Get Content Scroll Position Inside React Native TextInput
Published On: 2024-04-12
Posted By: Harish
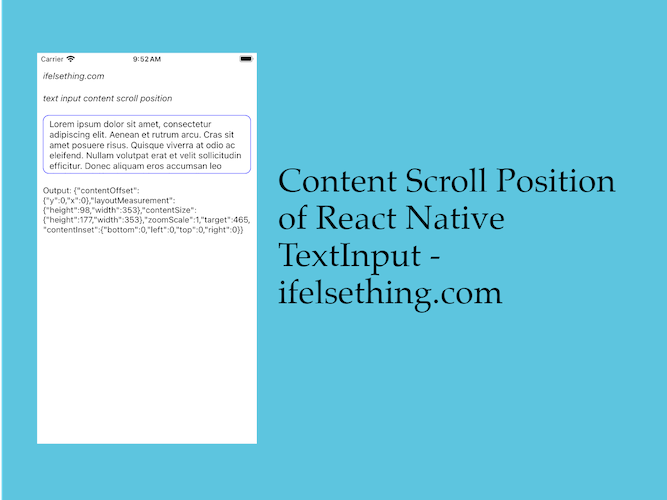
We can know the width and height of the text input content using onContentSizeChange callback. With more content and fixed input height, we will have a scroll inside the input. So, only getting content size gives error placements without scroll positions. So, getting scroll position is also needed if you are using content size.
We can get scroll positions with the help of onScroll callback which returns the content's scroll position whenever we scroll the text inside it.
To understand better, think of a description box with fixed height and more content. Now whenever we scroll that content, this callback is triggered.
We will implement a description box example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will create a simple description text input.
Import the TextInput
component in the App.tsx
file and add basic required props for the description input.
Add onScroll
callback with multiline property. Make input height fixed so that the content becomes scrollable.
//App.tsx
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Description'
placeholderTextColor='gray'
multiline={true}
onScroll={event => {
console.log(event.nativeEvent);
}}
/>
...
Run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
Enter some text inside the description box till the content becomes scrollable.
Now, whenever the content scrolls, onScroll
callback triggers and returns details about content inset and offset positions with other data including input content size.
#iOS Log
LOG {"contentInset": {"bottom": 0, "left": 0, "right": 0, "top": 0}, "contentOffset": {"x": 0, "y": 5}, "contentSize": {"height": 105, "width": 353}, "layoutMeasurement": {"height": 98, "width": 353}, "target": 405, "zoomScale": 1}
So in this way, we can know the position of the text input content using onScroll
callback. For this example, I generated dummy text using Lorum Ipsum generator.
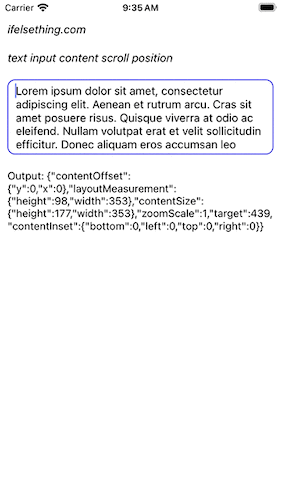
Complete code of our example,
//App.tsx
import React, { useState } from "react";
import {
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
View,
TextInputScrollEventData
} from "react-native";
export default function App() {
const [contentScrollData, setContentScrollData] = useState<TextInputScrollEventData | undefined>();
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
text input content scroll position
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Name'
placeholderTextColor='gray'
multiline={true}
onScroll={event => {
const data = event.nativeEvent;
setContentScrollData(data);
}}
/>
<Text>
Output: {contentScrollData ? JSON.stringify(contentScrollData) : 'Not Available'}
</Text>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderRadius: 10,
borderWidth: 1,
fontSize: 15,
padding: 10,
color: 'black',
height: 100
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});