Show Remote URL Image in React Native
Published On: 2024-05-07
Posted By: Harish
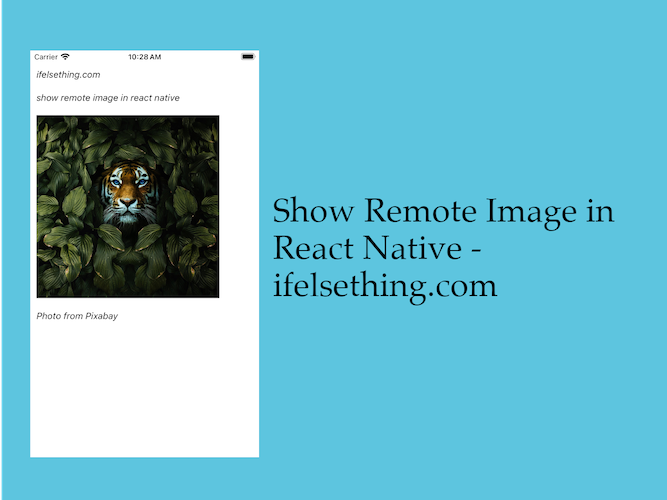
We saw how to show a local asset image stored on a device in react native. But what if we want to show a dynamic image having an URL, like an image hosted on cloud.
For that, we can use source prop or src prop of the Image component.
Lets see the process of displaying a remote image with an example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ImageRN
Example Implementation
We will display a simple remote image using its url link.
Import and add Image
component with source
prop. This prop can be used to show local images or also can be used to show remote images, by passing the link as the value to uri
key.
Check below code for better understanding.
//App.tsx
...
import { Image } from 'react-native';
...
<Image
source={{
width: 300,
height: 300,
uri: 'https://cdn.pixabay.com/photo/2023/12/07/19/45/tiger-8436227_1280.jpg'
}}
resizeMode="cover"
/>
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We can see that the remote image is loaded with fixed width and height.
We can specify the remote image's size using source prop's width and height keys, with normal style or using Image component’s width and height props.
We can also use src
of the Image component to show the remote image, which accepts only the remote image's link.
...
<Image
style={{
width: 300,
height: 300
}}
src='https://cdn.pixabay.com/photo/2023/12/07/19/45/tiger-8436227_1280.jpg'
resizeMode="cover"
/>
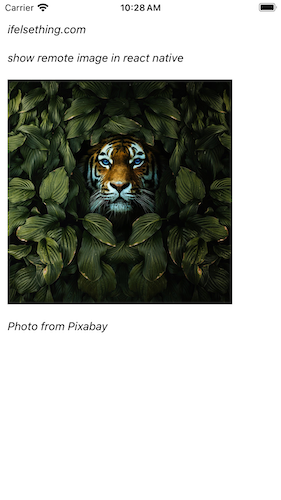
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
Image
} from "react-native";
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
show remote image in react native
</Text>
<Image
source={{
width: 300,
height: 300,
uri: 'https://cdn.pixabay.com/photo/2023/12/07/19/45/tiger-8436227_1280.jpg',
}}
resizeMode="cover"
// style={{
// width: 300,
// height: 300
// }}
// src='https://cdn.pixabay.com/photo/2023/12/07/19/45/tiger-8436227_1280.jpg'
/>
<Text style={styles.text}>
Photo from Pixabay
</Text>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
}
});