How to Use Svg in React Native
Published On: 2024-03-08
Posted By: Harish
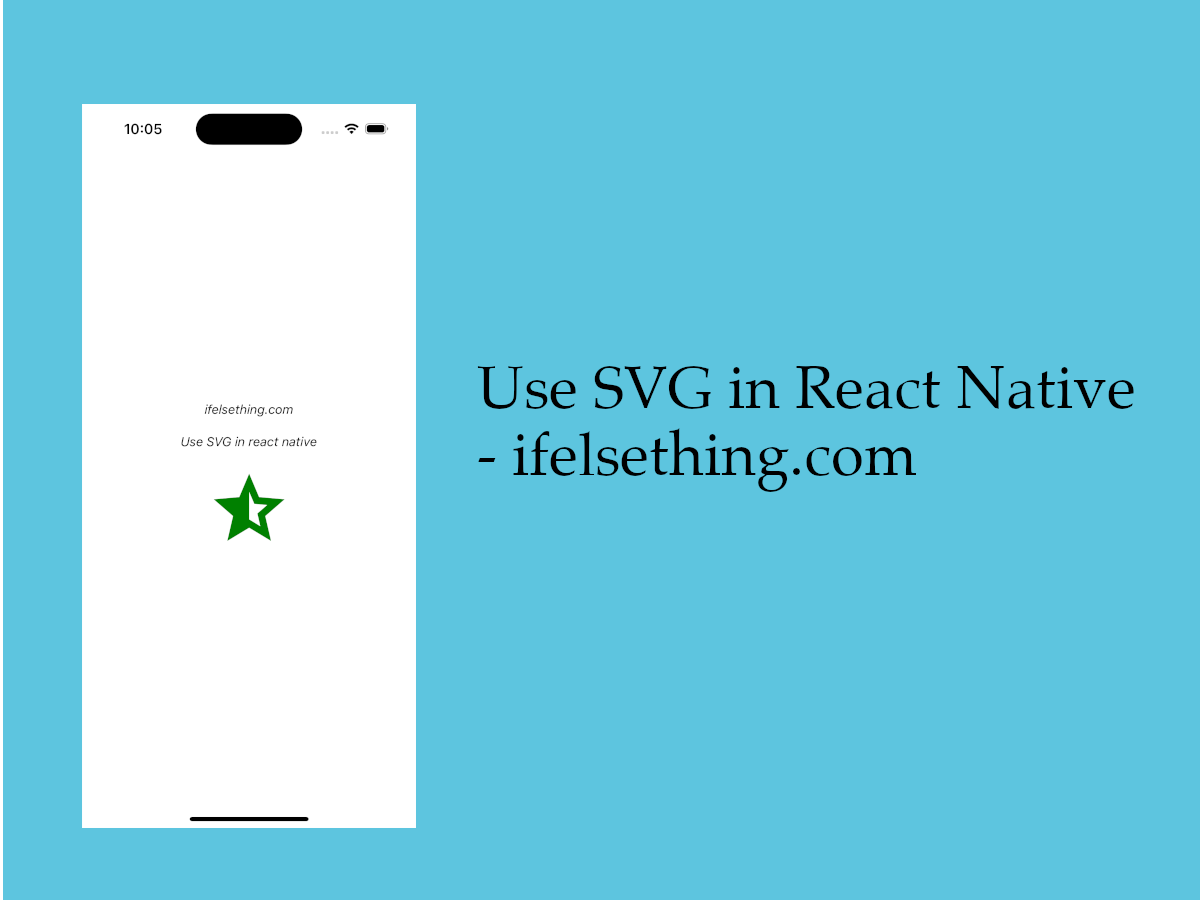
We have seen a way to add icons to react native apps. That library consists of a plethora of vector icons to use in our react native apps. But we cannot use our own icon with this react-native-vector-icons library library.
Using SVG as a component with react-native-svg package is a better way if you want values to change with user interaction or to conditionally apply colors or sizes. I normally use this way.
