How to Show Transformed Text in React Native TextInput Field?
Published On: 2024-02-16
Posted By: Harish
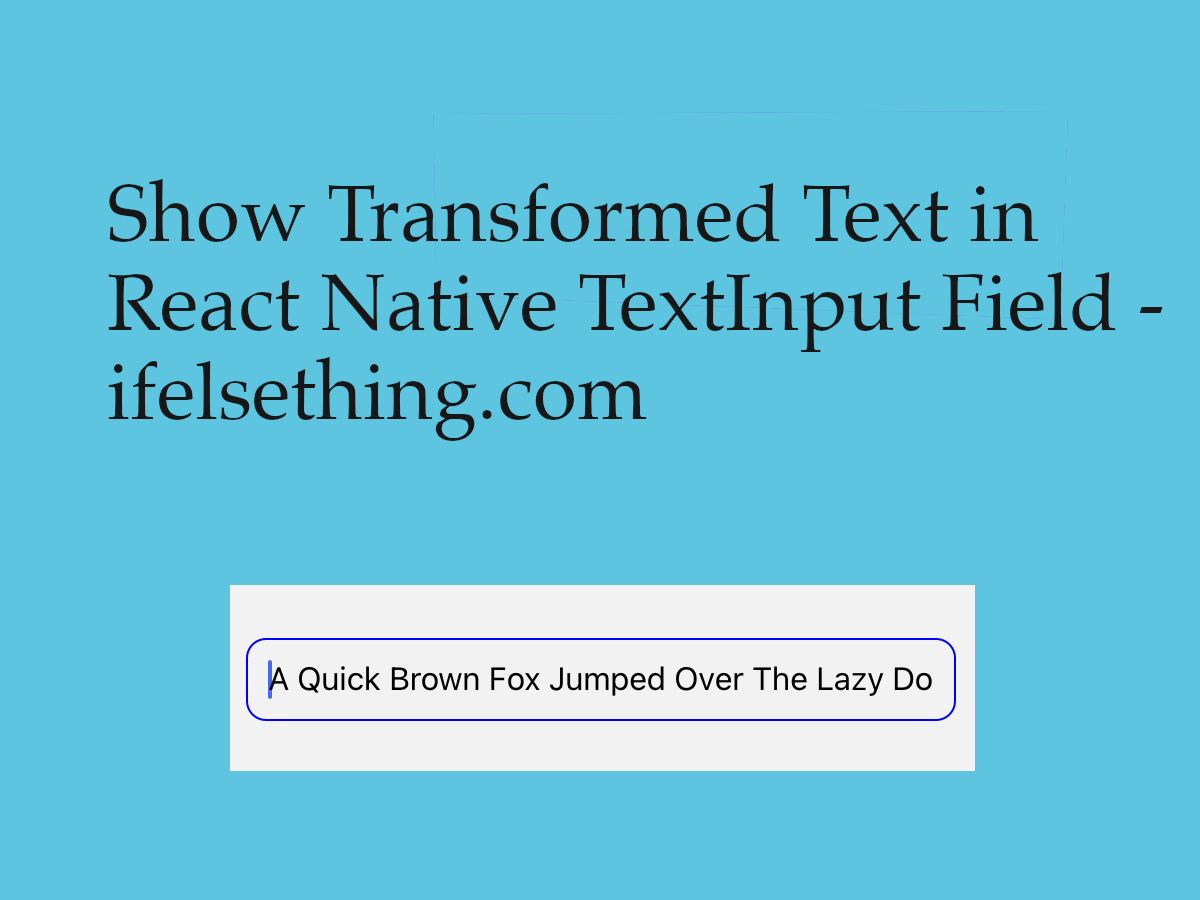
To transform text, we can use textTransform
style for text components, but for <TextInput>
field, they will not work.
To transform text in TextInput, we can use a programmatic approach or simply autoCapitalize
prop.
In programmatic approach, you have to take a state
by importing useState
, get the TextInput value through onChange
event, transform the value to desired styling, like value.toUpperCase()
and finally set the value to same TextInput field using value
prop.
Another approach is to simply add a new prop called autoCapitalize
to react native <TextInput>
field. Let's check them.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Add a TextInput Field and autoCapitalize Prop
Let's add a <TextInput>
field and add autoCapitalize
prop to TextInput.
Add autoCapitalize="none"
, if you don't want any default text transformation.
//app.tsx
import {TextInput} from 'react';
...
<TextInput
style={styles.input}
autoCapitalize="none"
/>
Add autoCapitalize="sentences"
, if you want the starting letter of the sentence to be in a capital letter and rest in small letters i.e, capitalize.
<TextInput
style={styles.input}
autoCapitalize="sentences"
/>
Add autoCapitalize="words"
, if you want the starting letter of every word to be in a capital letter.
<TextInput
style={styles.input}
autoCapitalize="words"
/>
Add autoCapitalize="characters"
, for default text transformation into uppercase.
<TextInput
style={styles.input}
autoCapitalize="characters"
/>
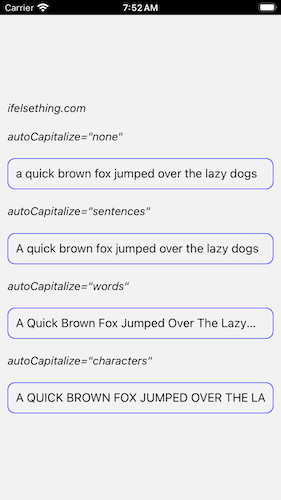