Select Text of React Native Text Input
Published On: 2024-03-03
Posted By: Harish
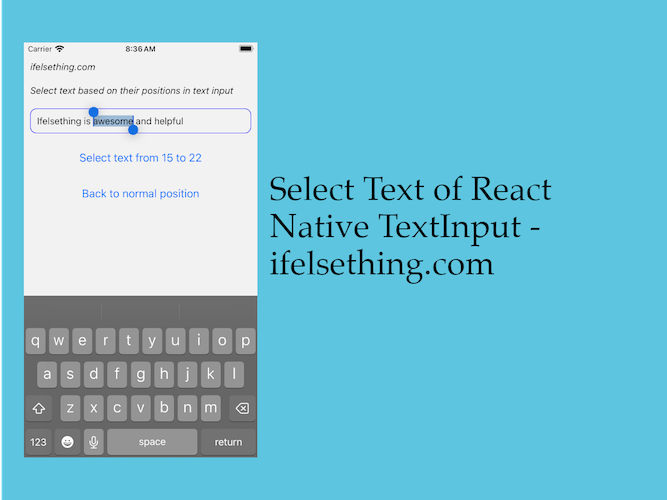
We already saw an implementation of changing cursor position using a selection prop. But what if you want to select text or a set of characters based on their positions?
In these scenarios, we can again use selection prop for text selection. For the cursor position to change, we set the same position to start
and end
keys. And for selection of text, we set different start and end positions to the selection prop.
Lets see the implementation,
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Import TextInput Component
We will be using the same code of text input cursor change post implementation for this post too.
In that example, we changed the position of the cursor on the press of a button. In this post, we will use the same button but we have to change the changePosition
function.
Instead of passing only a single position value to function, we will pass both start and end position of the text which we wanted to select programmatically.
For this example, I calculated the positions based on the text which I'm going to type and they start from 15th position and end at 22nd position.
Now change the PositionType
type with an optional end
, because the end key is optional for selection prop and for our implementation too.
Change changePosition
function to accept start and optional end position. And pass the positions to the function on press to select characters between those numbers.
//app.tsx
...
type PositionType = {
start: number,
end?: number
};
...
const changePosition = (start: number, end?: number) => {
setPosition({
start: start,
end: end
});
}
...
<Button
title="Select characters from 15 to 22"
onPress={() => {
changePosition(15, 22);
}}
/>
...
Now run the app to get similar output as below gif.
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
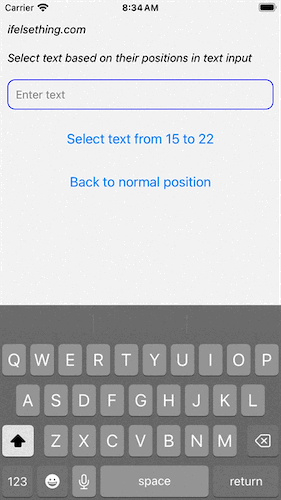
Complete code of our example,
//App.tsx
import { useState } from "react";
import {
View,
Text,
StyleSheet,
TextInput,
Button,
} from "react-native";
type PositionType = {
start: number,
end?: number
}
export const App = () => {
const [position, setPosition] = useState<PositionType>();
const [value, setValue] = useState<string>();
const changePosition = (start: number, end?: number) => {
setPosition({
start: start,
end: end
});
}
return (
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Select text based on their positions in text input
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder="Enter text"
placeholderTextColor='gray'
value={value}
selection={position}
onSelectionChange={(e) => {
const selection = e.nativeEvent.selection;
setPosition(selection)
}}
onChangeText={setValue}
autoFocus={true}
showSoftInputOnFocus={true}
autoCorrect={false}
/>
<Button
title="Select text from 15 to 22"
onPress={() => {
changePosition(15, 22);
}}
/>
<Button
title="Back to normal position"
onPress={() => {
if (!value) return;
changePosition(value.length);
}}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
}
});