Textarea for React Native
Published On: 2024-04-09
Posted By: Harish
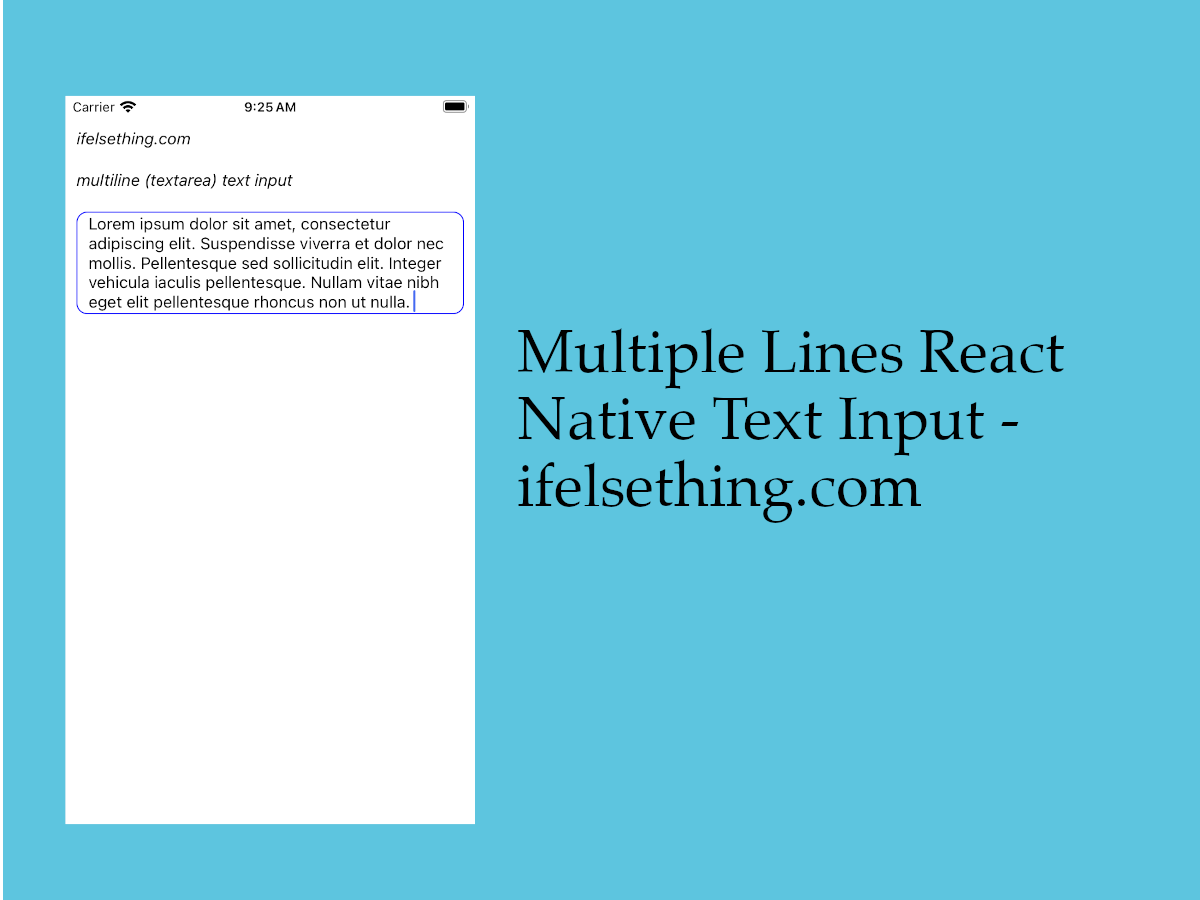
React native has a general text input with single line text by default. But what if you need a bigger text input with multiple lines of text, like a description field.
To get multiple lines of text, we can use a multiLine prop to show multi line text.
We will see a simple multi line implementation.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will create a simple text input for description.
Import the TextInput
component in the App.tsx
file and add basic required props for description.
//App.tsx
...
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Description'
placeholderTextColor='gray'
/>
...
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see a general single line text input. Now to get multiple line texts, we can use multiline prop. This prop converts text input similar to textarea for react native.
So, add multiline={true}
to the text input.
...
<TextInput
...
multiline={true}
/>
...
input: {
...
paddingTop: 10,
paddingBottom: 10,
paddingHorizontal: 10,
maxHeight: 50
}
After reload, we can see a single text input but if we enter text, it wraps text into multiple lines.
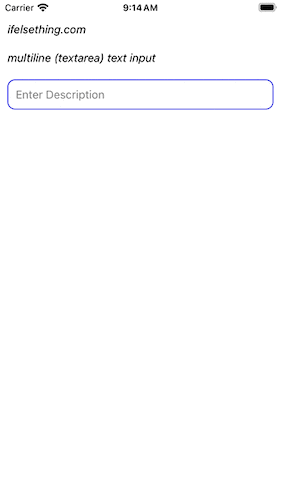
Complete code of our example,
//App.tsx
import {
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
View,
} from "react-native";
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
multiline (textarea) text input
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Description'
multiline={true}
placeholderTextColor='gray'
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderRadius: 10,
borderWidth: 1,
paddingTop: 10,
paddingBottom: 10,
paddingHorizontal: 10,
fontSize: 15,
color: 'black',
maxHeight: 50
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});