We can normally import a .png
or .jpg
file and use it in the Image
component without any issue. But to use svg, we need external libraries. Mostly, designers use svg icons to get icon scalability with increase in device resolution. So, in this post we will look into a way to use svg as icons or images in react native.
For this purpose, we are going to use react-native-vector-image library.
Let's see the implementation.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init SvgRN
Example Implementation
We will implement a simple screen with the icon to display.
First, we will install the package. Open the terminal inside the project and install the package using the below command.
npm install react-native-vector-image @klarna/react-native-vector-drawable
#or
yarn add react-native-vector-image @klarna/react-native-vector-drawable
After a successful installation, we have to manually link this package to iOS and android.
iOS
For iOS, open the terminal, cd to the project's ios folder and first install pods.
# To use ios folder
cd ..ios
# Install pods
pod install
After installation, open the ios folder and click on the newly generated .xcworkspace
file. After a successful build, head to the project's target and open Build Phases > Bundle React Native code and images
section.
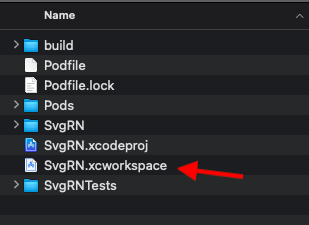
In that section add, NODE_BINARY="../node_modules/react-native-vector-image/strip_svgs.sh"
to shell script and mention newly added NODE_BINARY path to /bin/sh -c
.
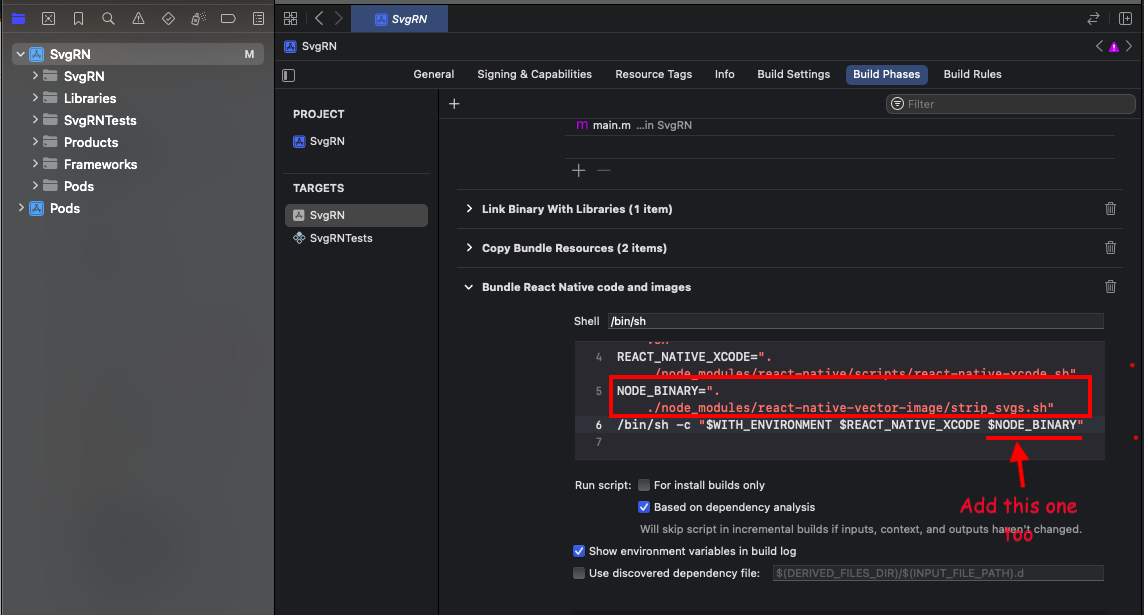
After adding the script, build the project.
Android
For android, open the project's android folder in Android Studio. If you don't have android studio, you can download it from official website.
After successful build, open /app/build.gradle
. We can easily find this file from the android folder by visiting android/app/build.gradle
. Please note that there will be another build.gradle
file outside of the app folder which is not needed now, open the one which is in the app folder.
