap inside text input, you will see the log of onPressIn
callback. And if you release that tap, onPressOut
callback event is logged.
LOG Press In: {"changedTouches": [[Circular]], "identifier": 1, "locationX": 161, "locationY": 15.5, "pageX": 171, "pageY": 121.5, "target": 389, "timestamp": 3046643.804847, "touches": [[Circular]]}
LOG Press Out: {"changedTouches": [[Circular]], "identifier": 1, "locationX": 161, "locationY": 15.5, "pageX": 171, "pageY": 121.5, "target": 389, "timestamp": 3046741.673141, "touches": []}
Above log output is from iOS device. From the above log, we can see that both events have details about the location of tap and timestamp. In fact, both output the same events but with different timestamps.
So, with these press callbacks of text input, we can know when the user is tapped to enter text or release the tap after tapping.
These are similar to onTouchStart
and onTouchEnd
of touchable library callbacks and can be used to get touch events of text input.
To show output, we will use the useState
hook to store tapping and releasing events.
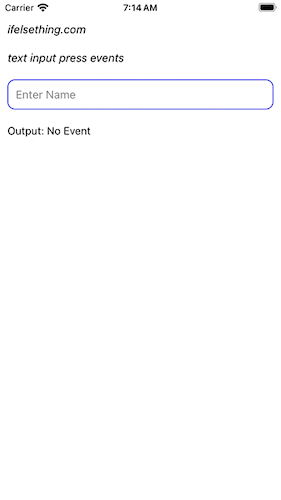
Complete code of our example,
//App.tsx
import { useState } from "react";
import {
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
View
} from "react-native";
export default function App() {
const [eventType, setEventType] = useState<string | undefined>();
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
text input press events
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Name'
placeholderTextColor='gray'
onPressIn={event => {
console.log("Press In: ", event.nativeEvent)
setEventType("Pressed In");
}}
onPressOut={event => {
console.log("Press Out: ", event.nativeEvent);
setEventType("Pressed Out");
}}
/>
<Text>
Output: {eventType ? eventType : 'No Event'}
</Text>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderRadius: 10,
borderWidth: 1,
fontSize: 15,
padding: 10,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});