Default Value and Value of React Native TextInput
Published On: 2024-04-14
Posted By: Harish
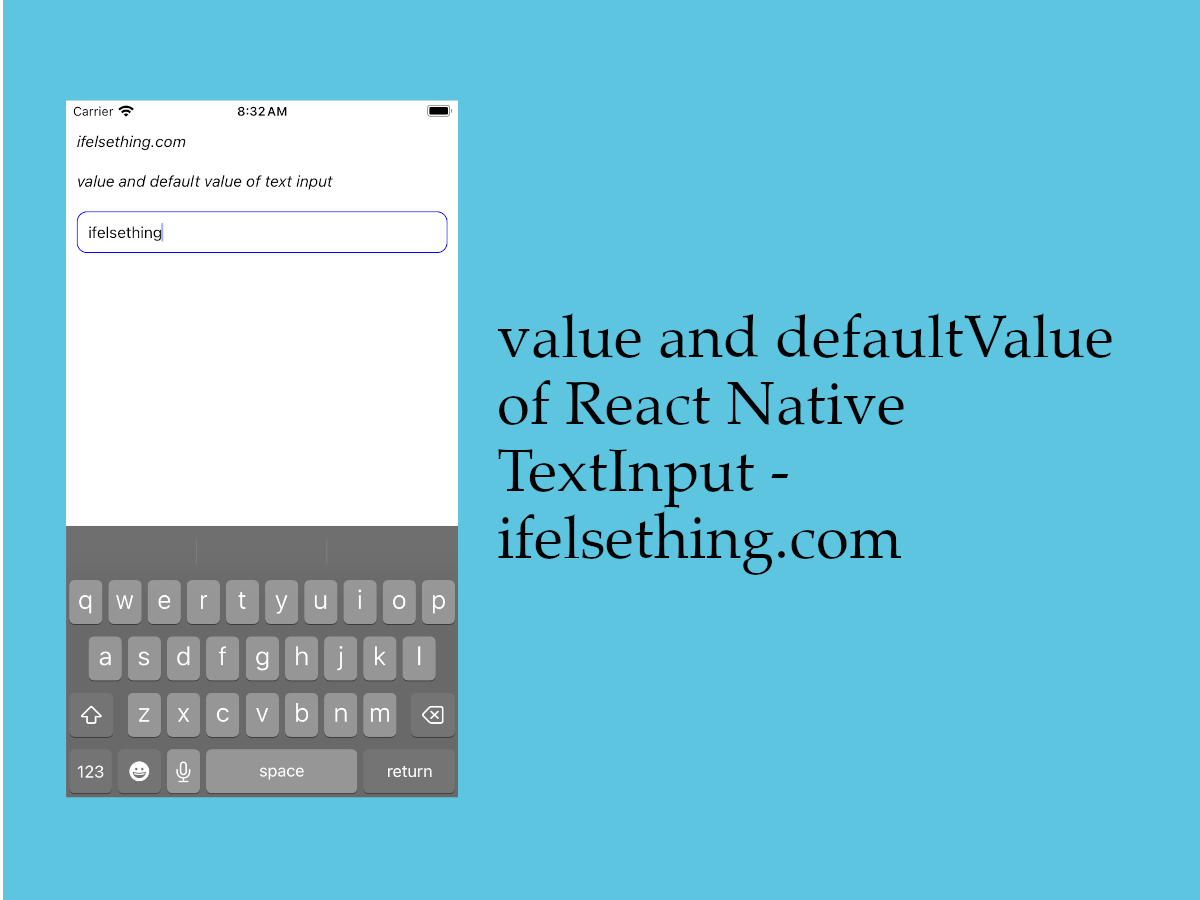
The defaultValue prop is a simple value property which is used to display static text on render and changeable.
On the other side, value prop is the actual value of the text input which will not change when static. We have to use a state variable to set and get the value.
We will do that with a simple text input example.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init TextInputRN
Example Implementation
We will create a simple name text input with default value.
Import the TextInput
component in the App.tsx
file and add basic required props for the name input.
Now add a defaultValue
prop with a static name.
//App.tsx
import { TextInput } from 'react-native';
...
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Name'
placeholderTextColor='gray'
defaultValue="ifelsething"
autoCapitalize="none"
autoCorrect={false}
/>
...
Run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
After the render, you will see the value appears by default. And if you press backspace, this value gets deleted.
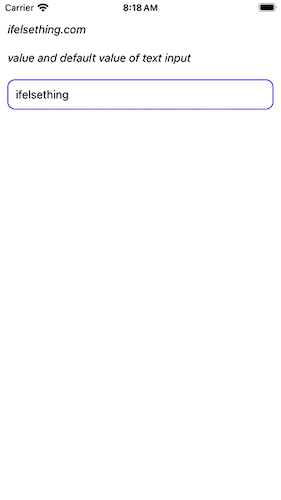
Now do the same with value
prop. Replace defaultValue with value prop.
...
<TextInput
...
value="ifelsething"
/>
...
After the reload, if you try to delete the value, it will not delete and stays there. We can say that the value prop is persistent.
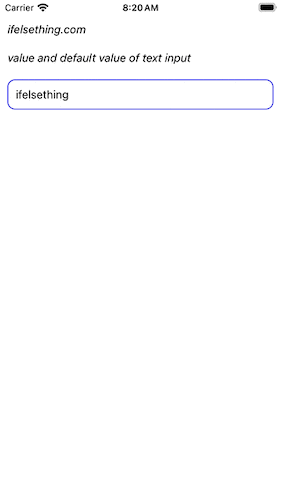
So, to show the updated value, we have to use onChangeText callback which we have seen previously to get the text value while typing.
Here the process is to declare a state variable, get the text from onChangeText callback and assign this state variable to value property.
...
const [text, setText] = useState<string | undefined>();
...
<TextInput
...
value={text}
onChangeText={(t: string) => setText(t)}
/>
...
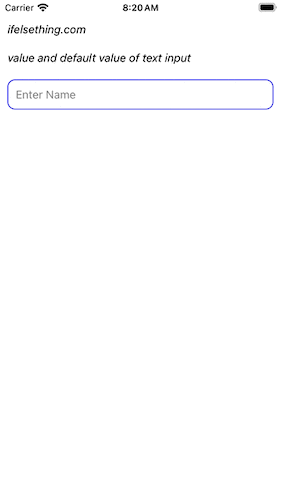
Complete code of our example,
//App.tsx
import React, { useState } from "react";
import {
Text,
StyleSheet,
TextInput,
SafeAreaView,
StatusBar,
View
} from "react-native";
export default function App() {
const [text, setText] = useState<string | undefined>();
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container} >
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
value and default value of text input
</Text>
<TextInput
style={styles.input}
keyboardType='default'
placeholder='Enter Name'
placeholderTextColor='gray'
//defaultValue="ifelsething"
value={text}
onChangeText={(t: string) => setText(t)}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
input: {
borderColor: 'blue',
borderRadius: 10,
borderWidth: 1,
fontSize: 15,
padding: 10,
color: 'black'
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
});