How to Add Multiple Sticky Headers in React Native
Published On: 2023-12-30
Posted By: Harish
We have seen a way to add a sticky header in scrollview. But what if we want multiple sticky headers? ScrollView sticky header can be used for content with different structured views. If we have the same structured data for multiple headers, we can use React Native's SectionsList component.
For this example, we can think of a contact app with an alphabet as a sticky header followed by its contact's list.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ReactNativeStickyHeaderSectionListExample
Create a data array
SectionsList takes an array list of objects containing title and data as keys. Whatever the data which you want to show, has to be in this format. Below we are creating a dummy contacts list with a title.
//app.tsx
const data = [
{
title: 'A',
data: [
'contact 1',
'contact 2',
'contact ifelsething',
'contact 4',
'contact 5',
'contact 6',
'contact 7',
'contact 8',
'contact 9',
'contact 10',
]
},
{
title: 'B',
data: [
'contact 1',
'contact 2',
'contact 3',
'contact 4',
'contact 5',
'contact 6',
'contact 7',
'contact 8',
'contact 9',
'contact 10',
]
},
...
...
];
You can pass objects, strings, arrays etc inside data
key array. For this post, it's a string.
//app.tsx
const data //Object example array = [
{
title: 'A',
data: [
{
name: 'contact 1',
phone: '123456'
},
{
name: 'contact 2',
phone: '123446'
},
...
...
]
},
{
title: 'B',
data: [
{
name: 'contact 1',
phone: '123456'
},
{
name: 'contact 2',
phone: '123446'
},
...
...
]
},
...
...
];
Now pass the data to SectionList's section prop. renderItem prop in SectionList is to render content component and renderSectionHeader prop is for sticky header component.
//app.tsx
const renderItem = (item: any) => {
return <View style={styles.item}>
<Text style={styles.text}>{item}</Text>
</View>
};
return (
<SectionList
sections={data}
keyExtractor={(item, index) => item + index}
renderItem={({item}) => renderItem(item)}
renderSectionHeader={({section: {title}}) => (
<Text style={styles.header}>{title}</Text>
)}
/>
);
if you run the project
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
you will get the output like this,
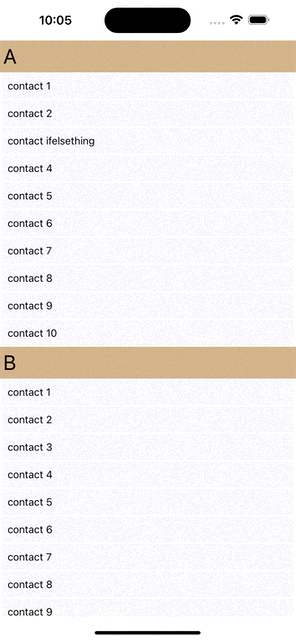
Complete code,
//app.tsx
import {
Text,
View,
StyleSheet,
SectionList
} from "react-native";
const data = [
{
title: 'A',
data: [
'contact 1',
'contact 2',
'contact ifelsething',
'contact 4',
'contact 5',
'contact 6',
'contact 7',
'contact 8',
'contact 9',
'contact 10',
]
},
...
...
];
export const App = () => {
const renderItem = (item: any) => {
return <View style={styles.item}>
<Text style={styles.text}>{item}</Text>
</View>
};
return (
<SectionList
sections={data}
keyExtractor={(item, index) => item + index}
renderItem={({item}) => renderItem(item)}
renderSectionHeader={({section: {title}}) => (
<Text style={styles.header}>{title}</Text>
)}
/>
);
}
const styles = StyleSheet.create({
scrollView: {
flex: 1,
backgroundColor: 'white'
},
header: {
padding: 5,
fontSize: 30,
backgroundColor: 'tan'
},
item: {
fontSize: 18,
padding: 10,
borderWidth: 1,
borderColor: 'white',
justifyContent: 'flex-start',
backgroundColor: 'ghostwhite'
},
text: {
color: 'black',
fontSize: 15,
},
});