How to Know if React Native Keyboard is Opened or Closed?
Published On: 2024-02-27
Posted By: Harish
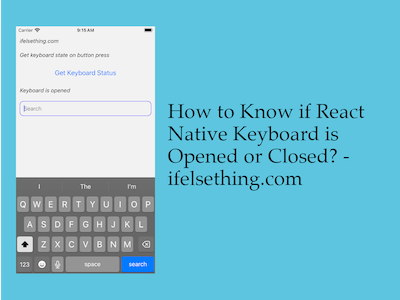
With keyboard events, we can know its different states of showing, hiding or its every frame changes.
These events are completely available for iOS or iPadOS devices, but for android devices, only keyboardDidShow
and keyboardDidHide
events are available with condition. You can know more about it at react native keyboard events documentation.
So, if you are focusing on react native iOS apps and want the keyboard state instantly when they happen, you can use keyboard events. But if you need the keyboard state only when called like by pressing a button, you can use the Keyboard.isVisible()
function.
This function returns true
if the keyboard is showing, false
if it is in a hidden state.
Please note that this function is a simplified version of keyboardDidShow
and keyboardDidHide
events. Keyboard's isVisible function only updates when the keyboard is completely shown or hidden.
Let's check this in action.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init KeyboardRN
Import Keyboard Module
Import required components with the Keyboard
module. We need TextInput
, Text
and Button
components.
We have to use useState
to store the updated keyboard state on button press. And useEffect
to get the keyboard state when the screen renders.
Now, to get the state of the keyboard, we can use Keyboard.isVisible() function.
Below is the complete code of the screen implementation.
//app.tsx
import { useEffect, useState } from "react";
import {
View,
Text,
StyleSheet,
TextInput,
Keyboard,
Button
} from "react-native";
type KeyboardState =
| 'opened'
| 'closed'
| undefined
;
export const App = () => {
const [status, setStatus] = useState<KeyboardState>(undefined);
const getKeyboardStatus = () => {
const isVisible: boolean = Keyboard.isVisible();
const currentState: KeyboardState = isVisible ? 'opened' : 'closed';
setStatus(currentState);
};
useEffect(() => {
getKeyboardStatus();
}, []);
return (
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Events when keyboard frames change
</Text>
<Button
title="Get Keyboard Status"
onPress={getKeyboardStatus}
/>
<Text style={styles.text}>
Keyboard is {status}
</Text>
<TextInput
style={styles.input}
keyboardType='default'
returnKeyType='search'
placeholder="Search"
placeholderTextColor='gray'
autoFocus={true}
showSoftInputOnFocus={true}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});
Now run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We used showSoftInputOnFocus prop on the text input to open the keyboard when screen renders. But the function will return false
as initially its state will be in closed
state. When we press the update button, the value gets updated to true
as the Keyboard.isVisible()
function updates when the event is completely finished.
Unlike keyboardDidShow
and keyboardDidHide
events, this Keyboard.isVisible()
function is available on iOS as well as android operating systems.
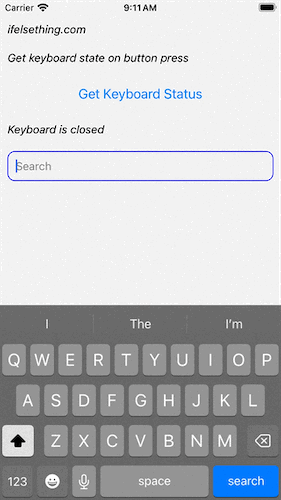