How to get Keyboard Metrics without Using Events in React Native?
Published On: 2024-02-27
Posted By: Harish
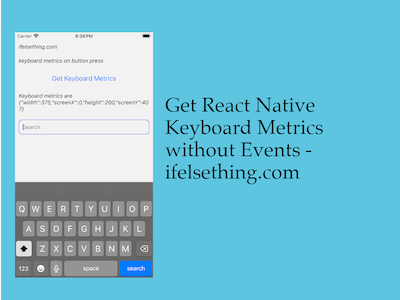
With keyboard events and frame changes events we can get the metrics of the keyboard.
What if we don't want to use keyboard events to get its metrics? In those situations, we can use the Keyboard.metrics()
method to get the keyboard metrics in current state.
In this post we will see an implementation similar to Keyboard.isVisible() post example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init KeyboardRN
Import Keyboard Module
We will import TextInput
, Text
and Button
components with the Keyboard
module. With the use of Keyboard.metrics() method to get metrics of opened keyboard.
Like Keyboard.isVisible()
method, this method is also similar to keyboardDidShow
and keyboardDidHide
events but only returns current metrics, not the complete event.
Returns metrics only after completion of the event. So, in this example we will get the updated metrics with the press of a button.
Below is the complete code of the screen implementation.
//app.tsx
import { useEffect, useState } from "react";
import {
View,
Text,
StyleSheet,
TextInput,
Keyboard,
Button
} from "react-native";
type KeyboardMetrics = {
screenX: number;
screenY: number;
width: number;
height: number;
} | undefined;
export const App = () => {
const [metrics, setMetrics] = useState<KeyboardMetrics>(undefined);
const getKeyboardMetrics = () => {
const metrics: KeyboardMetrics = Keyboard.metrics();
setMetrics(metrics);
};
useEffect(() => {
getKeyboardMetrics();
}, []);
return (
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
keyboard metrics on button press
</Text>
<Button
title="Get Keyboard Metrics"
onPress={getKeyboardMetrics}
/>
<Text style={styles.text}>
Keyboard is {metrics ? JSON.stringify(metrics) : "undefined"}
</Text>
<TextInput
style={styles.input}
keyboardType='default'
returnKeyType='search'
placeholder="Search"
placeholderTextColor='gray'
autoFocus={true}
showSoftInputOnFocus={true}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});
Now run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
If the keyboard is showing, then we will get its current metrics. If it's not open, metrics will be undefined.
Unlike keyboardDidShow
and keyboardDidHide
events, this Keyboard.metrics()
function is available on iOS as well as android operating systems.
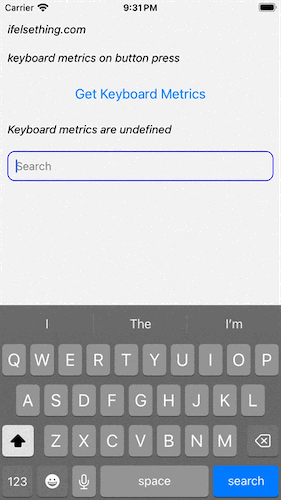