Show or Hide Scrollbar of React Native ScrollView
Published On: 2024-06-25
Posted By: Harish
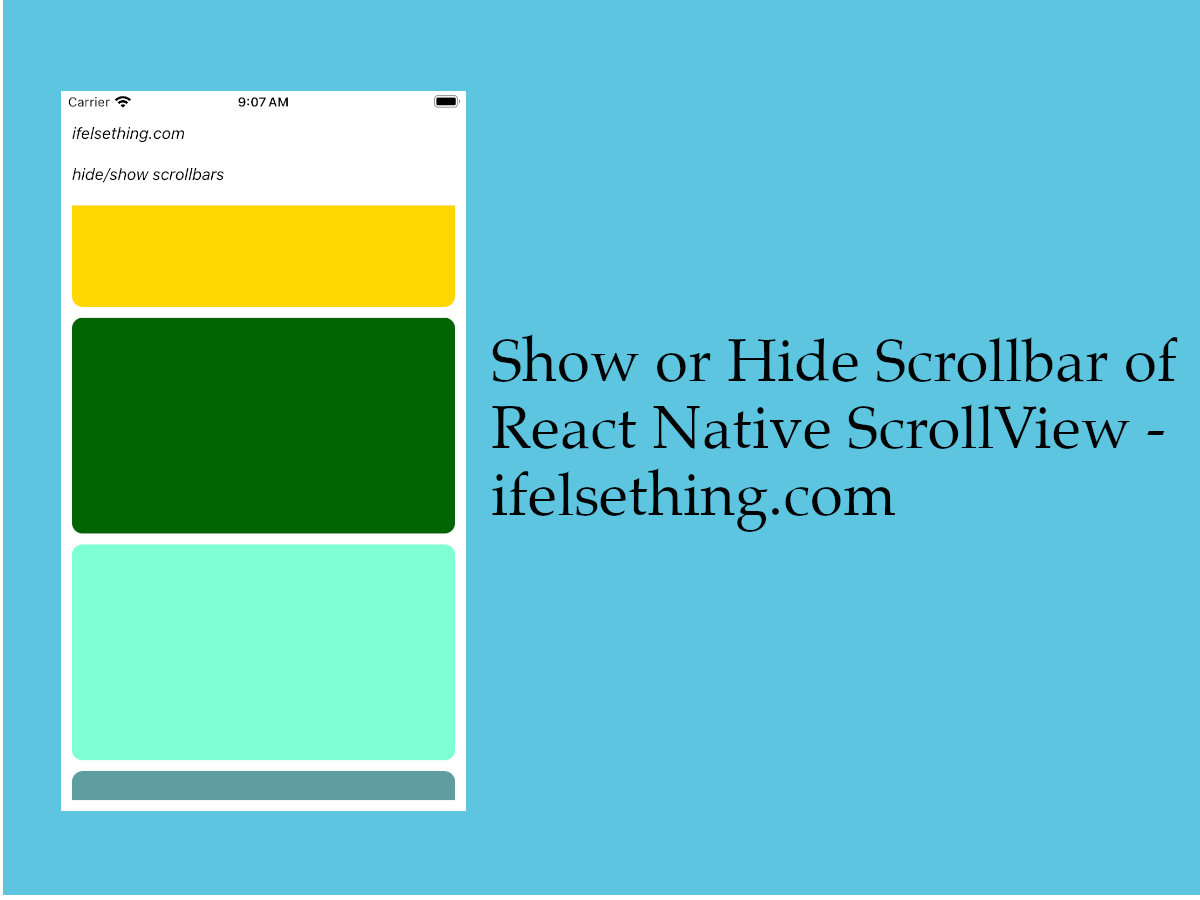
It's a default behavior to show a scrollbar for a scrollable list. With the help of a scrollbar, we can see how much we scrolled from the top of the list.
But in some instances, we may not need a scrollbar while scrolling. In those situations, we can use showsHorizontalScrollIndicator or showsVerticalScrollIndicator props based on the list orientation.
These props accept boolean values and default value is true
. If false
, the scrollbar will hide while scrolling.
Let's see what these props will do with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ScrollViewRN
Example Implementation
We will create a simple vertical scrollview with color blocks as content and show/hide scrollbar while scrolling.
Import and add ScrollView
with few scrollable color blocks.
//App.tsx
...
import { View, ScrollView } from 'react-native';
...
<ScrollView
contentContainerStyle={styles.content_container}
>
{
colors
.map((color: string) => {
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
>
</View>
)
})
}
</ScrollView>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see a few scrollable color blocks. If you scroll, you will see a light scrollbar at the right side of the list.
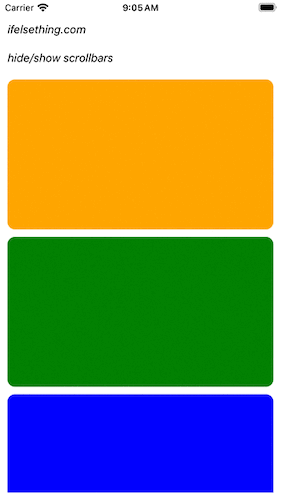
Now add showsVerticalScrollIndicator={false}
to scrollview and try to scroll now.
...
<ScrollView
...
showsVerticalScrollIndicator={false}
>
...
</ScrollView>
...
You will find that the scrollbar is hidden.
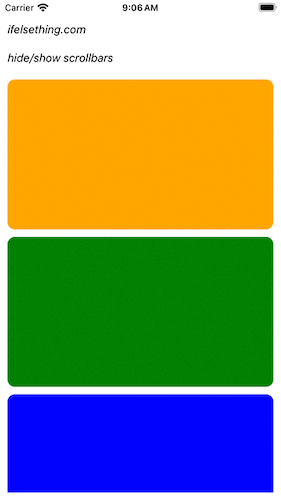
If the list is oriented horizontally, use showsHorizontalScrollIndicator={false}
for the scrollview.
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
ScrollView,
} from "react-native";
const colors = [
'orange',
'green',
'blue',
'maroon',
'violet',
'darkorange',
'gold',
'darkgreen',
'aquamarine',
'cadetblue'
];
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
hide/show scrollbars
</Text>
<ScrollView
//horizontal (for horizontal list)
//showsHorizontalScrollIndicator={false} (for horizontal list)
showsVerticalScrollIndicator={false}
contentContainerStyle={styles.content_container}
>
{
colors
.map((color: string) => {
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
>
</View>
)
})
}
</ScrollView>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
gap: 10
},
view: {
width: '100%',
height: 200,
borderRadius: 10
}
});