Separate FlatList Items with a Component
Published On: 2024-09-08
Posted By: Harish
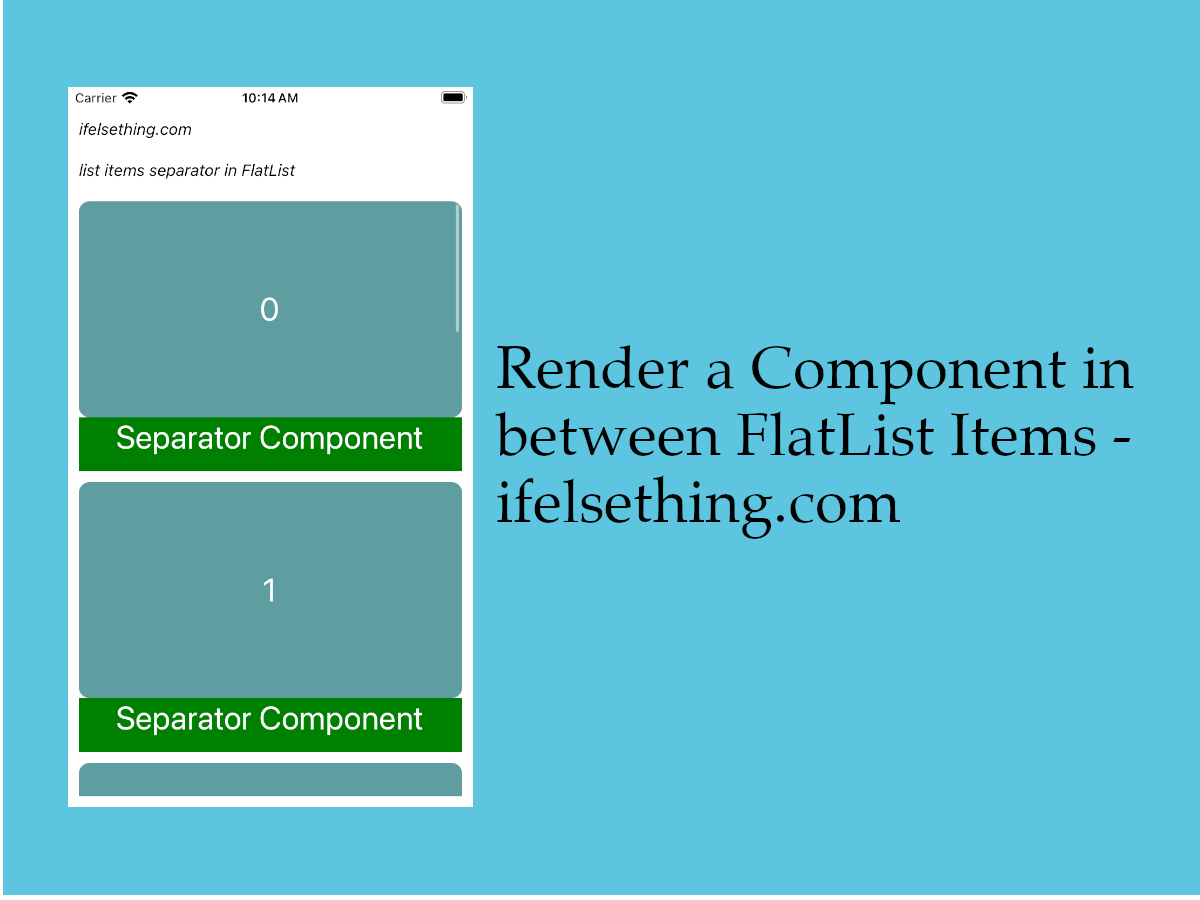
As FlatList inherits properties of VirtualizedList component, FlatList also has ItemSeparatorComponent to render a component in between list items, excluding top and bottom of the FlatList..
Let's see its usage with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init FlatListRN
Example Implementation
We will create a basic FlatList and separate its items using ItemSeparatorComponent prop. We will not use a line divider to separate items as we already did the same in the VirtualizedList ItemSeparator component example.
Import FlatList
from react-native and add to App.tsx
file. You can find more info about a basic FlatList by visiting FlatList with a basic example post.
//App.tsx
...
import { FlatList } from 'react-native';
...
<FlatList
contentContainerStyle={styles.content_container}
data={data}
keyExtractor={item => item + ""}
renderItem={({ item }) => {
return (
<Item item={item} />
)
}}
/>
...
You can find complete code at the bottom of this post.
If we run the app now,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
You will see a simple blocks list.
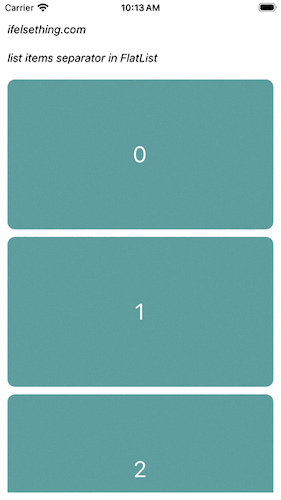
Now we will add a single component, which renders in between list items.
Add ItemSeparatorComponent
functional prop to FlatList
.
...
<FlatList
...
ItemSeparatorComponent={() => <ItemSeparator />}
/>
...
<ItemSeparator>
is an example component view which renders in between list items. Check the code at the bottom of this post.
If you run the app,
You will see a list with a common view in between list items.
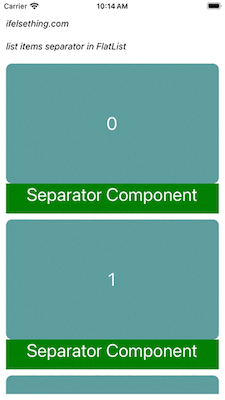
We can achieve this same view by using this <ItemSeparator>
component directly in renderItem
callback. But using there, this component renders every time renderItem view renders, which may show performance issues for large lists. So, using ItemSeparatorComponent
is the best choice to make for showing a component in between FlatList items.
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
FlatList
} from "react-native";
export default function App () {
const data = [...Array(10).keys()];
const Item = ({ item }: { item: number }) => {
return (
<View
key={item}
style={styles.item_view}
>
<Text style={styles.item_text}>{item}</Text>
</View>
)
};
const ItemSeparator = () => {
return (
<View
style={[
{
height: 50,
backgroundColor: 'green'
}
]}
>
<Text style={styles.item_text}>Separator Component</Text>
</View>
)
};
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
list items separator in FlatList
</Text>
<FlatList
contentContainerStyle={styles.content_container}
data={data}
keyExtractor={item => item + ""}
ItemSeparatorComponent={() => <ItemSeparator />}
renderItem={({ item }) => {
return (
<Item item={item} />
)
}}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
gap: 10
},
item_view: {
width: '100%',
height: 200,
borderRadius: 10,
backgroundColor: 'cadetblue',
alignItems: 'center',
justifyContent: 'center'
},
item_text: {
color: 'white',
fontSize: 30,
alignSelf: 'center'
}
});