How to Use Icons in React Native
Published On: 2024-03-06
Posted By: Harish
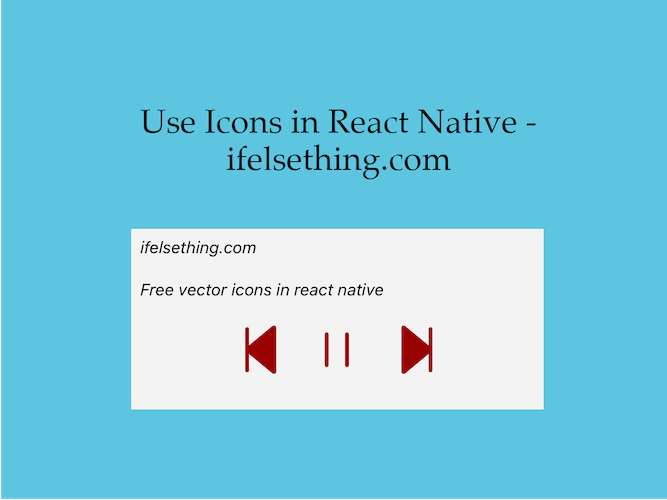
Almost every app needs an icon to go well with the design. In web frameworks or web libraries, we can easily use an SVG icon. But for mobile devices, using a SVG or vector icon requires external npm packages.
We can use images as icons but they will pixelate with higher screen resolution devices. So, using an SVG icon or vector icon is much more beneficial in the long run.
To use an icon in a react native project, we can use a package called react-native-vector-icons
which provides free vector icons to use in iOS and android apps. Supports other operating systems too. You can find out more at react-native-vector-icons github repo.
If you want to use custom SVG, you can go with react-native-vector-images package which converts SVG to vector image which has more control over this icon package. Even further, using react-native-svg library, we can conditionally apply width, height, fill and other properties by creating the SVG component and passing the values as normal props.
In this post I will walk through the process of installing this library on iOS and android devices.
Let's start.
Note: If you are using an existing project, I recommend you to backup the code or simply make a copy of the project before installing.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init VectorIcons
Package Installation
After creating a new project, clear the contents of the App.tsx
file and return only SafeAreaView
for now.
Now install react-native-vector-icons npm library.
#npm
npm i react-native-vector-icons --save
#yarn
yarn add react-native-vector-icons
Here our project is in typescript, so we need to install type declarations as dev dependencies for the package. No need for javascript projects.
#npm
npm i @types/react-native-vector-icons --save-dev
#yarn
yarn add @types/react-native-vector-icons -D
Create a react-native.config.js file
After installing, don't run the project. Create a file named react-native.config.js
at the root of the project (in the folder where the package.json
file is placed).
And add below code to it.
//react-native.config.js
module.exports = {
dependencies: {
'react-native-vector-icons': {
platforms: {
ios: null,
},
},
},
};
This file’s code is to avoid auto linking(react-native above 0.60 version) in iOS devices. This is to avoid addition of files required to use on projects. If we need only one design, we can only link that one design vector file if we don’t auto link in iOS. More information can be found at github.
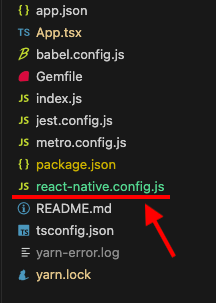
iOS setup
Setup in iOS takes a lot of time. So let's install it first for iOS devices.
I'm using VSCode IDE. You can use any other IDE if you want. I walk through based on vscode here.
Step 1: Pods installation
Open VSCode terminal, cd to ios
folder and install pods. To make sure you have the latest cocoapods, install cocoapods first.
#first
cd ios
#second
sudo gem install cocoapods
#third
pod install
Wait for installation to complete at each command. This pod install
creates a new .xcworkspace
file in the project's ios folder. Open ios folder and double click that to open the project in the XCode application.
Step 2: Copy vector fonts to iOS target in XCode
After a successful installation, open your project's node_modules
and search for react-native-vector-icons
projects in the node_modules folder (node_modules/react-native-vector-icons) and find a folder named fonts
.
