Scroll Speed in React Native
Published On: 2024-06-09
Posted By: Harish
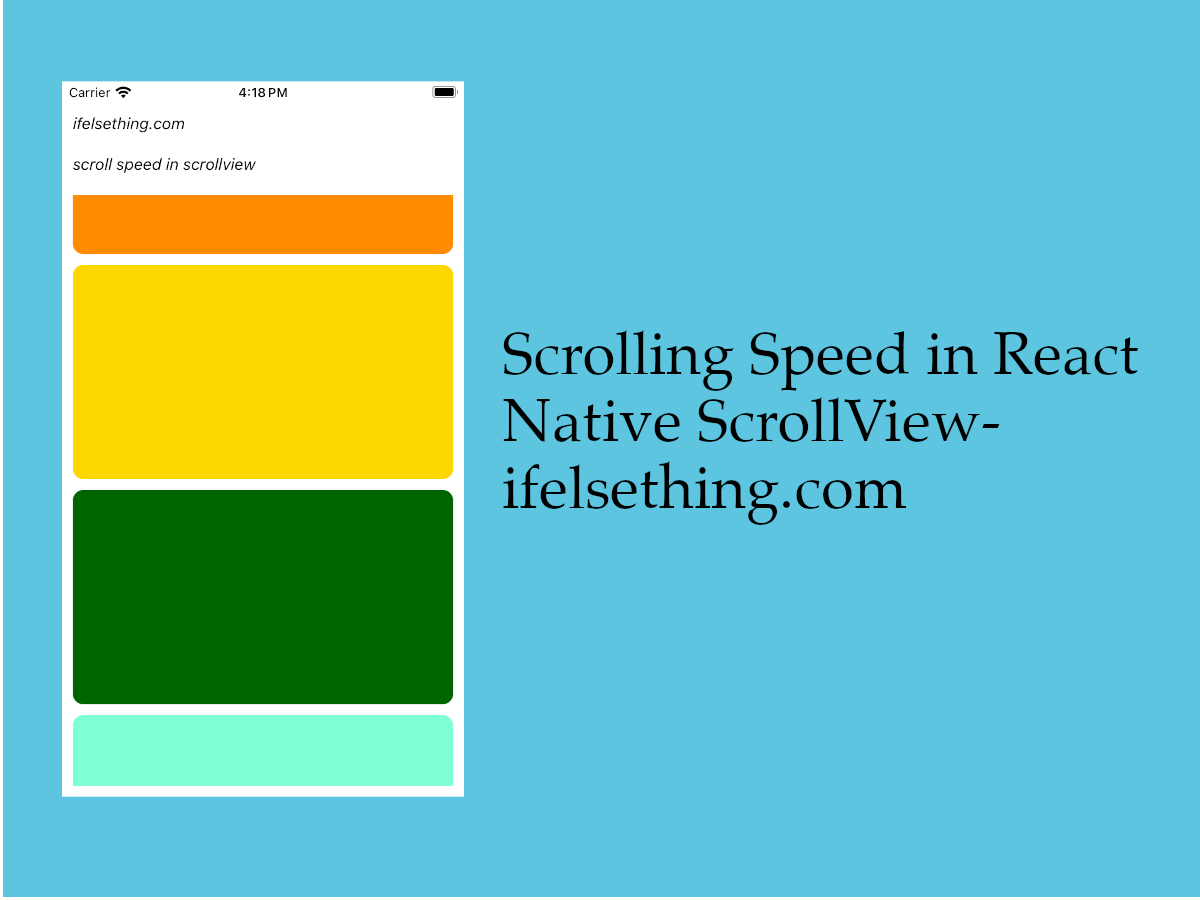
We have seen a way to shift the scroll position to a different position rather than from starting position on load. In this post, we will see a way to control the scroll speed of scrollview content.
We have decelerationRate prop, which takes values to control the deceleration rate. If we give fast
value, the scroll will decelerate fast. For normal behavior, give normal
as value. Default value is normal. It also accepts number value to get the desired scrolling speed.
Lets see its working example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ScrollViewRN
Example Implementation
We will create color blocks scrollview to check its scroll deceleration speed.
Import and add ScrollView
with some color blocks. Refer complete code block below.
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see some scrollable color blocks. If you scroll, you will see a smooth scroll and that is default normal behavior.
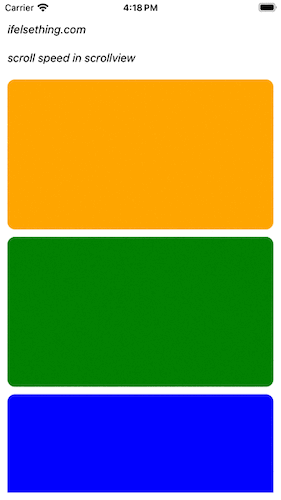
Add decelerationRate="fast"
to scrollview and re-load the app.
...
<ScrollView
...
decelerationRate="fast"
>
</ScrollView>
...
Now, if we scroll, we will see that the scroll speed got reduced and feels sticky. As the prop name suggests, the rate of deceleration is increased. In this way we can control the scrolling speed.
We can also use decimal values 0 to 1 to get the required scrolling speed like decelerationRate={0.8}
.
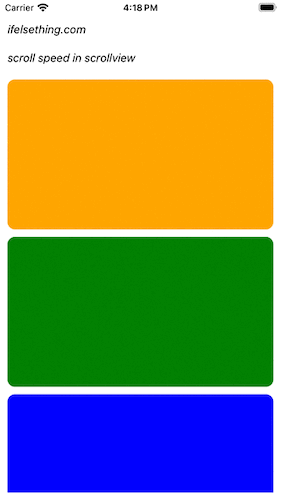
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
ScrollView,
} from "react-native";
const colors = [
'orange',
'green',
'blue',
'maroon',
'violet',
'darkorange',
'gold',
'darkgreen',
'aquamarine',
'cadetblue'
];
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
scroll sensitivity in scrollview
</Text>
<ScrollView
decelerationRate="fast"
contentContainerStyle={styles.scrollview_container}
>
{
colors
.map((color: string) => {
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
/>
)
})
}
</ScrollView>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
scrollview_container: {
gap: 10
},
view: {
flex: 1,
width: '100%',
height: 200,
borderRadius: 10,
alignSelf: 'center'
}
});