Select All Text of React Native Text Input on Focus
Published On: 2024-03-05
Posted By: Harish
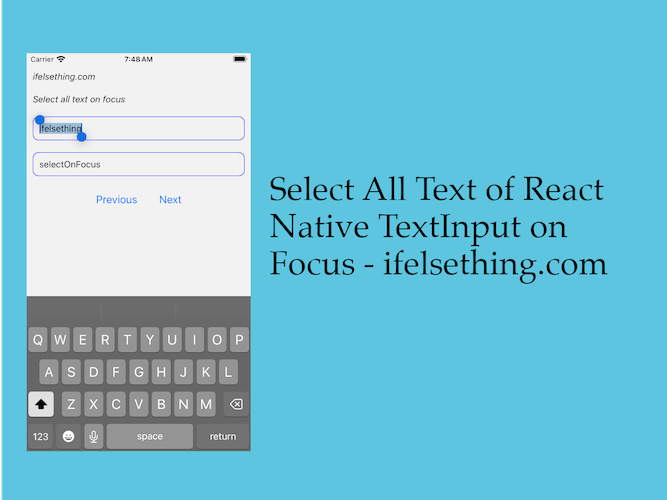
We can programmatically select text between start and end positions of the text using onSelectionChanged callback. So, if we want to select a complete text, we can pass start
as 0
and end
as the length of the text. But for this implementation, we have to use onSelectionChanged and onFocus callbacks. This is a time taking process to implement.
For this scenario, we can use selectTextOnFocus prop without any extra implementations. If we use true
value, complete text will get highlighted on focus.
Lets see in below example,
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
For our example, we will take two text inputs and two buttons. Default text will be assigned to both the text inputs and we will focus on each text inputs on next or previous button press. To get this behavior, we can use useRef to programmatically focus on a text input.
Clear the contents of the App.tsx
file and import TextInput
and Button
components from react-native
. Take two text inputs and add default props to them.
//app.tsx
import {
StyleSheet,
TextInput,
Button
} from "react-native";
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
value="ifelsething"
showSoftInputOnFocus={true}
autoCorrect={false}
autoFocus={true}
/>
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
value="selectOnFocus"
autoCorrect={false}
/>
...
As you can see, we have default values to both the text inputs. Now take two buttons and label them as Previous
and Next
.
Now we will focus on the first text input on the previous button and on the next button press, focus on the second text input.
To achieve this behavior, we will useRef to programmatically focus on text input on a button press. Import useRef
from react and assign as text input references. Now using these references, we will focus on text input using the focus()
method.
import { useRef } from "react";
...
const firstRef = useRef(null);
const secondRef = useRef(null);
...
<TextInput
...
ref={firstRef}
/>
<TextInput
...
ref={secondRef}
/>
...
<Button
title="Previous"
onPress={() => {
if(!firstRef) return;
firstRef.current?.focus();
}}
/>
<Button
title="Next"
onPress={() => {
if(!secondRef) return;
secondRef.current?.focus();
}}
/>
...
Now comes the main part, to select all text inside a text input on focus, we can use selectTextOnFocus
prop which takes boolean values. If true, select all text on focus and vice versa.
Add selectTextOnFocus prop to both inputs.
...
<TextInput
...
ref={firstRef}
selectTextOnFocus={true}
/>
<TextInput
...
ref={secondRef}
selectTextOnFocus={true}
/>
...
Now, if you run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
You will see that on screen render, the first text input gets automatically selected. If we press the Next
button, the second input's text will get selected and highlighted. We can see the behavior in the gif below.
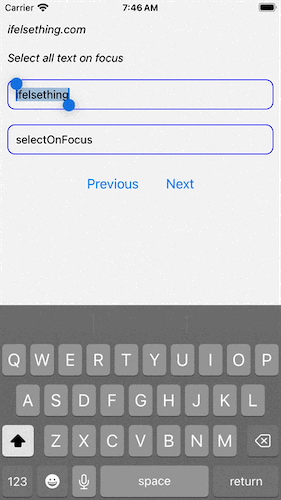
Complete code of our example,
//App.tsx
import { useRef } from "react";
import {
View,
Text,
StyleSheet,
TextInput,
Button
} from "react-native";
export const App = () => {
const firstRef = useRef(null);
const secondRef = useRef(null);
return (
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Select all text on focus
</Text>
<TextInput
ref={firstRef}
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
value="ifelsething"
showSoftInputOnFocus={true}
autoCorrect={false}
selectTextOnFocus={true}
autoFocus={true}
/>
<TextInput
ref={secondRef}
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
value="selectOnFocus"
autoCorrect={false}
selectTextOnFocus={true}
/>
<View style={styles.button_block}>
<Button
title="Previous"
onPress={() => {
if (!firstRef) return;
firstRef.current?.focus();
}}
/>
<Button
title="Next"
onPress={() => {
if (!secondRef) return;
secondRef.current?.focus();
}}
/>
</View>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
button_block: {
flexShrink: 1,
flexDirection: 'row',
gap: 20,
justifyContent: 'center'
}
});