Copy this fonts folder somewhere else like on desktop and drag that fonts
folder into XCode's project target. If you want to use icons of only specific design, find the design inside the fonts folder and copy only that font file into the XCode project target.
I'm using only a single font design AntDesign
so, I copied only one font in this project.
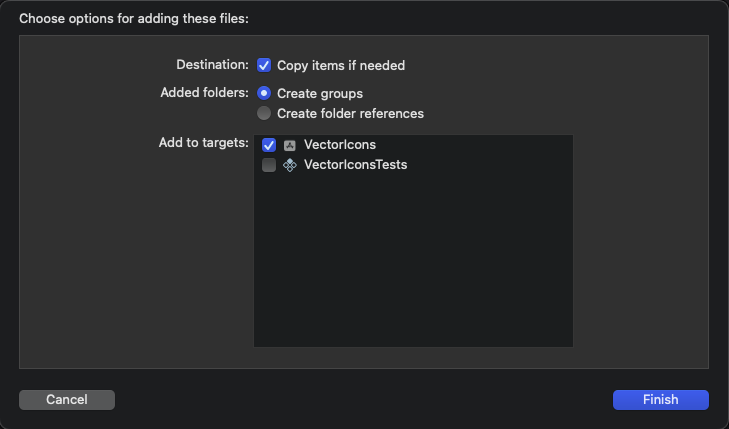
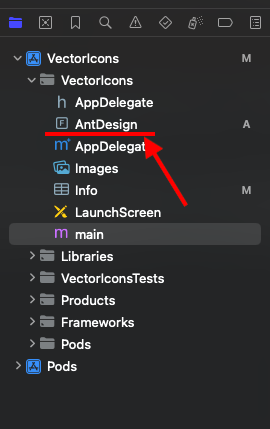
Here I directly copied the single font file to the project. It is advisable to keep font(s) in a folder.
Step 3: Include copied font name in info.plist
After copying, go to the project's info.plist
and create a new key named Fonts provided by application
under Information Property List
. You can create it by clicking the +
sign of the Information Property List key.
And mention the added font name(s) in that key by creating a new item for each font. We copied only one font, so enter that font name with the extension at item 0
. You can find the available list here.
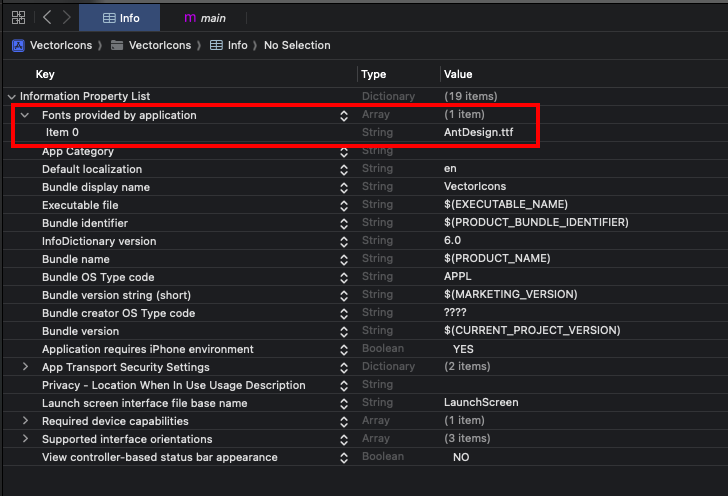
After that, install pods again to complete setup for iOS devices. Now we will move on to android devices
# cd to ios folder
pod install
Build Issues:
If you face any build issues, the first thing to do is to Clean Build Folder
by pressing Shift + Command + K
or by clicking Product > Clean Build Folder
.
If the problem persists, close the XCode application and delete the project's derived data folder by visiting /Users/computer-name-here/Library/Developer/Xcode/DerivedData/
(replace computer-name-here
with your computer name). You can directly visit that folder by pressing Shift + Command + G
and pasting the path.
This applies to basic build errors. If an error is repeated even after cleaning the build, you have to manually know the reason and rectify.
Android
Setup process for android devices is very easy.