Paste below line at the bottom of build.gradle
file like in the image below.
apply from: file("../../node_modules/react-native-vector-image/strip_svgs.gradle")
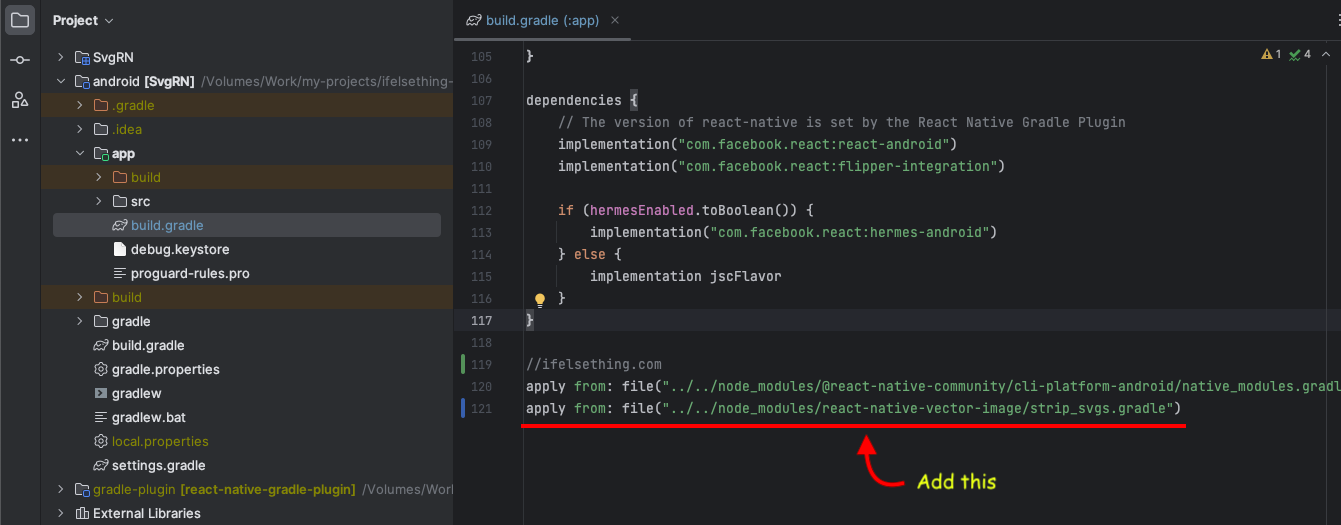
Again build project to complete setup.
Add Svg Icon
Now go to the app.tsx
file, clear not-needed boilerplate code and return only a View
for now.
Import react-native-vector-image library and use the VectorImage
component of the library with an svg icon. I copied one google material svg icon into the assets folder. Assets folder does not exist and that is optional to create. Generally, we place images and fonts in the assets folder.
//app.tsx
...
import VectorImage from 'react-native-vector-image';
const SvgIcon = require('./assets/half-star.svg');
...
return (
...
<VectorImage source={SvgIcon} />
...
)
To generate a vector based svg, we have to run
# This is important
yarn react-native-vector-image generate
We can use an svg only when we run the above command without forgetting for each new svg import.
Have to build the project for every svg to vector image generation i.e. build after running above command. If you don't find the svg image, open XCode for iOS and Android Studio for Android to build projects.
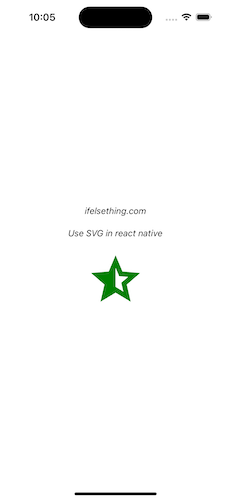
Complete code of our example,
//App.tsx
import React from 'react';
import {
SafeAreaView,
StatusBar,
StyleSheet,
} from 'react-native';
import VectorImage from 'react-native-vector-image';
const SvgIcon = require('./assets/half-star.svg');
function App(): React.JSX.Element {
return (
<SafeAreaView style={styles.container}>
<StatusBar
barStyle={'dark-content'}
/>
<VectorImage source={SvgIcon} />
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}
});
export default App;