Styling ScrollView Children React Native
Published On: 2024-05-31
Posted By: Harish
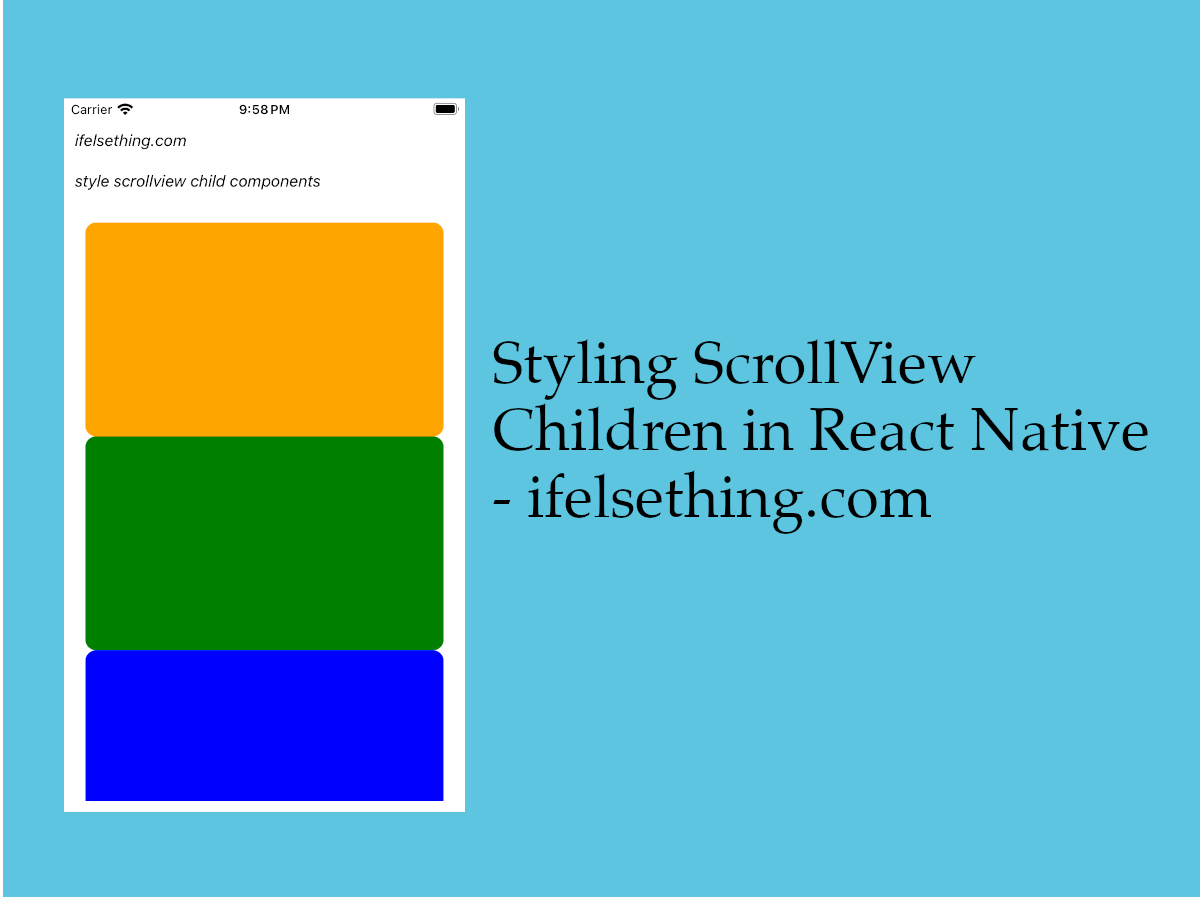
Generally we style components with the style prop. But for the ScrollView component, we have both style prop as well as contentContainerStyle prop.
Style prop is to style ScrollView as a view container but contentContainerStyle prop is to style its child views.
Lets see this by styling scrollview.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ScrollViewRN
Example Implementation
We will create a scrollview screen with color blocks, apply styles to scrollview and its content using above props.
Import and add ScrollView from react-native with random color block views to get content for scrolling.
//App.tsx
...
import { View, ScrollView } from 'react-native';
...
<ScrollView>
{
colors
.map((color: string) => {
return (
<View
key={color}
style={[
styles.view,
{
backgroundColor: color
}
]}
>
</View>
)
})
}
</ScrollView>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We can see color blocks without any styling.
Now we want some padding for the scrollview and some gaps between blocks.
So, add style prop with padding and a gap.
...
<ScrollView
style={{ padding: 10, gap: 10 }}
>
...
</ScrollView>
...
If we reload the app, we can see that padding will be applied to the container but the gap will not be applied to the color blocks.
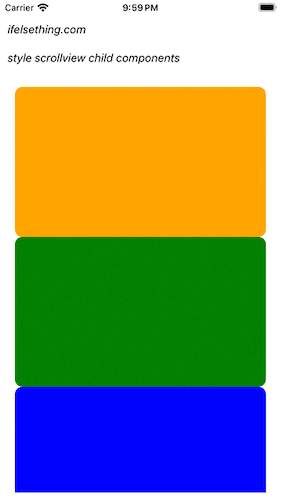
This is because style property only applies to scrollview as a whole container, not to its children.
For better understanding, think scrollview has a view as a wrapper container to its children. Now whatever we want to apply to its children applies to view wrapper not to the children inside it.
So, to apply styles to scrollview children, we have to use contentContainerStyle
prop.
Now add contentContainerStyle prop and add a gap to it.
...
<ScrollView
style={{ padding: 10 }}
contentContainerStyle={{ gap: 10 }}
>
...
</ScrollView>
...
If we re-run the app, we can see the gap between blocks.
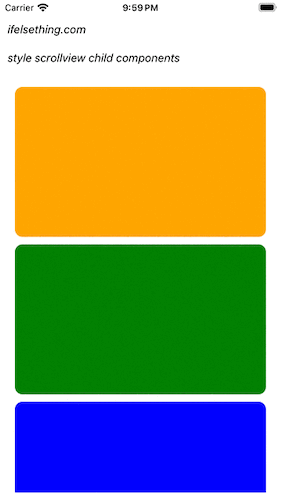
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
ScrollView,
} from "react-native";
const colors = [
'orange',
'green',
'blue',
'maroon',
'violet',
'darkorange',
'gold',
'darkgreen',
'aquamarine',
'cadetblue'
];
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
style scrollview child components
</Text>
<ScrollView
style={{ padding: 10 }}
contentContainerStyle={{ gap: 10 }}
>
{
colors
.map((color: string) => {
return (
<View
key={string}
style={[
styles.view,
{
backgroundColor: color
}
]}
/>
)
})
}
</ScrollView>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
view: {
flex: 1,
width: '100%',
height: 200,
borderRadius: 10,
alignSelf: 'center'
}
});