Step 1: Add a line to the app’s gradle file
Open project's android folder in Android Studio. If you don't have an android studio, you can download it from here.
After opening, wait until a successful build and then open the build.gradle
file inside android/app/
folder. Not the one which is outside of the app
folder.
And paste the below line at the bottom of the build.gradle
file.
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
And that's it. Build the project and after successful build, you are ready to use it on android devices.
We can also include only specific fonts to build. You can find more info here.
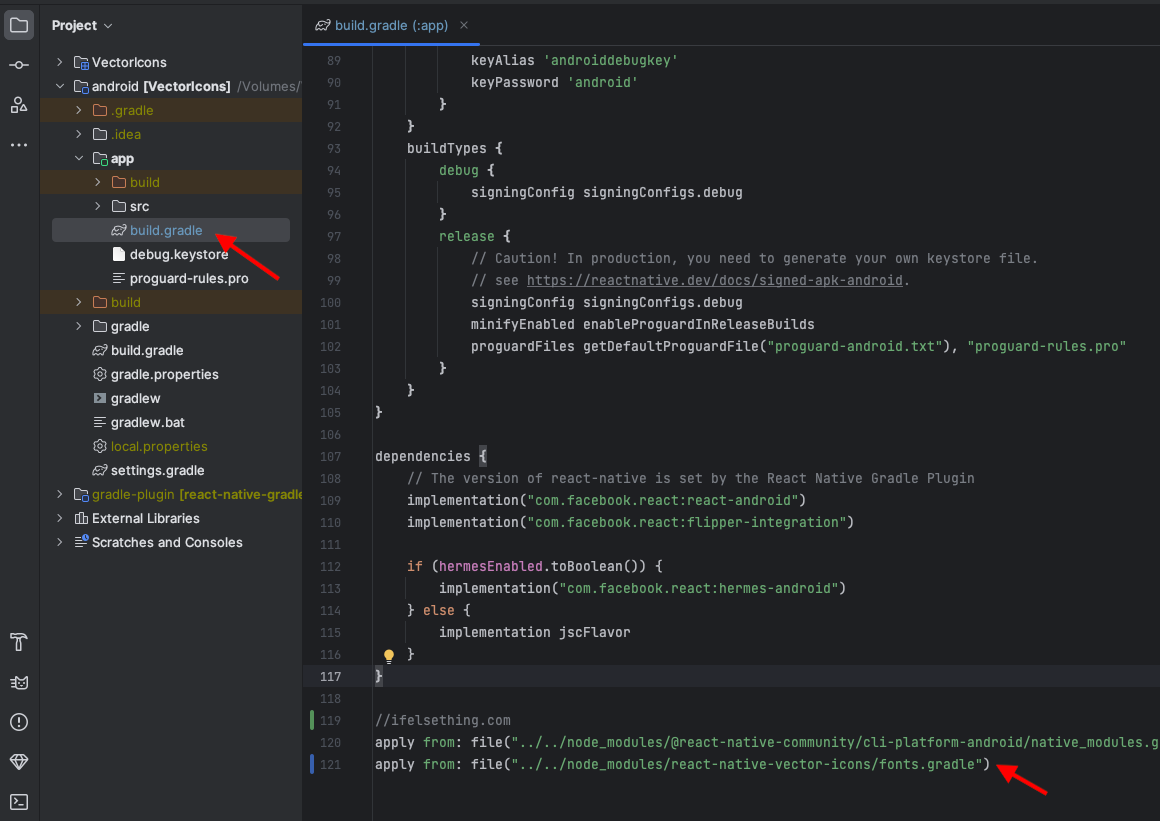
Icons integration
Now that setup is completed, we will go back to our VSCode IDE and open the app.tsx
file. For this post, I will use previous, pause and next icons from AntDesign
.
Import the font which we added to iOS and android during setup. In our case, it's AntDesign
in iOS and every font in android, so AntDesign font will also be present in android device.
//app.tsx
...
import AntDesignIcon from 'react-native-vector-icons/AntDesign';
...
