Show No Data on Empty FlatList
Published On: 2024-09-08
Posted By: Harish
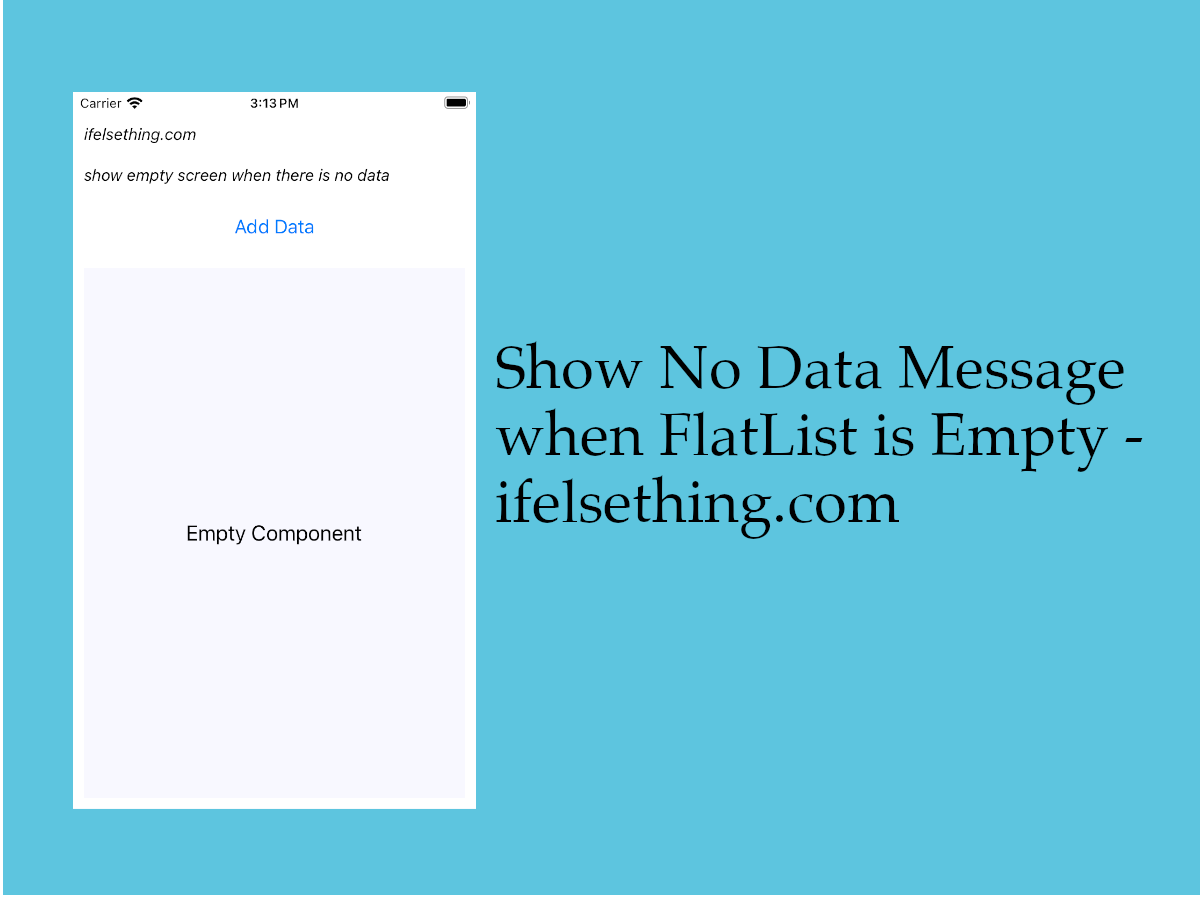
In general, we can write a condition to show a view with a ‘No Data Found’ message on empty list or show FlatList when the list has data. But this approach needs extra code. Instead of that, we can use the ListEmptyComponent functional prop to let the users know about the empty list.
We already saw this functional prop in VirtualizedList with an empty data component post. As FlatList inherits VirtualizedList props, ListEmptyComponent can be used for FlatList too.
Let's see its usage with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init FlatListRN
Example Implementation
We will create a simple list and make that list empty on press of a button to show an empty data component.
Import FlatList
from react-native and add to App.tsx
file. You can find more info about a basic FlatList by visiting FlatList with a basic example post.
//App.tsx
...
import { FlatList } from 'react-native';
...
<FlatList
contentContainerStyle={styles.content_container}
data={data}
keyExtractor={item => item + ""}
renderItem={({ item }) => {
return (
<Item item={item} />
)
}}
/>
...
If we run the app now,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see a simple blocks list.
If we make this list empty, FlatList will show nothing.
Now add the ListEmptyComponent
prop to FlatList
.
...
<FlatList
...
ListEmptyComponent={() => <EmptyComponent />}
/>
...
Instead of an empty screen, <EmptyComponent>
component's data will be shown on empty list.
Reload the app to see the contents of the empty component.
We will use the useState
hook and Button
component to clear the list or add the data to the list. Refer below for complete code.
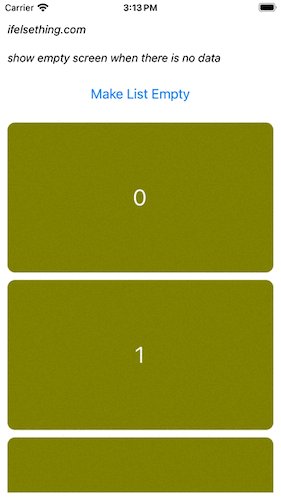
Complete code of our example,
//App.tsx
import React, { useState } from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
FlatList,
Button
} from "react-native";
export function App() {
const [ data, setData ] = useState<Array<number>>([...Array(10).keys()]);
const Item = ({ item }: { item: number }) => {
return (
<View
key={item}
style={styles.item_view}
>
<Text style={styles.item_text}>{item}</Text>
</View>
)
};
const EmptyComponent = () => {
return (
<View style={styles.empty_component}>
<Text style={styles.empty_component_text}>
Empty Component
</Text>
</View>
)
};
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
show empty screen when there is no data
</Text>
<View>
{
data.length != 0
&& <Button
title='Make List Empty'
onPress={() => setData([])}
/>
}
{
data.length == 0
&& <Button
title='Add Data'
onPress={() => setData([...Array(10).keys()])}
/>
}
</View>
<FlatList
contentContainerStyle={styles.content_container}
data={data}
keyExtractor={item => item + ""}
ListEmptyComponent={() => <EmptyComponent />}
renderItem={({ item }) => {
return (
<Item item={item} />
)
}}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
content_container: {
flexGrow: 1,
gap: 10
},
item_view: {
width: '100%',
height: 200,
borderRadius: 10,
backgroundColor: 'olive',
alignItems: 'center',
justifyContent: 'center'
},
item_text: {
color: 'white',
fontSize: 30,
},
empty_component: {
flex: 1,
backgroundColor: 'ghostwhite',
justifyContent: 'center',
alignItems: 'center'
},
empty_component_text: {
fontSize: 20,
color: 'black',
},
});