Use Svg as a Component in React Native
Published On: 2024-03-08
Posted By: Harish
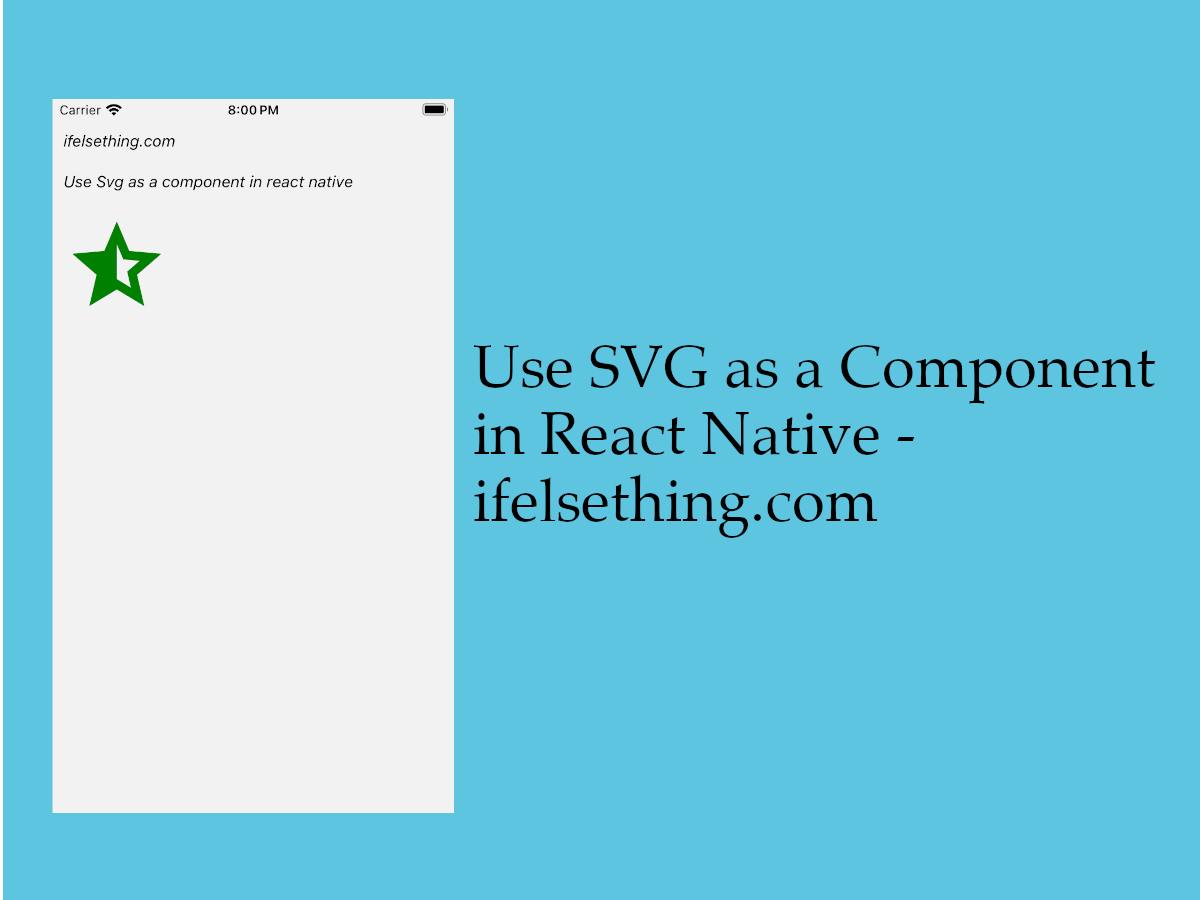
We have seen a way to add icons to react native projects with a library using react-native-vector-icons package. And to add our custom SVG we used another package react-native-vector-image which converts SVG to vector image.
What if you want SVG as a component where we can use props to change size and color of the svg? In that case, we can use another SVG library package, react-native-svg..
Lets see an implementation for this package.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init SvgComponentRN
Example Implementation
We will implement a simple screen with an icon to display.
Install react-native-svg
package using the below command.
npm install react-native-svg
#or
yarn add react-native-svg
And that's it. For iOS, we have to just install pods and build the project. For android, build project directly.
For iOS, open the terminal, cd to the project's ios folder and install pods.
# To use ios folder
cd ..ios
# Install pods
pod install
Add SVG as a Component
Now open the App.tsx
file, import the View
component and return it for now.
Now create a component which returns svg data code. Check below code.
//app.tsx
...
const HalfStarIcon = () => {
return (
<svg
height="100"
viewBox="0 -960 960 960"
width="100"
fill="green"
>
<path
d="m480-363 126 77-33-144 111-96-146-13-58-136v312ZM233-120l65-281L80-590l288-25 112-265 112 265 288 25-218 189 65 281-247-149-247 149Z" />
</svg>
)
};
...
In the above functional component, we are returning data of our example svg to render.
As react native does not support native svg tags, this component will not render. Now import the required tags from the react-native-svg package and replace them with the svg tags.
...
import Svg, { Path } from "react-native-svg";
...
const HalfStarIcon = () => {
return (
<Svg // change from 'svg' to 'Svg'. Similarly change to other tags.
...
>
<Path
...
/>
</Svg>
)
};
...
As you can see, we replaced the tags with the tags from the package. This library supports most of the svg tags. You can create a component directly from SVGR Playground.
Now passing props is also easy.
...
const HalfStarIcon = (props: SvgType) => {
const { width = 50, height = 50, color = "green" } = props;
return (
<Svg
width={width}
heigh={height}
fill={color}
...
>
<Path
...
/>
</Svg>
)
};
...
Finally use this component to render an icon. Check the complete code below.
Run the app to see the final result.
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
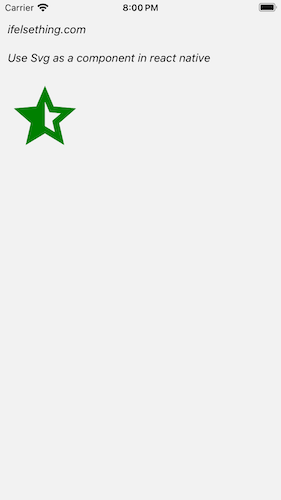
Complete code of our example,
//App.tsx
import {
View,
Text,
StyleSheet
} from "react-native";
import Svg, { Path } from "react-native-svg";
type SvgType = {
width?: number,
height?: number,
color?: string
};
export const App = () => {
const HalfStarIcon = (props: SvgType) => {
const { width = 50, height = 50, color = "green" } = props;
return (
<Svg
height={height}
viewBox="0 -960 960 960"
width={width}
fill={color}
>
<Path
d="m480-363 126 77-33-144 111-96-146-13-58-136v312ZM233-120l65-281L80-590l288-25 112-265 112 265 288 25-218 189 65 281-247-149-247 149Z" />
</Svg>
)
};
return (
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
Use Svg as a component in react native
</Text>
<HalfStarIcon width={100} height={100} />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20,
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
}
});