Now use this icon anywhere you need. Name prop is required and other props are optional. We will use stepbackward
, pause
and stepforward
icon names of AntDesign. You can find all icon names HERE;
...
<View style={styles.icon_block}>
<AntDesignIcon name="stepbackward" size={50} color="#900" />
<AntDesignIcon name="pause" size={50} color="#900" />
<AntDesignIcon name="stepforward" size={50} color="#900" />
</View>
...
And the output will be like the images below.
iOS:
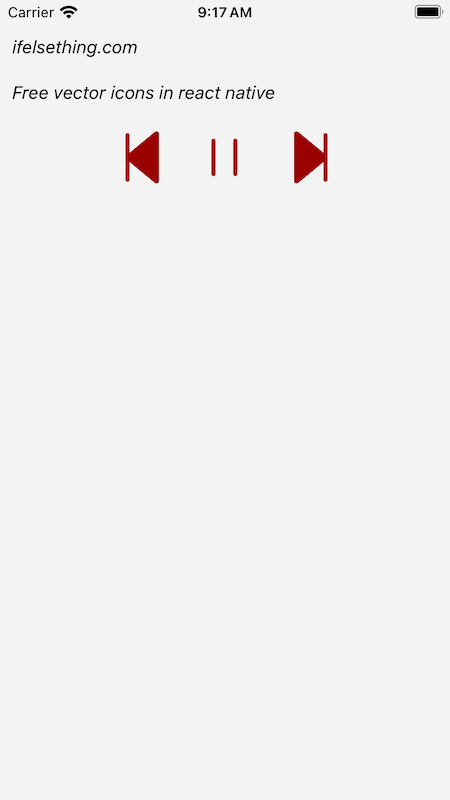
Android:
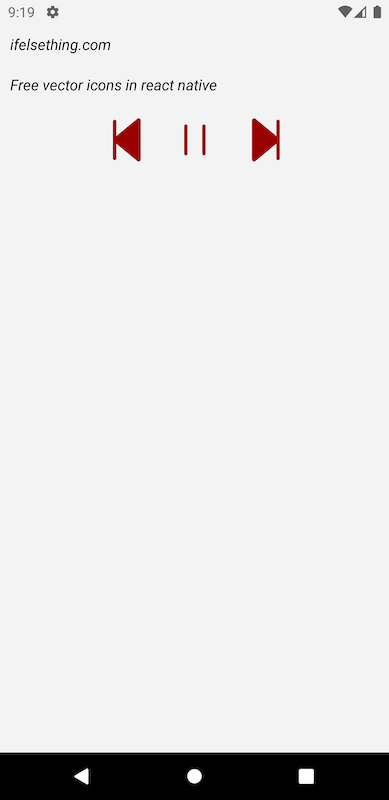
Complete code of this example,
//App.tsx
import React from 'react';
import {
SafeAreaView,
StatusBar,
StyleSheet,
Text,
useColorScheme,
View,
} from 'react-native';
import AntDesignIcon from 'react-native-vector-icons/AntDesign';
import {
Colors
} from 'react-native/Libraries/NewAppScreen';
function App(): React.JSX.Element {
const isDarkMode = useColorScheme() === 'dark';
const backgroundStyle = {
backgroundColor: isDarkMode ? Colors.lighter : Colors.darker, //changed for my use case
flex: 1
};
return (
<SafeAreaView style={backgroundStyle}>
<StatusBar
barStyle={isDarkMode ? 'dark-content' : 'light-content'} //changed for my use case
backgroundColor={backgroundStyle.backgroundColor}
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Free vector icons in react native
</Text>
<View style={styles.icon_block}>
<AntDesignIcon name="stepbackward" size={50} color="#900" />
<AntDesignIcon name="pause" size={50} color="#900" />
<AntDesignIcon name="stepforward" size={50} color="#900" />
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
margin: 10,
gap: 20,
},
input: {
borderColor: 'blue',
borderWidth: 1,
borderRadius: 10,
padding: 10,
fontSize: 15,
color: 'black',
backgroundColor: 'green'
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
icon_block: {
flexDirection: 'row',
gap: 20,
justifyContent: 'center'
}
});
export default App;